Scaling Ruby Applications: Strategies for Handling High Traffic
In today’s digital landscape, web applications often face the challenge of handling high traffic loads. This is both an exciting and daunting task for Ruby developers. Ruby, known for its elegant syntax and developer-friendly nature, can encounter performance bottlenecks when dealing with a surge in traffic. However, with the right strategies and best practices, you can ensure your Ruby applications scale gracefully and maintain optimal performance even under heavy loads. In this article, we will explore various approaches to scaling Ruby applications effectively.
Table of Contents
1. Caching for Performance Optimization:
Caching is a fundamental technique to enhance the performance of Ruby applications, especially when dealing with high traffic. By storing frequently accessed data in memory, you can reduce the need for repeated database queries and expensive calculations.
1.1 Page Caching:
Page caching involves saving the entire HTML output of a page to a cache and serving it directly to users. This can be highly effective for static or semi-static pages that don’t change frequently.
ruby class PagesController < ApplicationController def home # ... # Your regular controller logic here # ... expires_in 1.hour, public: true end end
1.2 Fragment Caching:
For parts of your page that change less often, like individual components, fragment caching can be used. This caches specific sections of your views and helps reduce the load on the server.
ruby <% cache @recent_posts do %> <!-- Render recent posts here --> <% end %>
2. Load Balancing and Horizontal Scaling:
As traffic increases, a single server might not be enough to handle the load. Load balancing distributes incoming requests across multiple servers, ensuring a smoother user experience and improved response times.
2.1 Using a Load Balancer:
Deploy a load balancer that distributes incoming requests among several backend servers. Popular options include Nginx, HAProxy, and Amazon ELB.
nginx http { upstream backend { server app_server1; server app_server2; server app_server3; } server { listen 80; location / { proxy_pass http://backend; } } }
3. Database Optimization:
Database performance is crucial for scaling Ruby applications. Optimize your database queries, use appropriate indexing, and consider database sharding or replication to distribute the load.
3.1 Query Optimization:
Write efficient database queries that fetch only the required data. Utilize tools like the Bullet gem to identify and eliminate N+1 query issues.
ruby # In Gemfile gem 'bullet' # In config/environments/development.rb config.after_initialize do Bullet.enable = true Bullet.rails_logger = true end
3.2 Database Indexing:
Index columns that are frequently used in WHERE clauses. This accelerates data retrieval and query performance.
ruby class CreatePosts < ActiveRecord::Migration[6.0] def change create_table :posts do |t| t.string :title t.text :content t.timestamps end add_index :posts, :title end end
4. Asynchronous Processing:
Heavy traffic can lead to delays in processing tasks. By leveraging asynchronous processing, you can offload time-consuming tasks to background jobs, ensuring the responsiveness of your application.
4.1 Using Delayed Job:
Delayed Job is a popular gem that provides a simple way to handle background tasks in Ruby applications.
ruby # In Gemfile gem 'delayed_job_active_record' # In a controller or service class UsersController < ApplicationController def create # ... UserMailer.delay.send_welcome_email(user) # ... end end
5. Content Delivery Networks (CDNs):
CDNs distribute your assets (images, CSS, JavaScript) to various server locations, reducing the load on your primary server and improving load times for users across the globe.
5.1 Integrating with a CDN:
Integrate your application with a CDN like Cloudflare or Amazon CloudFront to serve static assets efficiently.
html <!-- In your layout file --> <%= stylesheet_link_tag "application", media: "all" %> <%= javascript_include_tag "application" %> <%= image_tag "logo.png" %>
6. Horizontal vs. Vertical Scaling:
When scaling your Ruby application, you can choose between horizontal scaling (adding more servers) and vertical scaling (upgrading server resources). Each approach has its pros and cons, and the right choice depends on your application’s specific needs.
6.1 Horizontal Scaling:
Add more servers to distribute the load, allowing your application to handle increased traffic. This approach is suitable for applications that have a variable load pattern.
6.2 Vertical Scaling:
Upgrade your existing server’s resources, such as CPU, memory, or storage, to handle higher loads. This approach is ideal when you anticipate gradual traffic growth.
Conclusion
Scaling Ruby applications to handle high traffic is a challenging but rewarding endeavor. By implementing caching strategies, load balancing, database optimizations, asynchronous processing, and leveraging CDNs, you can ensure your applications maintain exceptional performance even during traffic spikes. Remember that each application is unique, so consider your specific requirements when selecting the right scaling strategies. Armed with these techniques, you can confidently tackle high traffic and provide a seamless experience to your users, ensuring your Ruby applications are ready for the demands of the digital world.
Table of Contents
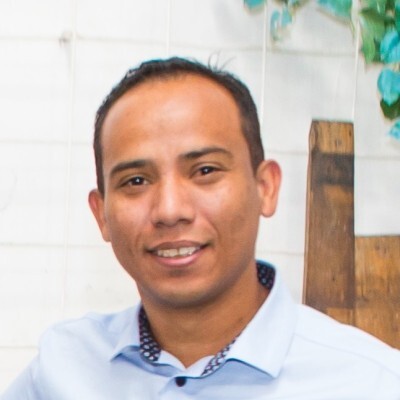
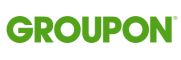