How to use modules in Ruby?
In Ruby, modules are a powerful tool for organizing and reusing code in a structured and modular way. They allow you to define collections of methods, constants, and classes without creating instances of those classes. Modules are often used to group related functionality together, provide namespaces, and achieve multiple inheritance-like behavior. Here’s how to use modules in Ruby:
- Defining Modules:
To define a module, use the `module` keyword followed by the module name and a block of code containing methods, constants, and class definitions. Module names are typically written in CamelCase with an initial uppercase letter.
```ruby module MathFunctions def add(a, b) a + b end def subtract(a, b) a - b end end ```
- Including Modules:
To use the functionality defined in a module, you include the module in a class using the `include` keyword. This makes all the module’s methods and constants available to instances of that class.
```ruby class Calculator include MathFunctions def calculate result = add(5, 3) puts result end end ```
In this example, the `Calculator` class includes the `MathFunctions` module, allowing instances of `Calculator` to use the `add` and `subtract` methods.
- Mixins and Multiple Modules:
Ruby supports multiple inheritance-like behavior through mixins. You can include multiple modules in a class, and they will all contribute their methods and constants.
```ruby class AdvancedCalculator include MathFunctions include TrigonometryFunctions def calculate result = add(5, 3) sine = sin(45) puts result, sine end end ```
Here, `AdvancedCalculator` includes both `MathFunctions` and `TrigonometryFunctions` modules, gaining access to methods from both.
- Namespace and Constants:
Modules are often used to create namespaces for constants and methods, preventing naming conflicts in larger applications.
```ruby module Geometry PI = 3.14159265359 def area(radius) PI * radius**2 end end ```
Here, the `Geometry` module provides a namespace for the constant `PI` and the `area` method.
Modules in Ruby are a powerful mechanism for code organization, reuse, and encapsulation. They enable you to create modular and maintainable code by grouping related functionality together and preventing naming conflicts. Whether you need to add functionality to multiple classes or organize your codebase more efficiently, modules are a valuable tool in Ruby development.
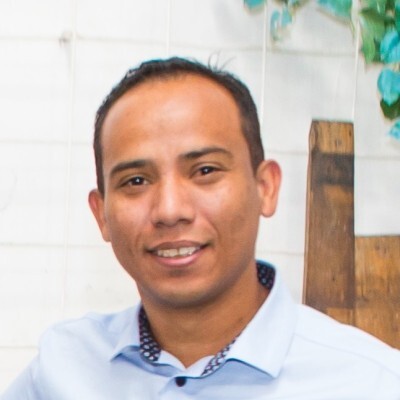
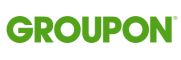