What is method chaining in Ruby?
Method chaining in Ruby is a powerful technique that allows you to call multiple methods on an object in a single line of code. It enables a more fluent and concise coding style by connecting method calls sequentially, with each method operating on the result of the previous one. This approach can lead to more readable and expressive code, as it eliminates the need for intermediate variables and simplifies complex operations.
In Ruby, method chaining is possible because most methods return the object they operate on, known as the receiver, allowing you to chain subsequent methods onto the result. Here’s an example:
```ruby # Without method chaining text = " Hello, World! " text = text.strip text = text.downcase text = text.gsub("world", "Ruby") # Using method chaining text = " Hello, World! ".strip.downcase.gsub("world", "Ruby") ```
In the second approach, `strip`, `downcase`, and `gsub` are chained together, making the code more concise and eliminating the need for intermediate assignments. Method chaining can be particularly useful when working with collections, as you can chain various operations like filtering, mapping, and reducing on enumerable objects.
It’s essential to be mindful of readability when using method chaining. While it can lead to concise code, overly long chains can become hard to follow. Striking a balance between brevity and clarity is crucial to maintain code maintainability and understandability. Overall, method chaining is a valuable technique in Ruby for simplifying and enhancing code readability.
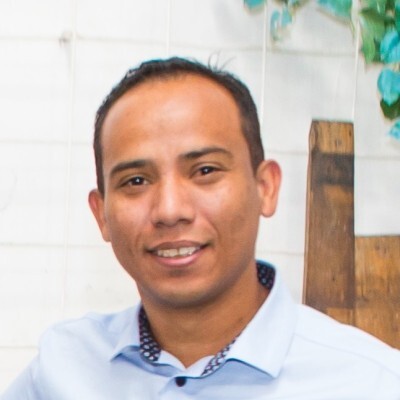
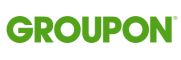