How to work with multi-threading in Ruby?
In Ruby, you can work with multi-threading to perform concurrent tasks using the `Thread` class from the standard library. Multi-threading allows you to execute multiple threads (smaller units of a program) concurrently, which can be especially useful for tasks like parallel processing and improving application responsiveness. Here’s a guide on how to work with multi-threading in Ruby:
- Require the ‘thread’ Library:
To use the `Thread` class, you need to require the ‘thread’ library at the beginning of your Ruby script or program:
```ruby require 'thread' ```
- Create Threads:
You can create threads by instantiating the `Thread` class and passing a block of code that you want to run concurrently. For example:
```ruby thread1 = Thread.new do # Code for the first thread end thread2 = Thread.new do # Code for the second thread end ```
- Join Threads:
The `join` method is used to wait for a thread to finish execution before proceeding. It’s a way to ensure that all threads have completed their tasks:
```ruby thread1.join thread2.join ```
- Run Threads Concurrently:
After creating threads, you can start them using the `start` method or simply let them run concurrently. Ruby’s Global Interpreter Lock (GIL) can limit true parallelism in multi-threaded Ruby programs, but threads can still be beneficial for I/O-bound tasks or parallel processing.
- Sharing Data:
When working with threads, be cautious about sharing data between threads. Ruby’s threads can share data, but this requires proper synchronization to avoid data corruption or race conditions. You can use tools like mutexes (`Mutex` class) to synchronize access to shared resources.
- Error Handling:
Exception handling in multi-threaded programs is crucial. Ensure that you have appropriate error handling and exception management in place to handle issues that might occur within threads.
- Thread Safety:
Keep in mind that not all Ruby libraries and gems are thread-safe. When using multi-threading, choose libraries and design your code with thread safety in mind to prevent unexpected behavior.
Working with multi-threading in Ruby can be powerful for improving the performance and responsiveness of your applications, particularly for I/O-bound or concurrent tasks. However, it’s important to be aware of potential pitfalls, such as shared resource management and thread safety, to write reliable multi-threaded code.
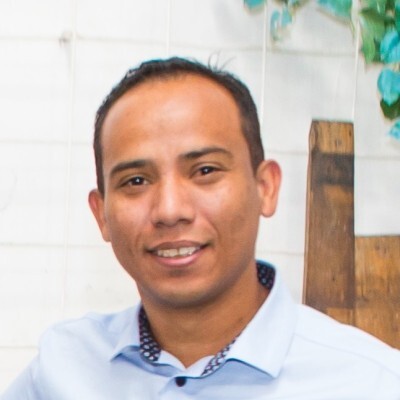
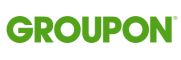