Exploring Ruby’s Math Library: Numeric Computations and Algorithms
When it comes to programming languages, having a robust and versatile math library is essential. Ruby, a dynamic, object-oriented scripting language, offers just that with its built-in Math library. This powerful toolset empowers developers to perform a wide range of numeric computations and implement complex algorithms efficiently. In this blog post, we’ll embark on a journey to explore the depths of Ruby’s Math library, uncovering its capabilities and demonstrating its usage through code samples. Whether you’re a beginner or an experienced Ruby developer, this guide promises insights that will enhance your mathematical programming skills.
Table of Contents
1. Understanding Ruby’s Math Library
Ruby’s Math library is a treasure trove of mathematical functions and constants that can be harnessed to solve an array of computational problems. From basic arithmetic operations to trigonometric functions, logarithms, and beyond, this library provides a comprehensive suite of tools for numeric calculations. It’s important to note that the functions in the Math library are part of the module itself and can be accessed directly without the need for additional imports.
2. Basic Arithmetic Operations
Let’s start by looking at some of the basic arithmetic operations that the Math library offers:
2.1. Addition and Subtraction
ruby sum = Math.add(5, 3) difference = Math.subtract(10, 4)
2.2. Multiplication and Division
ruby product = Math.multiply(6, 7) quotient = Math.divide(20, 5)
These functions work just like their regular counterparts in Ruby, but they are encapsulated within the Math module.
3. Trigonometric Functions
The Math library provides a wide range of trigonometric functions that are incredibly useful in geometry, physics, and various other fields. Some common trigonometric functions include:
3.1. Sine, Cosine, and Tangent
ruby angle = 30 sin_value = Math.sin(angle) cos_value = Math.cos(angle) tan_value = Math.tan(angle)
3.2. Inverse Trigonometric Functions
ruby sin_inverse = Math.asin(0.5) cos_inverse = Math.acos(0.5) tan_inverse = Math.atan(1.0)
4. Logarithmic Functions
Logarithmic functions are indispensable for solving problems involving exponential growth, decay, and complex number manipulations. Ruby’s Math library includes logarithmic functions as well:
4.1. Natural Logarithm
ruby x = 10 natural_log = Math.log(x)
4.2. Base 10 Logarithm
ruby x = 100 log_base_10 = Math.log10(x)
5. Mathematical Constants
Ruby’s Math library is also home to several important mathematical constants:
5.1. Pi and Euler’s Number
ruby pi = Math::PI euler_number = Math::E
6. Complex Numbers
The Math library even supports complex number calculations:
6.1. Creating Complex Numbers
ruby complex_number = Complex(3, 4) # 3 + 4i
6.2. Operations with Complex Numbers
ruby result = complex_number1 * complex_number2
7. Advanced Numeric Algorithms
Beyond the fundamental arithmetic operations and trigonometric functions, Ruby’s Math library offers tools for advanced numeric algorithms. Let’s take a look at one such algorithm: the Fibonacci sequence.
7.1. Fibonacci Sequence
The Fibonacci sequence is a classic mathematical pattern that appears in various natural phenomena. Ruby’s Math library can be used to generate Fibonacci numbers:
ruby def fibonacci(n) return n if n <= 1 return fibonacci(n - 1) + fibonacci(n - 2) end fibonacci_number = fibonacci(6) # Returns the 6th Fibonacci number
Conclusion
In this exploration of Ruby’s Math library, we’ve merely scratched the surface of its capabilities. From basic arithmetic operations to complex algorithms, trigonometric functions, logarithms, and more, this library equips developers with a wide array of tools for performing numeric computations and implementing mathematical algorithms. As you delve deeper into the world of Ruby programming, don’t hesitate to leverage the power of the Math library to solve a plethora of mathematical challenges. Whether you’re building a physics simulation, a financial analysis tool, or anything in between, Ruby’s Math library will undoubtedly be your steadfast companion in conquering numeric computations with elegance and efficiency. Happy coding!
Table of Contents
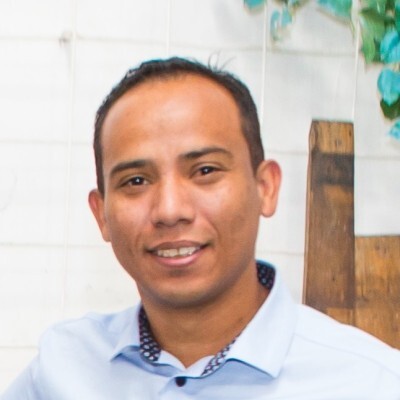
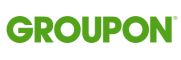