Harnessing the Power of Ruby’s Metaprogramming Abilities
Ruby is a dynamic, reflective, and object-oriented programming language that has gained popularity for its elegance, simplicity, and readability. As such, the demand to hire Ruby developers has seen a significant increase. Among its many features, Ruby’s metaprogramming abilities stand out, offering developers unprecedented power and flexibility. This makes Ruby developers highly versatile and capable of creating efficient, elegant solutions. Metaprogramming refers to the ability of a program to have knowledge of or manipulate itself, a feature that greatly enhances the capabilities of a Ruby developer.
In this blog post, we’ll dive into the world of Ruby metaprogramming, its benefits, and how to effectively harness its power whether you’re a seasoned Ruby developer or looking to hire Ruby developers for your project.
What is Metaprogramming?
Metaprogramming is the act of writing code that writes or manipulates other code in runtime. It’s a high level of abstraction that can reduce code redundancy, increase readability, and give developers powerful tools to create flexible code. Essentially, with metaprogramming, you’re writing code that generates or modifies other code.
In Ruby, everything is an object, and methods define the behavior of these objects. With metaprogramming, we can create methods dynamically, redefine them, or even remove them, all during runtime. Let’s start with some basic examples to see how it works.
Getting Started: `define_method`
To illustrate metaprogramming, let’s assume we’re creating a simple calculator. We can use `define_method` to dynamically create methods for addition, subtraction, multiplication, and division:
```ruby class Calculator ['add', 'subtract', 'multiply', 'divide'].each do |method| define_method(method) do |num1, num2| num1.send(method, num2) end end end
In this code, we’ve defined four methods using only three lines of code. The `define_method` function accepts a string or symbol that becomes the name of the new method. The block you pass into `define_method` is the body of the new method. This kind of dynamic method creation is a cornerstone of Ruby metaprogramming.
Diving Deeper: `method_missing`
Another important method in Ruby metaprogramming is `method_missing`. This is a built-in method that Ruby calls whenever it can’t find the method you’re trying to call on an object. We can override `method_missing` to handle undefined methods gracefully:
```ruby class SmartCalculator def method_missing(name, *args) return super unless ['add', 'subtract', 'multiply', 'divide'].include?(name.to_s) args.reduce { |memo, num| memo.send(name, num) } end end
Here, if we call a method that doesn’t exist but is one of our defined operations, we compute the operation; otherwise, we call `super`, which raises a `NoMethodError`. This enables us to define an almost unlimited number of methods dynamically.
`instance_eval` and `class_eval`
Two more methods used frequently in Ruby metaprogramming are `instance_eval` and `class_eval`. These methods evaluate a block or a string in the context of an instance or a class, respectively.
`instance_eval` changes the current object context to the object it’s called upon. This means we can dynamically add instance methods or instance variables to an object:
```ruby class Cat def initialize @sound = "Meow" end end garfield = Cat.new garfield.instance_eval do def speak @sound end end puts garfield.speak # Output: Meow
`class_eval`, also known as `module_eval`, operates similarly, but it allows us to add class methods or class variables:
```ruby Cat.class_eval do def self.species "Feline" end end puts Cat.species # Output: Feline
Ghost Methods and Dynamic Proxies
Ghost methods are methods that don’t actually exist but behave as though they do when called, thanks to `method_missing`. Dynamic proxies utilize this concept to forward method calls to another object.
Imagine a scenario where we have a `Document` object and a `ReadOnlyDocumentProxy` object that allows reading document data but not changing it:
```ruby class Document attr_accessor :content def initialize(content) @content = content end end class ReadOnlyDocumentProxy def initialize(document) @document = document end def method_missing(name, *args) check_access @document.send(name, *args) end def check_access raise "Read only access" if [:content=].include?(name) end end
This proxy can effectively control the access to our `Document` object and provides a powerful way to encapsulate and control object behavior dynamically.
Conclusion
Ruby’s metaprogramming abilities open the door to a whole new level of code abstraction and dynamism. While these concepts might seem complex and intimidating at first, they are powerful tools when used wisely. Understanding them will let you read, write and manipulate Ruby code more effectively. The true power of Ruby’s metaprogramming shines in developing domain-specific languages, building flexible APIs, and reducing code redundancy.
However, with great power comes great responsibility. This is a critical consideration when you plan to hire Ruby developers. Metaprogramming, while a powerful tool, can lead to code that’s hard to understand and debug if used unwisely. Therefore, it’s essential that if you are looking to hire Ruby developers, always strive for clarity in their code and use these metaprogramming tools sparingly and judiciously.
The journey into Ruby’s metaprogramming is a deep dive into the language’s object model. It helps to broaden your perspective as a Ruby developer and opens up possibilities you might not have imagined before. So go ahead and explore these concepts, harness the power of metaprogramming, and take your Ruby skills to the next level!
Table of Contents
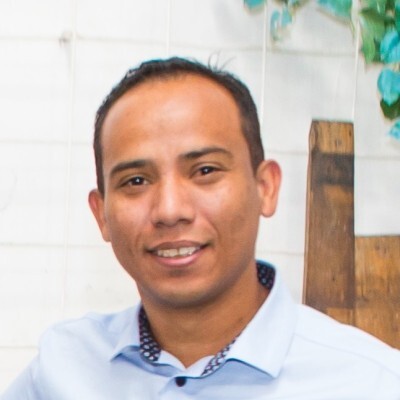
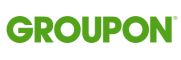