What is the ‘Mutex’ class in Ruby?
In Ruby, the `Mutex` class, short for “mutual exclusion,” is a fundamental synchronization mechanism used to manage access to shared resources in multi-threaded programs. It helps prevent race conditions, which can occur when multiple threads attempt to access or modify shared data simultaneously, leading to unpredictable and erroneous behavior. Here’s an explanation of the `Mutex` class and its purpose:
- Mutual Exclusion:
The primary purpose of a `Mutex` is to ensure mutual exclusion, meaning that only one thread can hold the lock (or “own” the mutex) at a time. When a thread acquires a `Mutex`, it effectively locks it, preventing other threads from entering the critical section of code that’s protected by the mutex.
- Critical Sections:
Mutexes are often used to protect critical sections of code where shared resources, such as variables or data structures, are accessed or modified. By locking the mutex before entering the critical section and releasing it when leaving, threads take turns executing this code, ensuring that only one thread accesses the shared resource at any given time.
- Preventing Race Conditions:
Race conditions occur when multiple threads access shared resources concurrently without synchronization. Mutexes prevent such conditions by providing a way for threads to coordinate and take turns accessing the shared data, avoiding data corruption and ensuring consistent program behavior.
- Mutex Methods:
Ruby’s `Mutex` class provides essential methods like `lock`, `unlock`, and `synchronize` for working with mutexes. The `lock` method is used to acquire the mutex, while `unlock` releases it. The `synchronize` method is a convenient way to ensure that a block of code is executed while holding the mutex, automatically releasing it afterward.
Here’s an example of using a `Mutex` to protect a shared counter in Ruby:
```ruby require 'thread' counter = 0 mutex = Mutex.new threads = [] 10.times do threads << Thread.new do mutex.lock counter += 1 mutex.unlock end end threads.each(&:join) puts "Final counter value: #{counter}" ```
In this example, the `Mutex` ensures that the counter is updated safely by preventing concurrent access. Without the mutex, the final counter value could be inconsistent and incorrect due to race conditions.
The `Mutex` class in Ruby plays a crucial role in managing concurrency and preventing race conditions in multi-threaded programs by allowing threads to safely coordinate access to shared resources. It’s an essential tool for writing thread-safe and reliable code in Ruby.
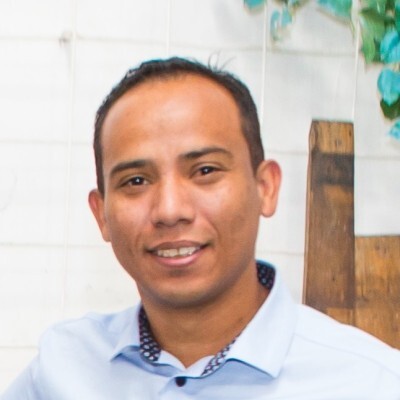
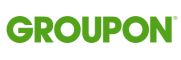