What is inheritance in Ruby?
Inheritance in Ruby is a fundamental concept in object-oriented programming (OOP) that allows you to create new classes based on existing classes, inheriting their attributes and behaviors. It promotes code reuse, abstraction, and hierarchy within your application’s class structure. In Ruby, a class that inherits from another class is called a subclass or derived class, while the class being inherited from is referred to as the superclass or base class.
Key points about inheritance in Ruby:
- Creating Subclasses: To create a subclass, you use the `<` symbol followed by the name of the superclass when defining the class. This establishes an “is-a” relationship, indicating that the subclass is a specialized version of the superclass.
```ruby class Animal def speak puts "Some sound" end end class Dog < Animal def speak puts "Woof!" end end ```
In this example, `Dog` is a subclass of `Animal`, and it inherits the `speak` method from its superclass.
- Inheriting Methods and Attributes: Subclasses inherit all the methods and attributes (instance variables) of their superclass. This means that you can reuse and extend the functionality of the superclass in the subclass without rewriting the same code.
- Overriding Methods: Subclasses have the option to override methods inherited from the superclass. This allows you to provide a specific implementation for a method in the subclass while maintaining the same method name as the superclass.
```ruby class Cat < Animal def speak puts "Meow!" end end ```
Here, the `Cat` class overrides the `speak` method to provide its own behavior.
- Super Keyword: In overridden methods, you can use the `super` keyword to call the corresponding method in the superclass. This enables you to leverage the functionality of the superclass while adding specific behavior in the subclass.
```ruby class Dog < Animal def speak super puts "Wagging tail" end end ```
In this example, `super` calls the `speak` method of the `Animal` superclass before adding the “Wagging tail” behavior.
Inheritance in Ruby is a powerful mechanism for creating hierarchical relationships among classes and organizing code in a structured and reusable manner. It helps in building a more modular and maintainable codebase by promoting the sharing and extension of functionality across related classes.
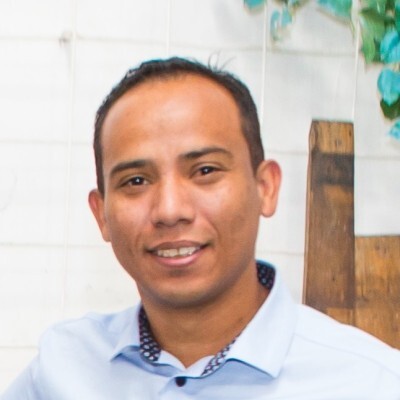
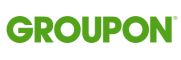