Ruby for Internet of Things (IoT): Connecting Devices and Collecting Data
The Internet of Things (IoT) has revolutionized the way we interact with the world around us. From smart thermostats and wearable devices to industrial sensors and connected vehicles, IoT technology has made its way into various aspects of our lives. At the heart of these interconnected devices lies the need for efficient communication, data collection, and analysis. In this blog post, we’ll explore how Ruby, a dynamic and versatile programming language, plays a significant role in building IoT applications. We’ll delve into connecting devices, collecting data, and leveraging Ruby’s strengths to create robust IoT solutions.
Table of Contents
1. Understanding the Role of Ruby in IoT
Before we dive into the technical details, let’s understand why Ruby is a suitable choice for IoT projects. Ruby’s simplicity and expressiveness make it an ideal candidate for rapid prototyping and development. Its object-oriented nature and extensive community-driven libraries enhance its capabilities in various domains, including IoT. With a focus on readability and developer-friendly syntax, Ruby minimizes the learning curve and encourages collaboration among teams.
2. Connecting Devices to the Network
Connecting devices to the network is the foundation of any IoT application. Ruby, with its built-in networking libraries and frameworks, simplifies this task considerably. One popular library for network communication is Socket. Let’s take a look at a basic example of using Socket to establish a connection between a client and a server:
ruby # Server side require 'socket' server = TCPServer.new(1234) loop do client = server.accept client.puts "Hello, IoT device!" client.close end ruby # Client side require 'socket' client = TCPSocket.new('localhost', 1234) response = client.gets puts response client.close
In this example, the server listens on port 1234, and when a client connects, it sends a greeting message. The client, in turn, connects to the server and receives the message. While this is a simple illustration, it showcases how Ruby’s Socket library can be utilized to establish basic communication between devices.
For more complex scenarios, where security and scalability are critical, Ruby developers can leverage frameworks like EventMachine or Celluloid. These frameworks provide abstractions for asynchronous programming, making it easier to manage multiple connections simultaneously and handle various protocols efficiently.
3. Collecting and Managing Data
Once devices are connected, the next challenge is collecting, managing, and processing the data they generate. Ruby offers several options for data storage, analysis, and visualization, allowing developers to build powerful IoT data pipelines.
3.1. Storing Data with Databases
Databases are integral to storing and retrieving IoT data. Ruby’s ActiveRecord library, commonly used with the Ruby on Rails framework, simplifies database interactions through an object-relational mapping (ORM) approach. This allows developers to work with databases using Ruby objects, reducing the complexity of SQL queries.
ruby class SensorData < ActiveRecord::Base end # Create a new data entry data_entry = SensorData.new(value: 25.6, timestamp: Time.now) data_entry.save # Retrieve data latest_data = SensorData.order(timestamp: :desc).first puts "Latest temperature reading: #{latest_data.value}"
For IoT projects that demand high-speed data ingestion and low-latency queries, InfluxDB can be an excellent choice. InfluxDB is a purpose-built time-series database that efficiently handles timestamped data, making it suitable for sensor data, metrics, and event tracking.
3.2. Data Analysis and Visualization
IoT data becomes valuable when it’s analyzed and transformed into actionable insights. Ruby supports various data analysis and visualization libraries that facilitate this process. Numo::NArray provides support for numerical arrays, making it useful for performing mathematical operations on sensor data.
ruby require 'numo/narray' temperature_readings = Numo::DFloat[23.4, 24.1, 22.8, 25.6, 26.3] average_temperature = temperature_readings.mean puts "Average temperature: #{average_temperature}"
For creating interactive visualizations, Rubyplot and GnuplotRB enable developers to generate graphs and charts from data. These libraries can aid in identifying trends, anomalies, and patterns within IoT data.
4. Handling Real-time Communication
Many IoT applications require real-time communication for tasks such as remote control, alerts, and monitoring. Ruby supports the implementation of real-time communication through technologies like WebSockets. The websocket-driver gem provides WebSocket support, allowing bidirectional communication between clients and servers.
ruby require 'websocket/driver' # Server side server = TCPServer.new(3000) loop do client = server.accept driver = WebSocket::Driver.server(client) driver.on :message do |event| driver.text "Received: #{event.data}" end driver.start end ruby require 'websocket/driver' # Client side require 'socket' require 'websocket/driver' client = TCPSocket.new('localhost', 3000) driver = WebSocket::Driver.client(client) driver.on :message do |event| puts "Server says: #{event.data}" end driver.start
In this example, the server and client exchange messages using WebSockets, enabling real-time communication between devices and servers.
5. Securing IoT Applications
Security is a paramount concern in IoT applications, as compromised devices can lead to severe consequences. Ruby provides various tools and practices to enhance the security of IoT systems.
5.1. Encryption and Authentication
Ruby supports encryption and authentication through libraries like OpenSSL. Using SSL/TLS protocols ensures that data transmitted between devices and servers remains confidential and tamper-proof.
ruby require 'openssl' private_key = OpenSSL::PKey::RSA.new(File.read('private_key.pem')) certificate = OpenSSL::X509::Certificate.new(File.read('certificate.pem')) context = OpenSSL::SSL::SSLContext.new context.cert = certificate context.key = private_key server = TCPServer.new(443) ssl_server = OpenSSL::SSL::SSLServer.new(server, context) loop do client = ssl_server.accept # Handle encrypted communication end
5.2. Access Control and Authorization
Implementing access control mechanisms is crucial to prevent unauthorized access to IoT devices and data. Ruby frameworks like Devise provide user authentication and authorization features, allowing developers to define user roles and permissions effectively.
Conclusion
Ruby’s versatility, ease of use, and extensive library ecosystem make it a valuable tool for building IoT applications. From connecting devices to collecting and processing data, Ruby empowers developers to create robust and efficient solutions. While challenges like security and real-time communication exist, Ruby’s support for encryption, authentication, and communication protocols enables developers to address these challenges effectively. As the Internet of Things continues to shape our world, Ruby remains a relevant and powerful choice for developers looking to create innovative IoT solutions. Whether you’re a seasoned Ruby developer or new to the language, exploring its potential in the realm of IoT can open up exciting possibilities for the future.
Table of Contents
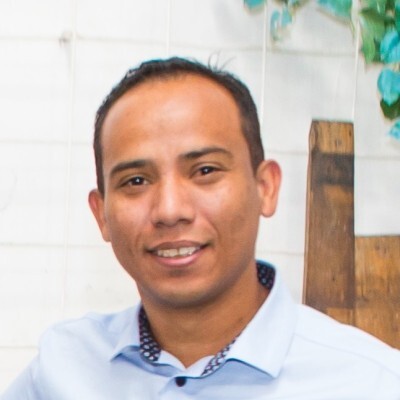
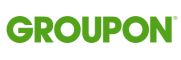