What are instance variables in Ruby?
In Ruby, instance variables are a fundamental concept in object-oriented programming, used to store and manage data that belongs to an instance of a class. Each object (instance) created from a class can have its own set of instance variables, allowing it to maintain and encapsulate its state. Here’s what you need to know about instance variables:
- Naming Convention: Instance variables in Ruby are prefixed with the `@` symbol followed by a variable name. For example, `@name` and `@age` are instance variables commonly used to represent properties of objects.
- Instance-Specific: Unlike local variables, which have a limited scope within a method, instance variables are accessible throughout the entire object. This means that multiple methods within the same object can access and modify the same instance variables.
- Initialization: Instance variables are often initialized in the class’s constructor method, typically named `initialize`. This method is called when an object is created, and it sets the initial values of instance variables.
```ruby class Person def initialize(name, age) @name = name @age = age end end ```
In this example, `@name` and `@age` are instance variables that store the name and age of a `Person` object.
- Access and Modification: You can access and modify instance variables using getter and setter methods. Getter methods retrieve the value of an instance variable, while setter methods allow you to change its value.
```ruby class Person def initialize(name, age) @name = name @age = age end def name @name end def age=(new_age) @age = new_age end end ```
In this example, the `name` method acts as a getter for `@name`, and the `age=` method acts as a setter for `@age`.
Instance variables are a crucial component of object-oriented programming in Ruby, as they enable objects to maintain and encapsulate their state, making it possible to represent and work with real-world entities in a structured and organized manner.
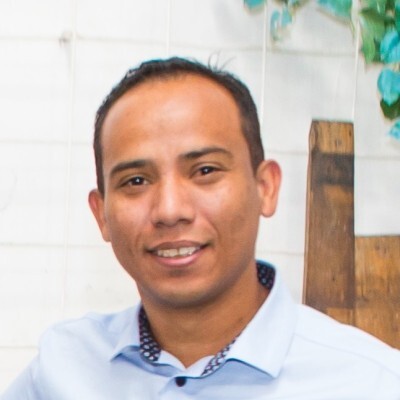
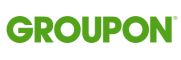