How to generate random numbers in Ruby?
Generating random numbers in Ruby is a common task, and it can be useful in various applications such as simulations, games, and data analysis. Ruby provides several methods and libraries for generating random numbers. Here are some of the most commonly used techniques:
- `rand` Method:
Ruby’s built-in `rand` method generates random floating-point numbers between 0.0 (inclusive) and 1.0 (exclusive) by default. You can also provide an argument to specify a range of random numbers.
```ruby random_number = rand # Generates a random number between 0.0 and 1.0 random_integer = rand(1..100) # Generates a random integer between 1 and 100 ```
- `Random` Class:
Ruby includes the `Random` class, which allows you to create a random number generator object. You can use this object to generate random numbers with more control and repeatability.
```ruby rng = Random.new random_number = rng.rand(1..10) ```
- `SecureRandom` Module:
If you need secure random numbers for cryptography or security-related applications, Ruby provides the `SecureRandom` module. It generates cryptographically secure random numbers and strings.
```ruby require 'securerandom' random_hex = SecureRandom.hex(16) # Generates a random 32-character hex string ```
- External Libraries:
Ruby also offers external libraries like `Faker` for generating random data that simulates real-world information such as names, addresses, and more. These libraries are particularly useful in testing and development.
```ruby require 'faker' random_name = Faker::Name.name ```
When generating random numbers, it’s essential to consider the specific requirements of your application. Depending on the use case, you can choose the appropriate method or library to ensure that the random numbers are suitable for your needs. Whether you need basic random numbers or secure, cryptographically strong ones, Ruby provides the tools to meet your requirements.
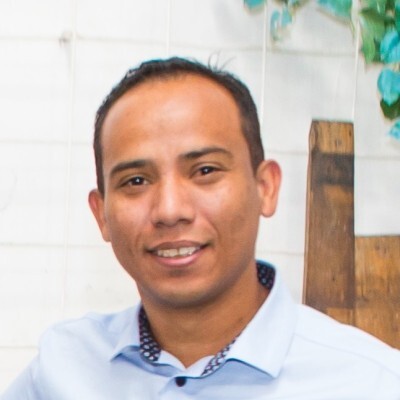
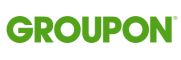