Understanding Memory Management in Ruby: Garbage Collection
Memory management is a critical aspect of any programming language, and Ruby is no exception. As a dynamically-typed and object-oriented language, Ruby provides developers with a high level of productivity and flexibility. However, this flexibility comes at the cost of effective memory management, as developers don’t have to worry about memory allocation and deallocation explicitly. Instead, Ruby employs a garbage collection system to automatically handle memory management, making the developer’s life easier.
Table of Contents
In this blog, we will delve into the intricacies of memory management in Ruby, with a particular focus on the Garbage Collection (GC) mechanism. We’ll explore how GC works, different GC algorithms, and tips to optimize memory usage in Ruby programs.
1. What is Memory Management?
Memory management refers to the process of controlling and coordinating computer memory, allowing programs to allocate and deallocate memory when needed. Efficient memory management is crucial for preventing memory leaks, optimizing performance, and avoiding system crashes due to running out of memory.
In low-level languages like C and C++, developers need to explicitly allocate and deallocate memory using functions like malloc() and free(). However, in high-level languages like Ruby, memory management is abstracted away from developers, and they don’t have to worry about memory allocation and deallocation directly.
2. The Need for Garbage Collection
Since Ruby is an object-oriented language, objects are created dynamically during the program’s execution. However, developers might lose track of objects that are no longer needed, leading to memory leaks. A memory leak occurs when memory that is no longer being used is not released, causing the program’s memory footprint to grow over time.
To tackle memory leaks and efficiently manage memory, Ruby uses a Garbage Collection (GC) system. GC is an automated process that identifies and reclaims memory occupied by objects that are no longer accessible or referenced by the program. By automatically freeing up memory, GC helps ensure the program’s memory usage remains under control.
3. How Garbage Collection Works in Ruby
Ruby’s garbage collection employs a “Mark and Sweep” algorithm to identify and reclaim unused memory. Let’s understand the basic steps of this algorithm:
3.1. Mark and Sweep Algorithm
- Mark Phase: The garbage collector starts by marking all the objects that are still accessible and in use. It begins with root objects, which include global variables and objects referenced directly by the program’s stack. The GC traverses through the object graph, marking objects as “in use” by setting a flag on each reachable object.
- Sweep Phase: After marking the accessible objects, the GC performs a sweep through the entire heap, looking for unmarked (unused) objects. It reclaims the memory occupied by these unmarked objects and returns it to the free memory pool, making it available for future object allocations.
The Mark and Sweep algorithm efficiently reclaims memory occupied by unreachable objects, but it comes with some drawbacks. The major concern is the stop-the-world nature of the algorithm, meaning the program’s execution is paused during the GC process. This can cause noticeable performance issues in applications with high memory usage.
3.2. Generational Garbage Collection
To improve garbage collection performance, Ruby uses a generational garbage collection approach. The idea behind generational GC is that most objects in a program become unreachable shortly after being created. By dividing objects into different generations based on their age, Ruby can prioritize garbage collection efforts on specific generations, reducing the overall work required.
Ruby’s generational garbage collection is divided into three generations:
- Young Generation (Young Objects): This generation contains recently created objects. The garbage collector performs garbage collection frequently on this generation, as most of the objects here become unreachable quickly.
- Intermediate Generation (Intermediate Objects): Objects that have survived a few garbage collection cycles in the young generation get promoted to the intermediate generation. The garbage collector performs less frequent GC on this generation compared to the young generation.
- Old Generation (Mature Objects): Objects that have survived several garbage collection cycles in the intermediate generation get promoted to the old generation. GC on the old generation is infrequent, as these objects tend to have longer lifetimes and lower chances of becoming unreachable.
By focusing garbage collection efforts on the younger generations, Ruby can minimize the impact of GC on application performance.
4. Manual Memory Management in Ruby
While Ruby’s garbage collection system efficiently manages memory, developers can still take some manual steps to optimize memory usage further. Some of these steps include:
4.1. Use nil for Unreferenced Variables:
Assigning nil to variables that are no longer needed can help the garbage collector reclaim memory occupied by those objects. For example:
ruby big_data = some_large_data_processing() # Process big_data…
big_data = nil # Set to nil when no longer needed
4.2. Explicitly Removing Object References:
When an object is no longer needed and can be garbage collected, explicitly remove its reference to speed up memory reclamation:
ruby def some_method obj = SomeObject.new # Use obj... obj = nil # Remove the reference when done end
4.3. Manual GC Invocation:
Though rarely necessary, Ruby provides a way to manually trigger garbage collection using GC.start. However, it’s essential to use this cautiously, as it can interfere with the automatic GC process and may not lead to performance improvements in most cases.
5. Garbage Collection Configuration
Ruby’s garbage collector can be fine-tuned by tweaking specific environment variables. These variables control various aspects of garbage collection, such as enabling/disabling GC, setting thresholds for each generation, and adjusting other parameters. Some of the important environment variables related to GC configuration are:
- RUBY_GC_HEAP_GROWTH_MAX_SLOTS: Controls the maximum heap slots to grow during GC.
- RUBY_GC_HEAP_INIT_SLOTS: Initial heap slots for Ruby.
- RUBY_GC_HEAP_OLDOBJECT_LIMIT_FACTOR: Sets the limit of old objects growth based on the heap size.
- RUBY_GC_MALLOC_LIMIT: Sets the number of allocated objects that trigger a garbage collection.
6. Optimizing Memory Usage in Ruby
To build efficient and memory-friendly Ruby applications, consider the following optimization techniques:
6.1. Limiting Object Creation
Creating too many objects in a short period can strain the garbage collector and lead to frequent GC cycles. To minimize object creation, consider using object pooling or reusing objects wherever possible. Additionally, prefer mutable objects over immutable ones for operations that involve frequent changes, as mutable objects can be modified in place.
6.2. Avoiding Memory Leaks
Be mindful of circular references or unintended strong references that can prevent objects from being garbage collected. Use weak references when appropriate to allow objects to be collected even when there are references to them.
6.3. Using Symbols and Immutable Objects
Use symbols for frequently used string literals, as they are immutable and only have one copy in memory. Immutable objects, like frozen strings and frozen arrays, can also help in reducing memory overhead.
7. Memory Profiling Tools
When optimizing memory usage in Ruby, memory profiling tools can be invaluable. Tools like memory_profiler and ObjectSpace provide insights into memory consumption and help identify memory-intensive areas in the code.
Conclusion
Memory management is a crucial aspect of Ruby programming, and the garbage collection system plays a vital role in ensuring efficient memory usage. Understanding how garbage collection works and employing manual memory management techniques can lead to better-performing Ruby applications. By optimizing memory usage, developers can create Ruby programs that are not only performant but also scalable and reliable, even under heavy workloads. So go ahead and write memory-efficient Ruby code and unleash the full potential of this beautiful language!
Table of Contents
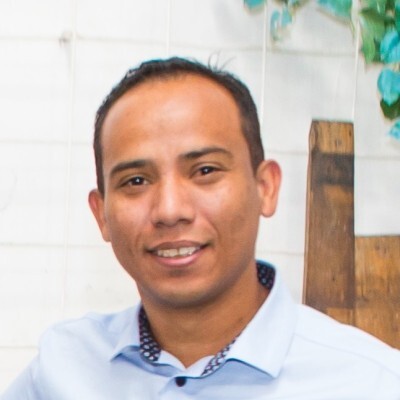
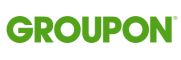