Creating Interactive Data Dashboards with Ruby: Visualizing Insights
In today’s data-driven world, making sense of complex datasets is crucial for informed decision-making. Interactive data dashboards provide a powerful solution for visualizing insights, enabling businesses to gain a deeper understanding of their data. While there are various tools and programming languages for building interactive dashboards, Ruby offers a flexible and elegant option that’s worth exploring. In this blog post, we will walk you through the process of creating interactive data dashboards with Ruby, including step-by-step guidance, code samples, and best practices.
Table of Contents
1. Why Choose Ruby for Data Dashboards?
Before diving into the technical details, let’s briefly explore why Ruby is a great choice for building data dashboards:
1.1. Ruby’s Expressive Syntax
Ruby is known for its clean and expressive syntax, which makes it easy to write and read code. This readability is crucial when developing data dashboards, as it simplifies the creation and maintenance of complex visualizations.
1.2. Rich Ecosystem
Ruby has a rich ecosystem of libraries and frameworks that can be leveraged to streamline dashboard development. One such library is Sinatra, a lightweight web framework that is well-suited for building small to medium-sized dashboards.
1.3. Flexibility and Customization
Ruby offers a high degree of flexibility, allowing you to tailor your data dashboard to your specific needs. You can integrate various data sources, libraries, and plugins to create a customized dashboard that meets your requirements.
1.4. Active Community
The Ruby community is active and supportive, which means you can find plenty of resources, documentation, and help when you encounter challenges during your dashboard development journey.
2. Setting Up Your Ruby Environment
Before we start building our interactive data dashboard, let’s ensure you have the necessary tools and environment set up. We will use Sinatra as our web framework and various Ruby gems for data manipulation and visualization.
2.1. Installing Ruby
If you don’t have Ruby installed on your system, you can download and install it from the official Ruby website: Ruby Official Website.
2.2. Setting Up Sinatra
Sinatra is a lightweight web framework that makes it easy to create web applications and APIs. You can install Sinatra using the following command:
ruby gem install sinatra
2.3. Other Dependencies
We’ll also need additional Ruby gems for data manipulation and visualization. You can add them to your project’s Gemfile and install them using Bundler:
ruby # Gemfile source 'https://rubygems.org' gem 'sinatra' gem 'pandas' gem 'plotly'
Install the gems with Bundler:
bash bundle install
3. Building a Simple Data Dashboard
Now that we have our environment set up, let’s create a simple data dashboard that visualizes some sample data. In this example, we’ll use Pandas for data manipulation and Plotly for creating interactive visualizations.
3.1. Data Preparation
To keep things straightforward, we’ll work with a CSV file containing sample data. You can use your own dataset by modifying the code accordingly. First, create a CSV file named sample_data.csv with the following content:
csv Date,Revenue,Profit 2023-01-01,1000,300 2023-02-01,1200,400 2023-03-01,1400,500 2023-04-01,1600,600 2023-05-01,1800,700 2023-06-01,2000,800
Now, let’s write a Ruby script that loads this data, processes it using Pandas, and prepares it for visualization:
ruby require 'pandas' # Load the CSV data data = Pandas.read_csv('sample_data.csv') # Create a Plotly figure figure = { data: [ { x: data['Date'], y: data['Revenue'], type: 'scatter', mode: 'lines+markers', name: 'Revenue' }, { x: data['Date'], y: data['Profit'], type: 'scatter', mode: 'lines+markers', name: 'Profit' } ], layout: { title: 'Revenue and Profit Over Time', xaxis: { title: 'Date' }, yaxis: { title: 'Amount' } } } # Display the Plotly figure Pandas.plotly.offline.plot(figure)
This script uses Pandas to load the data from the CSV file, creates a Plotly figure with two line charts (one for revenue and one for profit), and displays the interactive visualization in your default web browser.
3.2. Running the Dashboard
Save the script as dashboard.rb and run it using the following command:
bash ruby dashboard.rb
This will start a local web server, and you can access the dashboard in your web browser at http://localhost:4567. You should see an interactive chart displaying revenue and profit over time.
4. Adding Interactivity
While our simple dashboard provides a basic visualization, let’s enhance it by adding interactivity. Users often want to interact with data by selecting specific date ranges or applying filters. We can achieve this with a few modifications to our code.
4.1. Adding Date Range Picker
To allow users to select a date range, we can add a date range picker using the datepicker JavaScript library. First, include the necessary JavaScript and CSS files in your HTML template. Create a file named views/index.erb with the following content:
html <!DOCTYPE html> <html> <head> <title>Data Dashboard</title> <link rel="stylesheet" type="text/css" href="https://cdnjs.cloudflare.com/ajax/libs/datepicker/1.0.10/datepicker.min.css"> <script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/datepicker/1.0.10/datepicker.min.js"></script> </head> <body> <h1>Data Dashboard</h1> <div id="date-picker"></div> <div id="chart"></div> <script> document.addEventListener('DOMContentLoaded', function() { const datepicker = new Datepicker(document.getElementById('date-picker'), { autohide: true, format: 'yyyy-mm-dd', range: true }); datepicker.show(); datepicker.addEventListener('changeDate', function(e) { const startDate = e.dates[0]; const endDate = e.dates[1]; // Fetch and update data for the selected date range fetch(`/data?start_date=${startDate}&end_date=${endDate}`) .then(response => response.json()) .then(data => { // Update the chart with the new data Plotly.react('chart', data); }); }); }); </script> </body> </html>
This HTML template includes a date range picker and a container for our chart. We’ve also added JavaScript code to fetch data based on the selected date range and update the chart using Plotly.
4.2. Modifying the Ruby Script
Next, we need to modify our Ruby script to handle data requests and respond with JSON data based on the selected date range. Update your dashboard.rb script as follows:
ruby require 'sinatra' require 'pandas' require 'json' # Load the CSV data data = Pandas.read_csv('sample_data.csv') # Define a route for serving the dashboard get '/' do erb :index end # Define a route for fetching data get '/data' do start_date = Date.parse(params[:start_date]) end_date = Date.parse(params[:end_date]) # Filter the data based on the selected date range filtered_data = data[(data['Date'] >= start_date) & (data['Date'] <= end_date)] # Prepare data for the chart chart_data = [ { x: filtered_data['Date'], y: filtered_data['Revenue'], type: 'scatter', mode: 'lines+markers', name: 'Revenue' }, { x: filtered_data['Date'], y: filtered_data['Profit'], type: 'scatter', mode: 'lines+markers', name: 'Profit' } ] content_type :json chart_data.to_json end
This updated script defines two routes: one for serving the HTML template (‘/’) and another for fetching data (‘/data’). When the user selects a date range in the dashboard, an AJAX request is made to the /data route, which filters the data and responds with JSON data suitable for Plotly.
4.3. Running the Enhanced Dashboard
To run the enhanced dashboard, execute the following command:
bash ruby dashboard.rb
Access the dashboard in your web browser at http://localhost:4567. You can now select a date range using the date picker, and the chart will update dynamically to display data for the chosen range.
5. Deploying Your Ruby Dashboard
Once you’ve created and fine-tuned your interactive data dashboard, you may want to deploy it for broader access. Here are a few deployment options to consider:
5.1. Heroku
Heroku is a cloud platform that makes it easy to deploy web applications, including Ruby applications. You can deploy your Sinatra-based dashboard to Heroku with minimal effort. Ensure you have the Heroku CLI installed, create a Procfile in your project directory with the following content:
bash web: bundle exec ruby dashboard.rb -p $PORT
Then, follow Heroku’s documentation to create a new Heroku app and deploy your dashboard.
5.2. Docker
You can containerize your Ruby dashboard using Docker, allowing you to deploy it to various cloud platforms or your own server infrastructure. Create a Dockerfile in your project directory with the following content:
Dockerfile # Use an official Ruby runtime as a parent image FROM ruby:2.7 # Set the working directory WORKDIR /app # Copy the Gemfile and Gemfile.lock into the image COPY Gemfile Gemfile.lock ./ # Install Ruby dependencies RUN bundle install # Copy the rest of the application code into the image COPY . . # Expose the port that the application will run on EXPOSE 4567 # Start the application CMD ["bundle", "exec", "ruby", "dashboard.rb", "-p", "4567"]
Build the Docker image and run it:
bash docker build -t my-dashboard . docker run -p 4567:4567 my-dashboard
5.3. Cloud Hosting
Consider using cloud hosting services like AWS, Google Cloud, or Azure to deploy your Ruby dashboard. These platforms provide scalable infrastructure and various deployment options, including virtual machines and container services.
Conclusion
In this blog post, we’ve explored the process of creating interactive data dashboards with Ruby. We discussed why Ruby is a suitable choice for building dashboards and walked through setting up your development environment, creating a basic dashboard, and adding interactivity. By following the steps outlined here, you can create custom data dashboards that empower your organization to gain valuable insights from complex datasets. Whether you’re visualizing financial data, monitoring key performance indicators, or tracking user engagement, Ruby offers a versatile and enjoyable way to build interactive data dashboards. So, roll up your sleeves, dive into the code, and start visualizing your data with Ruby today!
Table of Contents
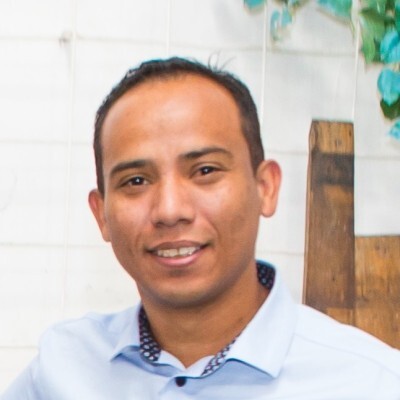
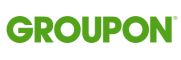