Exploring Ruby’s IoT Frameworks: Controlling Devices and Sensors
The Internet of Things (IoT) has transformed the way we interact with technology, allowing us to seamlessly connect and control devices remotely. Ruby, a versatile and elegant programming language, has made its mark in this ever-evolving landscape by offering robust frameworks that simplify the development of IoT applications. In this blog post, we’ll take a deep dive into Ruby’s IoT frameworks and explore how they enable us to effortlessly control devices and gather data from sensors. Along the way, we’ll provide code samples to showcase the practical implementation of these frameworks in real-world scenarios.
Table of Contents
1. Understanding IoT and its Significance
Before we delve into the specifics of Ruby’s IoT frameworks, let’s briefly recap what the Internet of Things is and why it matters. IoT refers to the network of interconnected devices that communicate and exchange data, often without human intervention. This technology has found applications in various industries, from smart homes and industrial automation to healthcare and agriculture. By enabling devices to collect, analyze, and share data, IoT opens up opportunities for enhanced efficiency, convenience, and innovation.
2. Introducing Ruby’s IoT Frameworks
Ruby, known for its elegant syntax and developer-friendly environment, offers several frameworks that cater to IoT development. Two prominent frameworks are Cylon.js and Artifice. Let’s take a closer look at each of them.
2.1. Cylon.js: Bridging Devices and Ruby
Cylon.js is a versatile JavaScript framework that enables developers to build IoT applications using various programming languages, including Ruby. It supports a wide range of hardware platforms, making it an excellent choice for controlling diverse devices and sensors.
2.1.1. Installing Cylon.js
To get started with Cylon.js, install the necessary dependencies using npm (Node Package Manager):
bash npm install cylon cylon-ruby
2.1.2. Controlling Devices with Cylon.js
Cylon.js provides a clean and intuitive syntax for controlling devices. Below is a simple example of how to use Cylon.js to control an LED connected to a Raspberry Pi using Ruby:
ruby require 'cylon' Cylon.robot do connection :raspi, adaptor: :raspi device :led, driver: :led, pin: 7 work do every 1.second do led.toggle end end end Cylon.start
In this example, we create a robot that connects to a Raspberry Pi and controls an LED connected to pin 7. The every method toggles the LED’s state every second.
2.2. Artifice: Building IoT Applications with Ease
Artifice is a Ruby-based IoT framework that focuses on simplicity and ease of use. It provides a set of tools for communicating with devices and sensors while abstracting away much of the complex setup.
2.2.1. Installing Artifice
To begin using Artifice, simply install it via RubyGems:
bash gem install artifice
2.2.2. Interacting with Devices using Artifice
Artifice simplifies the process of interacting with devices and sensors. Below is an example demonstrating how to use Artifice to read data from a temperature sensor connected to an Arduino:
ruby require 'artifice' Artifice.device('arduino') do sensor :temperature, type: :analog, pin: 0 end Artifice.run do temperature = Artifice.devices[:arduino].read_temperature puts "Current Temperature: #{temperature}°C" end
In this example, we define an Arduino device with a temperature sensor. The read_temperature method reads the temperature data from the analog pin and displays it.
3. Real-World Applications
Now that we have a grasp of Cylon.js and Artifice, let’s explore how these frameworks can be applied in real-world scenarios.
3.1. Smart Home Automation with Cylon.js
Cylon.js can be harnessed to create sophisticated smart home systems. Imagine controlling your lights, thermostat, and security cameras from a single platform. Here’s a snippet of code illustrating how you might control smart lights using Cylon.js:
ruby require 'cylon' Cylon.robot do connection :hue, adaptor: :hue, host: '192.168.1.100', username: 'your_username' device :lights, driver: :hue-lights, connection: :hue work do lights.toggle after 5.seconds do lights.brightness(50) lights.color('#00FF00') end end end Cylon.start
In this example, the robot connects to Philips Hue smart lights and toggles them on and off. After a delay, it adjusts the brightness and changes the color.
3.2. Environmental Monitoring with Artifice
Artifice can be employed to build environmental monitoring solutions. For instance, you could create a weather station that gathers data from various sensors and displays it in a user-friendly format. Here’s a simplified version of how you might read data from a weather sensor using Artifice:
ruby require 'artifice' Artifice.device('weather_station') do sensor :temperature, type: :analog, pin: 0 sensor :humidity, type: :digital, pin: 2 end Artifice.run do temperature = Artifice.devices[:weather_station].read_temperature humidity = Artifice.devices[:weather_station].read_humidity puts "Current Temperature: #{temperature}°C" puts "Current Humidity: #{humidity}%" end
In this example, the weather_station device is equipped with both temperature and humidity sensors. The code reads and displays the current temperature and humidity.
Conclusion
Ruby’s IoT frameworks, Cylon.js, and Artifice, provide developers with powerful tools to build sophisticated IoT applications. These frameworks simplify the process of controlling devices and gathering data from sensors, allowing developers to focus on creating innovative solutions without getting bogged down by the intricacies of hardware communication. By leveraging the elegance of Ruby, you can create efficient and robust IoT applications that have a real impact on various industries. Whether you’re venturing into smart home automation or environmental monitoring, these frameworks open up a world of possibilities for your IoT projects. So, why wait? Dive into Ruby’s IoT frameworks and start exploring the limitless potential of the Internet of Things.
Table of Contents
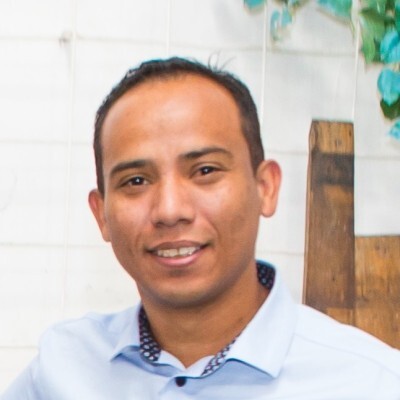
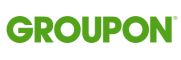