Optimizing Database Performance in Ruby: Techniques and Best Practices
In the world of modern software development, optimizing database performance is paramount to ensure a seamless user experience and efficient application behavior. Ruby, a popular programming language known for its elegant syntax and developer-friendly environment, powers countless web applications. When working with Ruby applications that involve database interactions, understanding how to optimize database performance becomes crucial. In this blog post, we will delve into techniques and best practices to help you achieve top-notch database performance in your Ruby applications.
Table of Contents
1. Why Database Performance Matters
Database performance plays a vital role in determining the responsiveness and scalability of your application. A slow-performing database can lead to sluggish application behavior, increased latency, and frustrated users. On the other hand, a well-optimized database can provide quicker response times, better scalability, and improved overall user satisfaction.
2. Choosing the Right Database
The foundation of optimizing database performance starts with choosing the right database management system (DBMS) for your Ruby application. Different databases have varying strengths and weaknesses that align with specific application requirements. While Ruby works seamlessly with a variety of databases, such as PostgreSQL, MySQL, SQLite, and MongoDB, selecting the appropriate database for your application’s workload is essential.
2.1. Evaluating Application Requirements
Before deciding on a database, assess your application’s requirements. Consider factors like data volume, read and write patterns, complexity of queries, and data consistency. For example, if your application involves complex relationships and requires support for advanced querying, PostgreSQL might be a suitable choice. On the other hand, if your application needs fast read and write operations with minimal configuration overhead, SQLite could be a viable option.
2.2. Scaling Considerations
Think about the potential for your application’s growth. Will your application need to handle increasing amounts of data and traffic over time? Choose a database that allows for easy scalability. Some databases, like PostgreSQL and MySQL, offer features like replication, sharding, and partitioning that can help distribute the load and ensure consistent performance as your application expands.
3. Efficient Data Modeling
Proper data modeling is at the core of database performance optimization. Well-designed database schemas can significantly impact query efficiency and data retrieval times.
3.1. Normalization vs. Denormalization
When designing your database schema, strike a balance between normalization and denormalization. Normalization involves breaking down data into smaller, related tables to minimize redundancy and improve data integrity. However, normalized schemas might require more complex joins, leading to slower query performance. Denormalization, on the other hand, involves combining data into fewer tables, which can enhance query speed but might sacrifice some level of data integrity. Assess your application’s requirements to determine the most suitable approach.
3.2. Indexing Strategies
Indexes are essential for efficient data retrieval, especially when dealing with large datasets. In Ruby applications, use database-specific indexing mechanisms to enhance query performance. For instance, PostgreSQL supports various index types, including B-tree, hash, and GIN (Generalized Inverted Index), each catering to different query scenarios. Regularly analyze query patterns and create indexes on columns frequently used in WHERE clauses or JOIN conditions to speed up query execution.
ruby # PostgreSQL example: Creating an index # Assuming 'users' table with 'email' column add_index :users, :email
3.3. Utilizing Caching
Caching frequently accessed data can drastically reduce the need for repeated database queries. Implement caching strategies using tools like Redis or Memcached. When a query result is cached, subsequent requests for the same data can be served directly from the cache, significantly improving response times.
ruby # Caching query result using Rails cache user = Rails.cache.fetch("user_#{user_id}") do User.find(user_id) end
4. Efficient Querying with ActiveRecord
ActiveRecord, the object-relational mapping (ORM) library in Ruby on Rails, simplifies database interactions. However, writing efficient queries using ActiveRecord is essential for optimal database performance.
4.1. N+1 Query Problem
The N+1 query problem occurs when a query retrieves a collection of records and subsequently generates an additional query for each record to fetch associated data. This can lead to an excessive number of database queries, resulting in performance bottlenecks. Use ActiveRecord’s eager loading feature to mitigate this issue.
ruby # N+1 query example without eager loading # Results in 1 query to fetch posts and N queries to fetch comments for each post posts = Post.all posts.each { |post| puts post.comments } # N+1 query solution with eager loading # Results in 2 queries: 1 for posts and 1 for comments posts = Post.includes(:comments)
4.2. Limiting and Pagination
When fetching large datasets, limit the number of retrieved records using the LIMIT clause. Additionally, implement pagination to retrieve data in smaller, manageable chunks. This approach improves query performance and prevents excessive memory consumption.
ruby # Limiting and pagination with ActiveRecord page_number = 1 page_size = 10 posts = Post.limit(page_size).offset((page_number - 1) * page_size)
ruby # Explaining query execution plan in PostgreSQL EXPLAIN ANALYZE SELECT * FROM users WHERE age > 25;
Conclusion
Optimizing database performance is a continuous effort that involves making informed decisions from the early stages of application development. By choosing the right database, efficiently designing data models, crafting optimized queries, and employing monitoring tools, you can ensure that your Ruby application delivers exceptional performance and a delightful user experience. Keep in mind that each application is unique, so tailor these techniques to match your specific requirements and constantly iterate on your optimization strategies to stay ahead in the ever-evolving landscape of software development.
Table of Contents
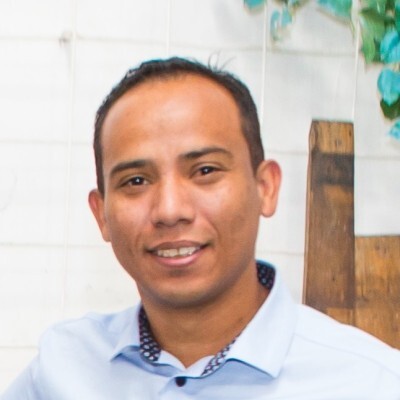
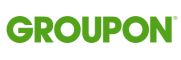