How to use the ‘open-uri’ library in Ruby?
The `open-uri` library in Ruby is a convenient and versatile module that allows you to easily open and read data from URLs, files, and other network resources. It’s a part of the Ruby standard library, which means you don’t need to install any additional gems to use it. `open-uri` simplifies tasks such as downloading files from the internet, fetching web content, or reading remote resources.
Here’s how you can use the `open-uri` library in Ruby:
- Requiring the Library:
First, you need to require the `open-uri` library in your Ruby script or program using the following statement:
```ruby require 'open-uri' ```
- Opening URLs:
You can open a URL by passing it to the `open` method, like this:
```ruby url = 'https://example.com' response = open(url) ```
This will create an `IO` object that you can use to read the content from the URL.
- Reading Content:
You can read the content of the URL using the `read` method:
```ruby html_content = response.read ```
This will store the content of the URL in the `html_content` variable as a string.
- Handling Errors:
It’s a good practice to handle exceptions when working with remote resources. The `open-uri` library may raise exceptions such as `OpenURI::HTTPError` or `OpenURI::HTTPRedirect` if there are issues with the URL or the request. You can use a `begin`…`rescue` block to catch and handle these exceptions.
```ruby begin response = open(url) html_content = response.read rescue OpenURI::HTTPError => e puts "Error: #{e.message}" end ```
- Opening Local Files:
`open-uri` is not limited to remote resources; you can also use it to open and read local files by providing a file path instead of a URL.
```ruby local_file_path = 'path/to/local/file.txt' file_content = open(local_file_path).read ```
- Additional Options:
`open-uri` provides some additional options, such as setting HTTP headers, controlling redirects, or specifying a proxy. You can pass these options as a hash to the `open` method.
The `open-uri` library is a handy tool for working with web content and files, especially when you need a simple way to fetch data from URLs. However, keep in mind that it may not be suitable for advanced web scraping or when dealing with complex HTTP requests. In such cases, you may want to explore more powerful gems like `HTTParty` or `Faraday` for finer control over your HTTP interactions.
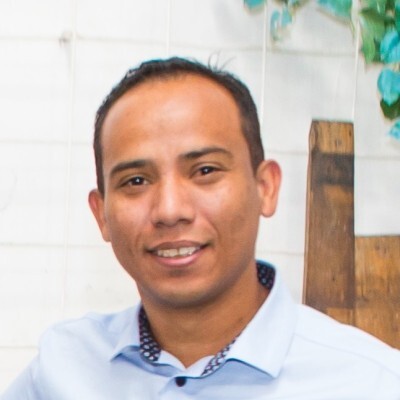
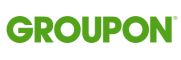