Ruby for Natural Language Understanding: Parsing and Interpreting Text
In the world of programming languages, Ruby stands out as a versatile and elegant choice. It’s not just for web development or scripting; Ruby is also a powerful tool for Natural Language Understanding (NLU). Whether you’re building chatbots, sentiment analysis tools, or any application that deals with human language, Ruby can be your secret weapon. In this blog post, we will explore how to parse and interpret text using Ruby, covering key concepts, libraries, and practical code examples.
Table of Contents
1. Why Ruby for Natural Language Understanding?
Before we dive into the details, let’s address the “why” behind choosing Ruby for Natural Language Understanding. Ruby is known for its simplicity and readability, which makes it an excellent choice for working with text data. Here are a few reasons why Ruby shines in this context:
1.1. Clean and Readable Syntax
Ruby’s syntax is clean and intuitive, making it easy to write and understand code. When dealing with text, clarity in your code is crucial, and Ruby delivers on this front.
1.2. Abundance of Libraries
Ruby has a vibrant ecosystem of libraries and gems that can be leveraged for NLU tasks. From tokenization to sentiment analysis, you’ll find a gem for almost every text processing need.
1.3. Community and Documentation
The Ruby community is known for its helpfulness and documentation. If you encounter any challenges while working on your NLU project, you’re likely to find solutions and guidance from the Ruby community.
Now that we understand why Ruby is a great choice, let’s delve into the core concepts and tools for parsing and interpreting text.
2. Text Tokenization
Tokenization is the process of splitting text into individual words, phrases, or tokens. It’s a fundamental step in text processing, as it allows you to work with the smallest meaningful units of text. Ruby provides several ways to tokenize text, and one of the most commonly used methods involves regular expressions.
2.1. Using Regular Expressions
Ruby’s regular expression support makes it easy to tokenize text based on patterns. Here’s a simple example of how you can tokenize a sentence into words using regular expressions:
ruby text = "Ruby is a powerful language for NLU." tokens = text.split(/\s+/) puts tokens
In this example, we split the text variable into tokens based on one or more whitespace characters. The split method divides the text wherever it encounters a space, resulting in an array of tokens.
2.2. Using the NLP Gem
If you prefer a more specialized approach, you can use the NLP gem, which offers more advanced tokenization capabilities. First, you need to install the gem:
ruby gem install nlp
Now, let’s tokenize a sentence using the NLP gem:
ruby require 'nlp' nlp = NLP.new text = "Ruby is a powerful language for NLU." tokens = nlp.tokenize(text) puts tokens
The NLP gem provides more sophisticated tokenization, handling punctuation, contractions, and other language-specific nuances.
3. Part-of-Speech Tagging
Part-of-speech (POS) tagging involves assigning grammatical categories (e.g., noun, verb, adjective) to each token in a sentence. This is a crucial step in understanding the structure and meaning of a text. Ruby offers libraries like the stanford-core-nlp gem for performing POS tagging.
3.1. Using the stanford-core-nlp Gem
To use the stanford-core-nlp gem, you’ll need to set up a Stanford CoreNLP server. Once it’s running, you can perform POS tagging as follows:
ruby require 'stanford-core-nlp' # Start the Stanford CoreNLP server StanfordCoreNLP.use :english # Create a client client = StanfordCoreNLP.load # Text for POS tagging text = "Ruby is a powerful language for NLU." # Perform POS tagging result = client.annotate(text) sentences = result.get(:sentences) sentences.each do |sentence| tokens = sentence.get(:tokens) tokens.each do |token| word = token.get(:word) pos = token.get(:pos) puts "#{word}: #{pos}" end end
In this example, we use Stanford CoreNLP to annotate the text and extract POS tags for each token. This information is invaluable for understanding the grammatical structure of sentences.
4. Named Entity Recognition
Named Entity Recognition (NER) is the process of identifying and classifying entities in text, such as names of people, organizations, locations, and more. Ruby offers libraries like the ner gem for NER tasks.
4.1. Using the ner Gem
To use the ner gem for NER, you first need to install it:
ruby gem install ner
Now, let’s perform NER on a sample text:
ruby require 'ner' text = "Apple Inc. is headquartered in Cupertino, California. Tim Cook is the CEO." ner = Ner.new entities = ner.recognize(text) entities.each do |entity| puts "#{entity[:entity]}: #{entity[:value]}" end
In this code, we use the ner gem to recognize named entities in the text. The gem provides information about the type of entity and its value.
5. Sentiment Analysis
Sentiment analysis is a crucial task in Natural Language Understanding. It involves determining the sentiment or emotional tone expressed in a piece of text, whether it’s positive, negative, or neutral. Ruby has libraries like the sentimental gem for performing sentiment analysis.
5.1. Using the sentimental Gem
To use the sentimental gem, you first need to install it:
ruby gem install sentimental
Now, let’s analyze the sentiment of a sentence:
ruby require 'sentimental' text = "I love Ruby! It's an amazing language." analyzer = Sentimental.new analyzer.load_defaults sentiment = analyzer.sentiment(text) puts "Sentiment: #{sentiment}"
In this code, we use the sentimental gem to analyze the sentiment of the text. The gem provides a sentiment score, indicating the overall sentiment expressed in the text.
6. Building a Basic Chatbot
Now that we’ve explored some key NLU tasks in Ruby, let’s put our knowledge to use by building a basic chatbot. Our chatbot will respond to user input with predefined responses.
6.1. Setting Up the Chatbot
ruby class SimpleChatbot RESPONSES = { "hello" => "Hello! How can I assist you?", "how are you" => "I'm just a computer program, but thanks for asking!", "bye" => "Goodbye! Have a great day.", } def initialize @analyzer = Sentimental.new @analyzer.load_defaults end def respond(input) input = input.downcase sentiment = @analyzer.sentiment(input) if sentiment == :positive response = "That's great to hear! #{random_response}" elsif sentiment == :negative response = "I'm sorry to hear that. #{random_response}" else response = random_response end response end private def random_response RESPONSES.values.sample end end # Usage example chatbot = SimpleChatbot.new loop do print "> " input = gets.chomp break if input.downcase == "exit" response = chatbot.respond(input) puts response end
In this code, we define a SimpleChatbot class that responds to user input based on sentiment analysis. It uses the sentimental gem to determine the sentiment of the user’s input and generates an appropriate response.
Conclusion
Ruby is a versatile and elegant language that can be a powerful ally in Natural Language Understanding tasks. From tokenization to sentiment analysis and building chatbots, Ruby provides the tools and libraries you need to work with text data effectively. So, the next time you embark on an NLU project, consider Ruby as your language of choice. Its simplicity and readability will make your journey into the world of NLU all the more enjoyable and productive.
In this blog post, we’ve covered some essential NLU tasks in Ruby and provided code samples to help you get started. Whether you’re a seasoned Ruby developer or just getting started, exploring NLU in Ruby is a rewarding endeavor. So, roll up your sleeves, experiment with text data, and unlock the possibilities of Natural Language Understanding with Ruby. Happy coding!
Table of Contents
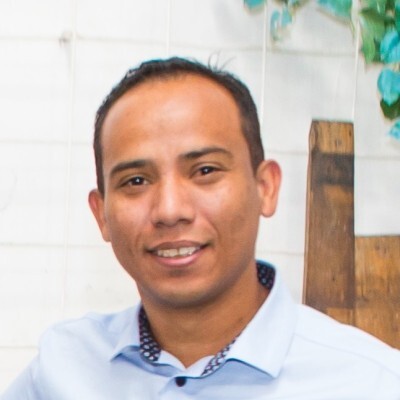
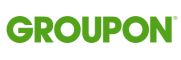