How to handle user input in Ruby?
Handling user input in Ruby involves interacting with users to gather data or make decisions within a program. This process can be achieved using various methods and techniques, depending on the nature of the input and the complexity of the application. Here are some common approaches to handle user input in Ruby:
- Standard Input (STDIN):
Ruby provides a built-in constant called `STDIN` that represents standard input from the command line. You can use `gets` to read input from the user. For example:
```ruby puts "Enter your name: " user_input = gets.chomp puts "Hello, #{user_input}!" ```
Here, `gets.chomp` is used to read a line of text from the user, and `chomp` removes the newline character from the end of the input.
- Command-Line Arguments:
As discussed earlier, you can access command-line arguments using the `ARGV` array to provide initial input when running a Ruby script. This is suitable for passing configuration options or file paths.
- Interactive Prompts:
Ruby libraries like `HighLine` or `TTY::Prompt` allow you to create interactive command-line prompts, enabling you to ask questions and receive input from the user in a structured manner.
```ruby require 'highline' cli = HighLine.new user_input = cli.ask("What's your favorite color? ") puts "You chose #{user_input}." ```
- Error Handling:
When handling user input, it’s essential to include error handling to account for unexpected or invalid input. Techniques like checking for input validation, using regular expressions, or implementing retry mechanisms can help ensure that user input is valid and that the program responds appropriately to errors.
- User Interfaces (UI):
For more complex applications, you may consider building graphical user interfaces (GUIs) using Ruby libraries like Shoes or GUI frameworks like Ruby on Rails. These provide interactive and visually appealing ways to collect and process user input.
Handling user input effectively is crucial for creating user-friendly and functional Ruby applications. Depending on the context and requirements of your program, you can choose the most suitable method or library to gather and process user input while ensuring a smooth and error-resistant user experience.
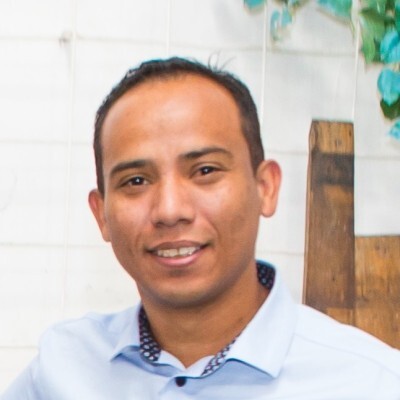
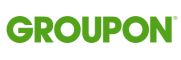