How to work with JSON data in Ruby?
Working with JSON data in Ruby is straightforward thanks to the built-in JSON library, which provides methods to parse JSON data into Ruby objects and vice versa. Here are the key steps to work with JSON data in Ruby:
Parsing JSON:
- Require the JSON Library: Ensure that the JSON library is available in your Ruby environment by requiring it at the beginning of your script or program: `require ‘json’`.
- Parsing JSON into Ruby Objects: To parse JSON data into Ruby objects (e.g., hashes and arrays), use the `JSON.parse` method. Pass the JSON string as an argument to this method. For example:
```ruby require 'json' json_string = '{"name": "John", "age": 30, "city": "New York"}' data = JSON.parse(json_string) puts data["name"] # Output: John ```
Generating JSON:
- Creating Ruby Data: First, create Ruby data that you want to convert to JSON format. This can be a hash, an array, or any combination of these.
- Converting to JSON: Use the `JSON.generate` method (or its alias `to_json`) to convert Ruby data into a JSON-formatted string. For example:
```ruby require 'json' ruby_data = { "name" => "Alice", "age" => 25, "city" => "Los Angeles" } json_string = JSON.generate(ruby_data) puts json_string ```
Reading and Writing JSON Files:
You can also work with JSON files using Ruby. To read a JSON file, you can use `File.read` to read the file’s contents as a string, and then parse it using `JSON.parse`. To write JSON data to a file, you can use `File.write` after converting your Ruby data to JSON format.
Here’s an example of reading and writing JSON data to/from a file:
```ruby require 'json' # Reading JSON from a file json_file_contents = File.read('data.json') parsed_data = JSON.parse(json_file_contents) # Modifying data parsed_data["age"] += 1 # Writing JSON back to the file File.write('data.json', JSON.generate(parsed_data)) ```
Ruby’s JSON library makes it easy to work with JSON data by providing methods for both parsing JSON into Ruby objects and generating JSON from Ruby data. This is particularly useful when dealing with web APIs, configuration files, or data interchange between systems that use JSON as a common format.
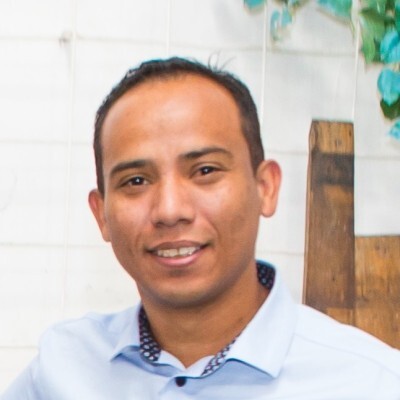
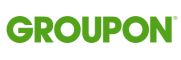