How to make HTTP requests in Ruby?
In Ruby, you can make HTTP requests to interact with web services, APIs, or fetch data from remote servers using the `Net::HTTP` library. Here’s a step-by-step guide on how to make HTTP requests in Ruby:
- Require the `net/http` Library:
Begin by requiring the `net/http` library, which provides classes and methods for making HTTP requests:
```ruby require 'net/http' ```
- Create a URI Object:
Define the URI (Uniform Resource Identifier) of the resource you want to access. You can create a `URI` object by parsing a URL string:
```ruby require 'uri' url = URI.parse('https://example.com/api/resource') ```
- Create an HTTP Request:
You can create an HTTP request by creating an instance of the `Net::HTTP` class and specifying the request method (GET, POST, PUT, DELETE, etc.):
```ruby http = Net::HTTP.new(url.host, url.port) request = Net::HTTP::Get.new(url) ```
You can replace `Net::HTTP::Get` with other methods like `Net::HTTP::Post` or `Net::HTTP::Put` to send different types of requests.
- Add Headers (Optional):
If your request requires headers (e.g., authentication tokens or custom headers), you can set them using the `add_field` method:
```ruby request.add_field('Authorization', 'Bearer your_token_here') ```
- Send the Request and Receive the Response:
Use the `request` you’ve created to send the HTTP request and receive the response:
```ruby response = http.request(request) ```
The `response` object contains information about the HTTP response, such as the status code, headers, and response body.
- Handle the Response:
You can access the response data as follows:
```ruby status_code = response.code headers = response.each_header.to_h body = response.body ```
You can then parse and work with the `body` based on the expected data format, typically JSON or XML.
- Error Handling:
Be sure to implement error handling to deal with potential network issues or unexpected responses, using standard Ruby exception handling.
Here’s an example of making a GET request to a hypothetical API:
```ruby require 'net/http' require 'uri' url = URI.parse('https://api.example.com/data') http = Net::HTTP.new(url.host, url.port) request = Net::HTTP::Get.new(url) response = http.request(request) if response.code == '200' puts "Response: #{response.body}" else puts "HTTP Error: #{response.code}" end ```
This is a basic overview of how to make HTTP requests in Ruby. You can customize the request headers, handle different HTTP methods, and handle responses based on your specific use case or API requirements. Additionally, you might consider using popular HTTP client libraries like `HTTParty` or `Faraday` for more advanced features and easier request handling.
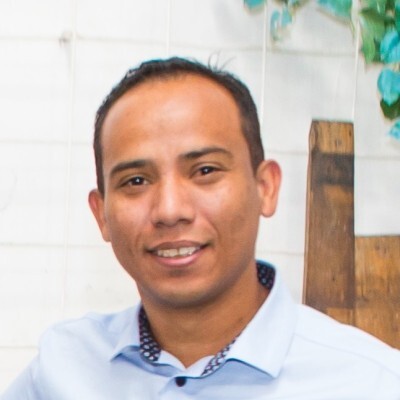
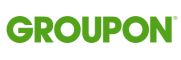