Ruby for Music Composition: Generating Musical Patterns and Scores
Music, a universal language that transcends borders and cultures, has been a source of inspiration and creativity for centuries. While traditional music composition involves pen, paper, and instruments, the digital age has opened up new horizons with the integration of programming languages. Ruby, a dynamic and versatile language, is not just for web development and automation; it can also be a powerful tool for music composition. In this blog, we will explore how Ruby can be used to generate musical patterns and scores, opening up a world of possibilities for musicians and programmers alike.
Table of Contents
1. Why Ruby for Music Composition?
Before we dive into the technical aspects of using Ruby for music composition, it’s essential to understand why Ruby is a great choice for this purpose.
1.1. Expressive and Readable Syntax
Ruby is known for its elegant and human-readable syntax. This makes it an excellent choice for writing music composition code, as it allows musicians and programmers to collaborate effectively. The code becomes a form of sheet music that is easy to understand and modify.
1.2. Versatile Libraries
Ruby boasts a rich ecosystem of libraries and gems that can simplify various tasks related to music composition. Whether you need to work with MIDI files, audio synthesis, or music theory, there are Ruby gems available to streamline your work.
1.3. Interactivity and Experimentation
One of the most exciting aspects of using Ruby for music composition is the ability to create interactive and experimental compositions. With Ruby, you can easily build interfaces that allow real-time control over the music, enabling unique and dynamic performances.
1.4. Integration with Other Tools
Ruby can be seamlessly integrated with other tools and technologies commonly used in music production, such as digital audio workstations (DAWs), allowing you to combine the power of code with traditional music production techniques.
Now that we’ve established why Ruby is a compelling choice for music composition let’s explore how to get started.
2. Setting Up Your Ruby Environment
Before you can begin generating musical patterns and scores with Ruby, you need to set up your development environment. Follow these steps to get started:
2.1. Install Ruby
If you don’t have Ruby installed on your system, you can download and install it from the official Ruby website (https://www.ruby-lang.org/). Make sure you have a recent version of Ruby installed, as it may come with important updates and improvements for your music composition projects.
2.2. Install RubyGems
RubyGems is Ruby’s package manager, and it allows you to easily install and manage Ruby libraries and gems. Most music-related libraries and tools are available as Ruby gems. To install RubyGems, follow the instructions on the official RubyGems website (https://rubygems.org/pages/download).
2.3. Choose a Text Editor or IDE
Select a text editor or integrated development environment (IDE) that you are comfortable with. Popular choices for Ruby development include Visual Studio Code, Sublime Text, and RubyMine. Ensure that your chosen editor has support for Ruby syntax highlighting and code execution.
2.4. Install Music-related Gems
Depending on your specific music composition needs, you may want to install Ruby gems that provide functionality for working with music. Some useful gems include:
- MIDIator: A gem for working with MIDI files and devices.
- SuperCollider-Tools: Tools for integrating with the SuperCollider audio synthesis engine.
- Sonic Pi: A live coding environment for creating music with Ruby.
You can install these gems using the gem install command, followed by the gem’s name.
3. Generating Musical Patterns with Ruby
Now that you have your Ruby environment set up, let’s dive into the exciting world of generating musical patterns with Ruby. We’ll start with a simple example of creating a basic drum pattern using Ruby.
Example 1: Creating a Drum Pattern
ruby require 'midiator' # Create a new MIDIator instance midi = MIDIator::Interface.new # Define the MIDI output port (use '0' for the default port) midi.use(:dls_synth) # Define the drum pattern drum_pattern = [ [:drum, 36, 100, 0.5], # Bass drum [:drum, 38, 100, 0.5], # Snare drum [:drum, 42, 100, 0.5], # Closed hi-hat [:drum, 36, 100, 0.5] # Bass drum ] # Play the drum pattern drum_pattern.each do |note| midi.play(*note) sleep(note[3]) end # Close the MIDI connection midi.close
In this example, we use the MIDIator gem to create a basic drum pattern. We define the MIDI output port, specify the drum pattern as an array of notes, and then play each note with the specified duration. This simple script generates a drum pattern that you can expand upon and modify to create more complex rhythms.
4. Creating Musical Scores with Ruby
While generating musical patterns is fun, you may also want to create full musical scores using Ruby. To achieve this, you can use gems like rvg (Ruby Vector Graphics) and prawn to generate sheet music in various formats.
Example 2: Generating Sheet Music with rvg and prawn
ruby require 'rvg/rvg' require 'prawn' # Define the musical score score = RVG::DPI.new(300, 200).viewbox(0, 0, 300, 200) do |canvas| canvas.background_fill = 'white' # Define a text label canvas.text(150, 100) do |text| text.tspan("My Music Score").styles(:text_anchor => 'middle', :font_size => 20, :font_weight => 'bold') end end # Generate the PDF score pdf_file = 'music_score.pdf' score.draw.write(pdf_file) # Convert the PDF to other formats if needed (e.g., PNG, SVG) Prawn::Document.generate('music_score.png', :page_size => 'A4') do image pdf_file, :at => [0, 800], :fit => [500, 500] end
In this example, we use rvg to create a musical score canvas and prawn to generate a PDF from the canvas. You can customize the score by adding musical notes, symbols, and text labels to create a complete musical composition.
5. Music Theory and Ruby
Understanding music theory is crucial for creating meaningful compositions. Fortunately, Ruby can help you work with music theory concepts and principles.
Example 3: Music Theory with Ruby
ruby require 'music-theory' # Define a chord progression in the key of C major progression = MusicTheory::ChordProgression.new('C major') # Generate a chord progression chords = progression.generate(length: 4) # Print the chord progression puts "Chord Progression in C major:" chords.each_with_index do |chord, index| puts "Bar #{index + 1}: #{chord}" end
In this example, we use the music-theory gem to generate a chord progression in the key of C major. You can explore more advanced music theory concepts and apply them to your compositions with Ruby.
6. Real-time Music Generation with Ruby
One of the most exciting aspects of using Ruby for music composition is the ability to create real-time and interactive music. Tools like Sonic Pi provide a live coding environment where you can experiment with code and instantly hear the results.
Example 4: Real-time Music Generation with Sonic Pi
ruby live_loop :drums do sample :drum_bass_hard sleep 1 sample :drum_snare_hard sleep 1 end live_loop :melody do play scale(:C4, :major).choose sleep 0.5 end
In this Sonic Pi example, we create two live loops—one for drums and another for melody. You can modify the code in real-time, adding or changing notes and rhythms, to create dynamic and interactive music performances.
7. Integrating with Audio Synthesis Engines
For more advanced music composition and sound design, you can integrate Ruby with audio synthesis engines like SuperCollider. SuperCollider is a powerful platform for real-time audio synthesis and algorithmic composition, and it can be controlled from Ruby.
Example 5: Integrating Ruby with SuperCollider
ruby require 'supercollider' # Connect to the SuperCollider server s = SuperCollider::Server.new # Create a synthesizer synth = s.synth :saw, freq: 440, amp: 0.5 # Sleep to allow time for sound to play sleep 2 # Release the synthesizer synth.release # Quit SuperCollider s.quit
In this example, we use the supercollider gem to connect to a SuperCollider server, create a synthesizer, play a note, and then release the synthesizer. This demonstrates how Ruby can be used to control complex audio synthesis processes.
Conclusion
Ruby’s expressive syntax, versatile libraries, and ease of use make it a compelling choice for music composition. Whether you’re generating musical patterns, creating complete musical scores, exploring music theory, or performing real-time music, Ruby has the tools and gems to support your creative journey. So, embrace the fusion of code and music, and let Ruby be your musical companion in the world of composition and sound exploration. Whether you’re a musician or a programmer, Ruby has something harmonious to offer. Start composing your musical masterpiece today!
Table of Contents
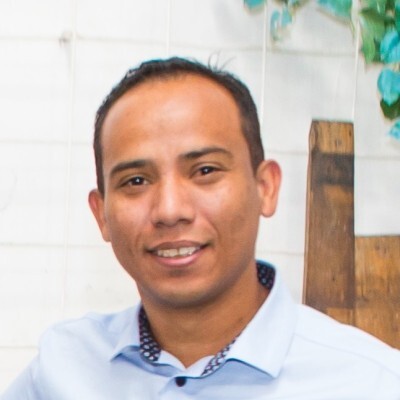
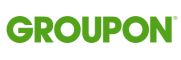