Understanding Ruby’s Object-Oriented Programming Paradigm
Object-oriented programming (OOP) is a popular programming paradigm that many programming languages, including Ruby, incorporate into their design. This blog post delves into how OOP is realized in Ruby, a critical knowledge area for those looking to hire Ruby developers. It guides you through Ruby’s key OOP concepts and elements, providing insights into what to look for when assessing the skills of potential Ruby developer candidates.
Ruby: A True Object-Oriented Language:
Ruby, a dynamic, open-source programming language, has been loved by developers around the globe for its simplicity and elegance. Created by Yukihiro Matsumoto, the language is designed with a focus on simplicity and productivity, prioritizing the happiness of programmers. But what truly sets Ruby apart is its adherence to the object-oriented programming paradigm.
In Ruby, everything is an object. Each value or entity —be it a string, a number, an array, or a hash— is considered an object. Each of these objects has a class (its ‘type’), methods (things it can do), and attributes (its properties or state).
Classes and Objects:
The fundamental building block of Ruby’s OOP model is the class. A class defines a blueprint for objects, specifying their behavior (methods) and state (attributes). Objects are instances of a class, embodying the defined behavior and state. Here’s an example of a Ruby class:
```ruby class Car def initialize(model, color) @model = model @color = color end def describe "This is a #{@color} #{@model}." end end car = Car.new('Tesla Model 3', 'red') puts car.describe # Outputs: "This is a red Tesla Model 3."
In this example, `Car` is a class with attributes `@model` and `@color`, and a method `describe`. We create an instance of `Car` with the `.new` method, passing in specific values for the attributes. The `describe` method then gives us a way to interact with the object’s state.
Encapsulation:
Ruby enforces the OOP concept of encapsulation, providing a way to hide an object’s internal state and functionality from the outside world. This leads to a robust codebase where each object manages its own state.
In the previous example, `@model` and `@color` are instance variables, only accessible within the object. Outside access is usually provided through methods, often called getters and setters. Ruby provides a handy way to generate these using `attr_accessor`, `attr_reader`, and `attr_writer`:
```ruby class Car attr_accessor :model, :color def initialize(model, color) @model = model @color = color end # ... end
Inheritance:
Inheritance is another pillar of OOP that Ruby implements. It allows classes to inherit methods and attributes from another class. The class being inherited from is called the superclass, while the class doing the inheriting is called the subclass. This relationship leads to a hierarchy that promotes code reuse and organization.
```ruby class ElectricCar < Car def initialize(model, color, battery_capacity) super(model, color) @battery_capacity = battery_capacity end # ... end
In this example, `ElectricCar` is a subclass of `Car`, inheriting its methods and attributes, but also adding a new attribute, `@battery_capacity`.
Polymorphism:
Polymorphism is the ability to present the same interface for differing underlying forms. In Ruby, this is commonly achieved through duck typing—a term coined in reference to the phrase, “If it walks like a duck and it quacks like a duck, then it must be a duck.”
```ruby class Pet def make_sound # ... end end class Cat < Pet def make_sound "Meow!" end end class Dog < Pet def make_sound "Woof!" end end pets = [Cat.new, Dog.new] pets.each { |pet| puts pet.make_sound } # Outputs: "Meow!", "Woof!"
Each pet has a `make_sound` method, but the implementation varies.
Conclusion:
Ruby’s object-oriented nature provides a robust and flexible framework for designing software, a key reason why businesses choose to hire Ruby developers. Through classes and objects, encapsulation, inheritance, and polymorphism, Ruby equips developers with powerful tools to structure their programs, leading to more maintainable, readable, and reliable code.
Understanding these concepts is crucial, not only for mastering Ruby but also for other OOP languages. They form the backbone of modern programming practices, setting the groundwork for advanced topics and patterns in software development. Hence, when you’re looking to hire Ruby developers, proficiency in these concepts can significantly elevate their coding skills.
The beauty of Ruby’s object-oriented design lies not just in its functionality but also in its simplicity. With Ruby, the journey to becoming an OOP expert — or for businesses, the journey to successfully hire Ruby developers who are OOP experts — is clear, enjoyable, and most importantly, achievable. Happy coding!
Table of Contents
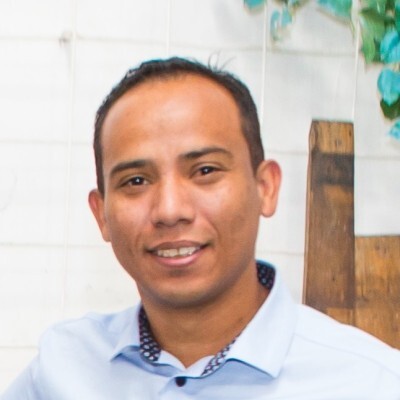
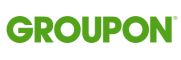