How to profile Ruby code for performance optimization?
Profiling Ruby code is a crucial step in optimizing the performance of your applications. It involves analyzing your code to identify bottlenecks and areas where improvements can be made. Ruby provides various tools and techniques for profiling code to ensure it runs efficiently.
- Benchmarking Tools: Ruby offers built-in benchmarking tools like the `Benchmark` module, which allows you to measure the execution time of specific code blocks. By comparing different implementations, you can identify which parts of your code need optimization.
```ruby require 'benchmark' result = Benchmark.measure do # Code to be measured end puts result ```
- Profiling Gems: There are third-party gems like `ruby-prof` and `stackprof` that provide more detailed insights into your code’s performance. These gems can generate comprehensive reports, including method-level performance data and call graphs, helping you pinpoint performance bottlenecks.
```ruby require 'ruby-prof' RubyProf.start # Code to be profiled result = RubyProf.stop printer = RubyProf::FlatPrinter.new(result) printer.print(STDOUT) ```
- Memory Profiling: Profiling memory usage is crucial, especially for long-running applications. Tools like `memory_profiler` can help you identify memory leaks and optimize memory consumption.
```ruby require 'memory_profiler' report = MemoryProfiler.report do # Code to be profiled for memory end report.pretty_print ```
- Application Monitoring: Consider using application monitoring and profiling tools like New Relic, Scout, or AppSignal for a broader view of your application’s performance. These tools can provide real-time insights into production code and help you identify performance issues as they occur.
When profiling your Ruby code, it’s essential to focus on the critical sections of your application that have the most significant impact on performance. Once bottlenecks are identified, you can employ various optimization techniques such as algorithmic improvements, caching, and database query optimization to enhance your code’s efficiency.
Profiling Ruby code is an essential step in optimizing performance. Ruby offers built-in and third-party tools to help you identify bottlenecks, measure execution times, and pinpoint memory usage issues. Profiling allows you to make informed decisions and implement optimizations that can lead to faster and more efficient Ruby applications.
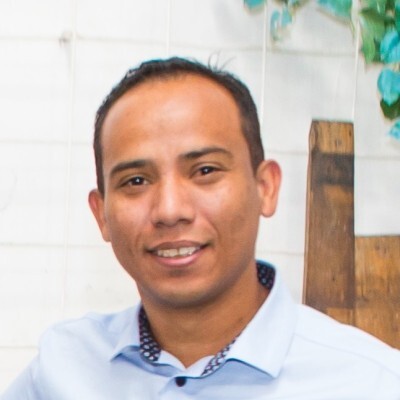
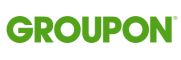