What is a lambda (or proc) in Ruby?
In Ruby, a lambda and a proc are both objects that represent blocks of code that can be stored in variables and passed around as objects. They are essentially anonymous functions, allowing you to encapsulate a piece of code for later execution. While they serve a similar purpose, there are subtle differences between them.
Lambda:
– A lambda in Ruby is an instance of the `Proc` class, created using the `lambda` keyword or the `->` (stabby lambda) syntax.
– Lambdas enforce strict argument arity, meaning they will raise an error if the number of arguments provided during invocation does not match the expected number.
– They return control to the calling method when executed.
– Lambdas are often used in situations where you need to pass a block of code as an argument to another method, or when you want to create an anonymous function that behaves consistently.
Here’s an example of a lambda:
```ruby add = lambda { |x, y| x + y } result = add.call(3, 4) # result will be 7 ```
Proc:
– A proc is also an instance of the `Proc` class, created using the `proc` keyword or the `Proc.new` method.
– Procs have more relaxed argument handling; they don’t enforce strict arity, which means if you pass the wrong number of arguments, they will not raise an error but will rather fill in missing arguments with `nil` and ignore extras.
– They return from the enclosing method where they were defined when executed.
– Procs are often used when you want more flexibility in argument handling or when you want to create a reusable block of code.
Here’s an example of a proc:
```ruby multiply = proc { |x, y| x * y } result = multiply.call(3, 4) # result will be 12 ```
Both lambdas and procs are useful for creating anonymous functions in Ruby, but they differ in how they handle argument arity and how they handle the return from the enclosing method. Your choice between them depends on the specific requirements of your code.
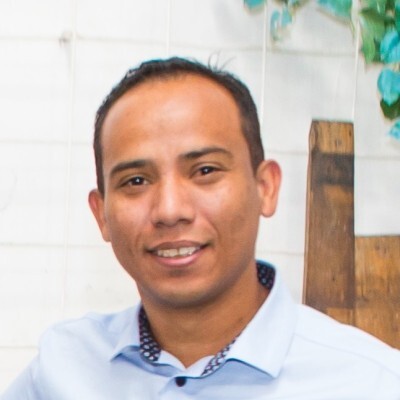
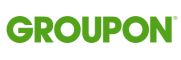