How to handle exceptions in Ruby?
Handling exceptions in Ruby is essential for gracefully managing unexpected errors or exceptional conditions that may occur during program execution. Ruby provides a robust mechanism for exception handling that allows you to capture and handle errors effectively. Here’s how you can handle exceptions in Ruby:
- Begin-Rescue-End Blocks: To handle exceptions, you use `begin`, `rescue`, and `end` blocks. The `begin` block contains the code that might raise an exception, the `rescue` block specifies the code to execute when an exception occurs, and the `end` block marks the end of the exception-handling code.
```ruby begin # Code that might raise an exception result = 10 / 0 rescue ZeroDivisionError # Code to handle the exception puts "Error: Division by zero" end ```
In this example, the `ZeroDivisionError` is raised due to dividing by zero, and the `rescue` block handles the exception by displaying an error message.
- Handling Multiple Exceptions: You can rescue multiple exceptions in a single `rescue` block by listing them with `=>`.
```ruby begin # Code that might raise an exception result = 10 / 0 rescue ZeroDivisionError, TypeError # Code to handle the exceptions puts "Error: Division by zero or type error" end ```
- Rescue Without Specifying Exception: You can use a `rescue` block without specifying a particular exception to catch all exceptions. However, it’s generally recommended to rescue specific exceptions whenever possible to avoid masking unexpected issues.
```ruby begin # Code that might raise an exception result = 10 / 0 rescue # Code to handle any exception puts "An error occurred" end ```
- Ensure Block: You can include an `ensure` block after the `rescue` block to specify code that will always execute, whether an exception is raised or not. This is useful for cleanup tasks.
```ruby begin # Code that might raise an exception file = File.open("file.txt", "r") # Process the file rescue IOError # Code to handle file-related exceptions puts "Error: File not found or unable to open" ensure file.close if file end ```
Exception handling in Ruby provides a structured way to handle errors, making your code more robust and preventing it from crashing when unexpected issues arise. It’s essential to choose the appropriate exception to rescue and provide meaningful error messages to aid in debugging and troubleshooting.
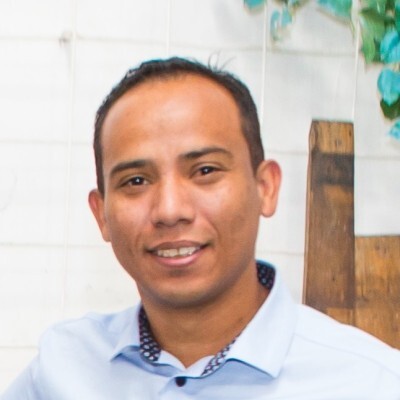
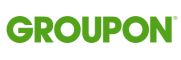