What is a mixin in Ruby?
In Ruby, a mixin is a way to share and reuse code among multiple classes without using traditional inheritance. It’s a powerful concept that promotes code modularity and helps avoid some of the limitations and complexities associated with single inheritance.
A mixin is essentially a module that contains a collection of methods that can be added to one or more classes. These methods can provide additional behavior, functionality, or attributes to those classes. Mixins are included in a class using the `include` keyword.
Here’s a simple example to illustrate how mixins work:
```ruby module Greetable def greet "Hello, #{@name}!" end end class Person include Greetable def initialize(name) @name = name end end class Animal include Greetable def initialize(name) @name = name end end person = Person.new("Alice") animal = Animal.new("Fluffy") puts person.greet # Output: Hello, Alice! puts animal.greet # Output: Hello, Fluffy! ```
In this example, we have a mixin called `Greetable` that defines a `greet` method. Both the `Person` and `Animal` classes include this mixin, allowing them to share the `greet` method without the need for a common superclass. This demonstrates how mixins enable code reuse and make it easy to add functionality to multiple classes independently.
Mixins are widely used in Ruby to implement cross-cutting concerns such as logging, authentication, and more. They promote a modular and flexible design, where classes can adopt and combine various mixins to create composite behavior, leading to cleaner and more maintainable code.
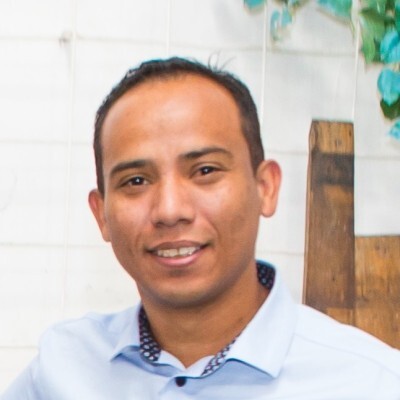
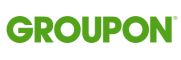