Exploring Ruby’s Multithreading Capabilities: Concurrency Made Easy
In the world of software development, efficiency and performance are paramount. As applications become more complex and data-intensive, the need to execute multiple tasks simultaneously becomes increasingly important. This is where multithreading comes into play, allowing developers to achieve concurrency and harness the full potential of modern hardware. Ruby, a versatile and dynamic programming language, offers robust multithreading capabilities that make concurrent programming a breeze. In this article, we’ll delve into Ruby’s multithreading features, understand their benefits, and explore how they can be effectively utilized for writing efficient and parallel code.
Table of Contents
1. Understanding Multithreading and Concurrency
Multithreading involves the execution of multiple threads within a single process. Each thread represents a separate sequence of instructions that can be scheduled and executed independently. This enables a program to perform multiple tasks concurrently, leveraging the processing power of modern CPUs. Concurrency is the concept of executing multiple tasks in overlapping time periods, allowing for more efficient utilization of system resources.
2. The Need for Multithreading
Modern computers often feature multiple processor cores, which can execute instructions simultaneously. However, many traditional programming languages utilize a single-threaded model, which limits the ability to fully utilize these multicore architectures. Multithreading addresses this limitation by enabling programs to execute multiple tasks concurrently, thereby improving performance and responsiveness.
3. Ruby’s Multithreading Features
Ruby provides a rich set of multithreading features that facilitate the creation and management of threads. Let’s explore some key aspects:
3.1. Thread Creation
Creating threads in Ruby is straightforward. The Thread class provides methods to create and manage threads. Here’s a simple example:
ruby thread1 = Thread.new do # Thread 1 logic here end thread2 = Thread.new do # Thread 2 logic here end # Wait for both threads to finish thread1.join thread2.join
3.2. Thread Synchronization
Synchronization is essential when working with multiple threads to prevent race conditions and ensure data consistency. Ruby offers synchronization mechanisms such as Mutex (mutual exclusion) to protect shared resources. Here’s an example:
ruby counter = 0 counter_mutex = Mutex.new threads = [] 10.times do threads << Thread.new do counter_mutex.synchronize do counter += 1 end end end threads.each(&:join) puts "Counter value: #{counter}"
3.3. Thread Communication
Threads often need to communicate with each other. Ruby provides Queue and ConditionVariable classes for inter-thread communication. Here’s a simplified example using a queue:
ruby work_queue = Queue.new producer_thread = Thread.new do 5.times do |i| work_queue.push("Work #{i}") sleep(1) end end consumer_thread = Thread.new do while (work = work_queue.pop) puts "Processing #{work}" end end producer_thread.join consumer_thread.join
3.4. Thread Safety
Ruby’s Global Interpreter Lock (GIL) limits true parallelism, making it more suitable for I/O-bound tasks rather than CPU-bound tasks. However, Ruby’s multithreading is still valuable for improving I/O-bound operations, such as network requests and file I/O.
4. Leveraging Ruby Multithreading: Use Cases
Let’s explore some common scenarios where Ruby’s multithreading capabilities can be harnessed for efficient and parallel programming:
4.1. Web Scraping
Web scraping often involves making multiple HTTP requests to fetch data from websites. By using multithreading, you can send multiple requests concurrently, significantly speeding up the scraping process.
ruby require 'net/http' urls = ['https://example.com/page1', 'https://example.com/page2', 'https://example.com/page3'] threads = urls.map do |url| Thread.new do response = Net::HTTP.get_response(URI(url)) puts "Fetched #{url}: #{response.code}" end end threads.each(&:join)
4.2. Parallelizing Data Processing
When working with large datasets, you can split the processing into smaller chunks and process them concurrently using threads.
ruby data = (1..1000).to_a chunk_size = 100 threads = data.each_slice(chunk_size).map do |chunk| Thread.new do chunk.each do |item| # Process the item end end end threads.each(&:join)
4.3. Asynchronous Tasks
Ruby’s multithreading can be used to perform asynchronous tasks, such as sending emails in the background while the main application continues to execute.
ruby require 'mail' Mail.defaults do delivery_method :smtp, address: 'smtp.example.com', port: 587 end threads = [] 10.times do threads << Thread.new do # Compose and send an email Mail.deliver do to 'recipient@example.com' subject 'Hello from Ruby Multithreading' body 'This is a multithreaded email!' end end end threads.each(&:join)
5. Best Practices and Considerations
While Ruby’s multithreading capabilities are powerful, there are some best practices and considerations to keep in mind:
- Choose the Right Use Cases: Multithreading is most beneficial for I/O-bound tasks. For CPU-bound tasks, other solutions like parallel processing libraries may be more suitable.
- Avoid Shared Mutable State: Minimize the use of shared mutable state between threads to avoid race conditions. When necessary, use synchronization mechanisms like mutexes.
- Thread Pooling: Creating too many threads can lead to overhead. Consider using thread pooling to limit the number of active threads.
- Error Handling: Ensure proper error handling and graceful termination of threads to prevent resource leaks.
- Performance Testing: Thoroughly test your multithreaded code to identify bottlenecks and ensure optimal performance.
Conclusion
Ruby’s multithreading capabilities provide a powerful tool for achieving concurrency and maximizing the efficiency of your programs. By understanding the fundamentals of multithreading and following best practices, you can harness the full potential of modern hardware and create responsive, high-performance applications. Whether you’re scraping the web, processing data, or performing asynchronous tasks, Ruby’s multithreading features make concurrent programming remarkably accessible and effective. So, why not give it a try and unlock the world of parallelism in your Ruby projects?
In this article, we’ve explored the basics of multithreading in Ruby, covering thread creation, synchronization, communication, and safety. We’ve also looked at practical use cases and considerations for utilizing multithreading effectively. Armed with this knowledge, you’re well-equipped to embark on your journey of writing efficient and concurrent Ruby applications. Happy coding!
Table of Contents
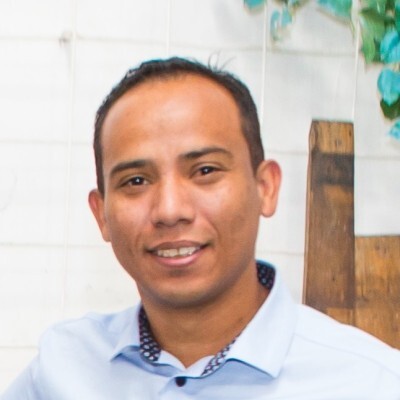
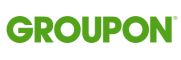