How to use the ‘if’ statement in Ruby?
In Ruby, the `if` statement is a fundamental control structure used to conditionally execute code based on a specified condition. It allows you to control the flow of your program by running certain code only when a given condition is met. Here’s how to use the `if` statement in Ruby:
- Basic `if` Statement:
The most common form of the `if` statement consists of the keyword `if`, followed by a condition, and then a block of code. If the condition evaluates to `true`, the code inside the block is executed; otherwise, it’s skipped.
```ruby age = 25 if age >= 18 puts "You are an adult." end ```
In this example, the code inside the `if` block will run because `age` is greater than or equal to 18.
- `if-else` Statement:
You can extend the `if` statement with an `else` clause to specify code that should run when the condition is not met (i.e., when it evaluates to `false`).
```ruby temperature = 25 if temperature > 30 puts "It's hot outside." else puts "It's not too hot." end ```
Here, if the `temperature` is greater than 30, the first message will be printed; otherwise, the message in the `else` block will be executed.
- `if-elsif-else` Statement:
For handling multiple conditions, you can use the `elsif` clause after the initial `if` statement. It allows you to check additional conditions sequentially.
```ruby score = 85 if score >= 90 puts "A" elsif score >= 80 puts "B" else puts "C" end ```
In this example, the code will print “B” because the `score` falls into the second condition range.
- Ternary Operator:
Ruby also offers a concise way to use the `if` statement for simple conditional assignments or expressions using the ternary operator (`? :`).
```ruby age = 20 result = (age >= 18) ? "Adult" : "Minor" ```
Here, `result` will be assigned “Adult” if the condition is true and “Minor” if it’s false.
The `if` statement is a powerful tool for controlling the flow of your Ruby programs based on conditions. It allows you to create logic that responds dynamically to changing data and user input, making your code more adaptable and versatile.
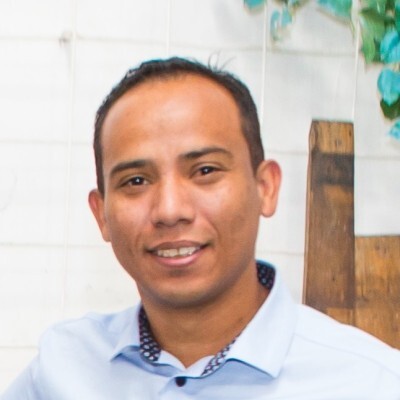
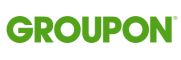