What is the difference between ‘gets’ and ‘chomp’ in Ruby?
In Ruby, ‘gets’ and ‘chomp’ are two commonly used methods when dealing with user input, particularly when reading text from the standard input (keyboard). They serve different purposes and are often used together to process user input effectively.
- `gets`:
– `gets` is a method used to read a line of text from the standard input (usually the keyboard). It allows the program to wait for the user to input a line of text.
– When `gets` is called, it captures the user’s input, including any newline character (`\n`) at the end of the input, which represents the Enter key press.
– The input captured by `gets` is stored as a string, and you can assign it to a variable for further processing.
```ruby user_input = gets ```
- `chomp`:
– `chomp` is a method used to remove the trailing newline character (`\n`) from a string. It is often used in conjunction with `gets` to eliminate the newline character from user input.
– Removing the newline character is essential because it can affect the formatting of the output or cause unexpected behavior when working with strings. For example, when displaying the input, you generally don’t want an extra line break.
```ruby user_input = gets.chomp ```
This code captures user input using `gets` and immediately applies `chomp` to remove the trailing newline character, ensuring that the resulting `user_input` variable contains only the text entered by the user.
`gets` is used to capture user input as a string, including the newline character, while `chomp` is used to remove the trailing newline character from that string. Using both methods together is a common practice when working with user input in Ruby to ensure that the input is in a clean and usable format.
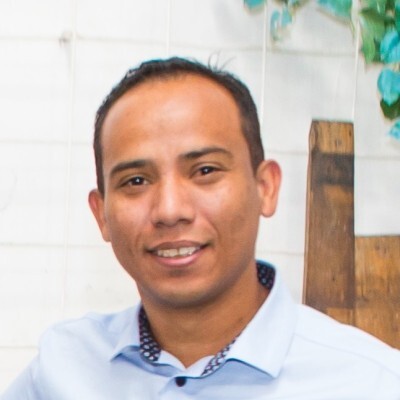
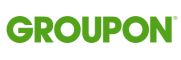