Integrating Ruby with Other Languages: Interoperability Techniques
Ruby is a versatile and expressive programming language known for its simplicity and elegance. However, there may be situations where you need to leverage the strengths of other programming languages to achieve specific tasks. Fortunately, Ruby offers various techniques for seamless integration with other languages. In this blog, we will delve into the world of Ruby’s interoperability with other languages, exploring techniques like Foreign Function Interface (FFI), C extensions, and working with web APIs. By the end, you’ll have a better understanding of how to combine the power of Ruby with the unique capabilities of other languages.
Table of Contents
1. Why Integrate Ruby with Other Languages?
Before we dive into the technical aspects, let’s briefly discuss the reasons why you might want to integrate Ruby with other languages.
- Performance Optimization: While Ruby is excellent for writing clean and maintainable code, it may not always be the most performant option for certain tasks. By integrating Ruby with a low-level language, you can offload performance-critical parts of your application for better execution speed.
- Legacy Code Integration: If you have a legacy codebase written in another language, you might want to incorporate Ruby into the mix without rewriting everything from scratch. This way, you can benefit from Ruby’s modern features while still utilizing existing functionality.
- Accessing Specific Libraries: Some libraries or frameworks might only be available in other languages. By integrating Ruby with these languages, you can tap into a broader range of tools and resources.
- Domain-specific Tasks: Certain domains may require specialized languages or tools. Integrating Ruby with such languages can provide a more comprehensive and tailored solution.
Now that we understand the motivations behind language integration let’s explore the various techniques Ruby offers for interoperability.
2. Foreign Function Interface (FFI)
The Foreign Function Interface (FFI) is a powerful mechanism that allows Ruby to call functions implemented in other languages directly. FFI provides a bridge between Ruby and native libraries without the need for writing C extensions. This means you can interact with dynamic libraries (.dll, .so, or .dylib) from Ruby code seamlessly.
2.1. Setting Up FFI
To use FFI, you’ll need to have the appropriate native library installed on your system. FFI comes pre-installed with Ruby 2.0 and later versions, so no additional gems are required.
Example: Using FFI to Call C Functions
Let’s demonstrate how to use FFI with a simple example. Assume we have a C library with a function to add two integers, and we want to call that function from Ruby.
C Code (add.c)
c int add(int a, int b) { return a + b; }
Ruby Code
ruby require 'ffi' module MyCFunctions extend FFI::Library ffi_lib './add.so' # Assuming add.so is the compiled shared library from add.c attach_function :add, [:int, :int], :int end result = MyCFunctions.add(3, 5) puts "Result: #{result}" # Output: Result: 8
In this example, we defined the C function add that takes two integers and returns their sum. Using the ffi_lib and attach_function methods from the FFI module, we loaded the C library and attached the add function to Ruby, enabling us to call it directly.
2.2. Pros and Cons of FFI
Pros:
- No need to write C extensions, making it easier to work with native libraries.
- Platform-independent as it relies on the underlying OS’s dynamic linking mechanism.
- Simplified memory management compared to C extensions.
Cons:
- May have some performance overhead compared to C extensions.
- Error handling can be challenging, as FFI doesn’t provide automatic memory management.
3. C Extensions
Ruby allows you to write C extensions, which are pieces of C code that can be compiled into native shared libraries and loaded directly into Ruby. C extensions offer a way to integrate Ruby tightly with C code and are especially useful for performance-critical tasks.
3.1. Creating a C Extension
Creating a C extension involves writing C code that adheres to the Ruby Extension API. The API provides macros and functions that facilitate communication between C and Ruby. Let’s walk through an example of creating a simple C extension to calculate the factorial of a number.
Example: Calculating Factorial with a C Extension
C Extension Code (factorial.c)
c #include "ruby.h" // Method Definition: factorial(n) VALUE method_factorial(VALUE self, VALUE num) { int n = NUM2INT(num); long result = 1; for (int i = 1; i <= n; i++) { result *= i; } return LONG2NUM(result); } // Ruby Method Definition: factorial(n) void Init_factorial() { VALUE FactorialModule = rb_define_module("Factorial"); rb_define_singleton_method(FactorialModule, "factorial", method_factorial, 1); }
Ruby Usage
ruby # Load the C extension require_relative 'factorial' # Call the factorial method from Ruby result = Factorial.factorial(5) puts "Factorial of 5: #{result}" # Output: Factorial of 5: 120
In this example, we created a C extension called factorial with a method named method_factorial. The C extension is initialized using the Init_factorial function, and the rb_define_singleton_method function connects the C method with the Ruby method.
3.2. Pros and Cons of C Extensions
Pros:
- Excellent performance due to the direct integration with C code.
- Full access to Ruby internals and C libraries, providing extensive functionality.
- Suitable for complex and CPU-intensive tasks.
Cons:
- Writing and debugging C code can be more challenging than Ruby.
- Platform-specific compilation and potential portability issues.
- Risk of memory leaks and segmentation faults if not handled carefully.
4. Web APIs and Ruby
Another common approach to integrating Ruby with other languages is through web APIs. Many languages provide web APIs that allow communication over HTTP or other protocols. By utilizing these APIs, you can interact with the functionalities provided by the target language or service.
4.1. RESTful APIs
Representational State Transfer (REST) is a popular architectural style for designing networked applications. It leverages the HTTP protocol for communication and uses standard CRUD (Create, Read, Update, Delete) operations for data manipulation.
Example: Using a RESTful API with Ruby
Let’s imagine that we have a Python-based web service exposing a RESTful API to perform basic arithmetic operations. We can use Ruby to interact with this API and perform calculations remotely.
Python-based Web Service
python # app.py from flask import Flask, request app = Flask(__name__) @app.route('/add', methods=['POST']) def add(): data = request.json result = data['a'] + data['b'] return {"result": result} @app.route('/subtract', methods=['POST']) def subtract(): data = request.json result = data['a'] - data['b'] return {"result": result}
Ruby Client
ruby require 'net/http' require 'json' def perform_calculation(url, endpoint, data) uri = URI("#{url}/#{endpoint}") http = Net::HTTP.new(uri.host, uri.port) request = Net::HTTP::Post.new(uri.path, 'Content-Type' => 'application/json') request.body = data.to_json response = http.request(request) JSON.parse(response.body)['result'] end url = 'http://example.com' data = { a: 10, b: 5 } add_result = perform_calculation(url, 'add', data) subtract_result = perform_calculation(url, 'subtract', data) puts "Addition result: #{add_result}" # Output: Addition result: 15 puts "Subtraction result: #{subtract_result}" # Output: Subtraction result: 5
In this example, we created a simple Python-based web service using Flask. The service exposes two endpoints: /add and /subtract. We then implemented a Ruby client that uses the net/http library to make HTTP POST requests to these endpoints, passing the necessary data and receiving the results.
4.2. Pros and Cons of Web APIs
Pros:
- Language-agnostic approach; can be used to interact with services in any language.
- Simplified integration with remote services and applications.
- Allows leveraging external capabilities without the need to manage native code.
Cons:
- Communication overhead due to network requests.
- Requires an internet connection or access to the remote service.
- May have limitations in terms of functionality exposed by the API.
Conclusion
Integrating Ruby with other programming languages opens up a world of possibilities for developers. Whether you want to improve performance, leverage existing codebases, access specialized libraries, or interact with remote services, Ruby provides various techniques for seamless interoperability.
In this blog, we explored three essential techniques: Foreign Function Interface (FFI), which allows Ruby to call functions implemented in other languages directly; C Extensions, which enable the creation of native shared libraries from C code; and interacting with external languages and services using Web APIs. Each approach has its advantages and limitations, and the choice depends on the specific requirements of your project.
By combining the elegance of Ruby with the strengths of other languages, you can build powerful, efficient, and flexible applications that cater to a wide range of use cases. Happy coding!
Table of Contents
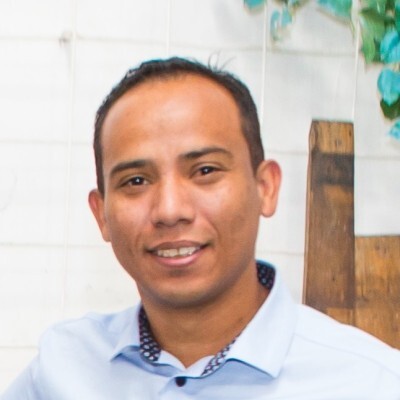
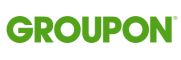