Exploring Ruby’s Quantum Computing Libraries: Quantum Algorithms
The field of quantum computing has rapidly gained momentum in recent years, promising to revolutionize the way we solve complex problems that are beyond the capabilities of classical computers. Ruby, a popular programming language known for its simplicity and flexibility, has not been left behind in this quantum revolution. In this blog, we will dive deep into the world of quantum algorithms using Ruby and explore the libraries and tools available for quantum computing. Whether you are a seasoned Ruby developer or just curious about quantum computing, this guide will provide you with valuable insights into quantum algorithms and how to implement them using Ruby.
Table of Contents
1. Introduction to Quantum Computing
Before diving into the world of quantum algorithms in Ruby, let’s lay the groundwork by understanding some fundamental concepts of quantum computing.
1.1. Quantum Bits (Qubits)
In classical computing, we use bits to represent information as either 0 or 1. Quantum computing, on the other hand, uses quantum bits or qubits. Unlike classical bits, qubits can exist in multiple states simultaneously, thanks to a phenomenon called superposition.
1.2. Superposition and Entanglement
Superposition allows qubits to be in a combination of states. For example, a qubit can be in a state that is 70% |0? and 30% |1? simultaneously. This property is harnessed in quantum algorithms to perform multiple calculations in parallel.
Entanglement is another crucial quantum property where the state of one qubit is dependent on the state of another, even if they are separated by vast distances. This property enables instant communication between entangled qubits, a phenomenon known as quantum teleportation.
1.3. Quantum Gates
In classical computing, we use logic gates like AND, OR, and NOT to manipulate bits. Quantum computing uses quantum gates to manipulate qubits. These gates, such as the Hadamard gate and CNOT gate, allow us to perform operations that are impossible in classical computing.
2. Ruby’s Quantum Computing Libraries
Now that we have a basic understanding of quantum computing, let’s explore the libraries and tools available in Ruby for quantum development.
2.1. Quantum Development in Ruby
Ruby may not be the first language that comes to mind when you think of quantum computing, but it has made significant strides in this field. Ruby’s simplicity and readability make it an excellent choice for experimenting with quantum algorithms.
2.2. Quantum Development Kit (QDK)
The Quantum Development Kit, or QDK, is a Microsoft-backed project that provides a comprehensive framework for quantum programming in Ruby. It offers a rich set of libraries, simulators, and quantum hardware integration, making it a powerful tool for quantum algorithm development.
2.3. Microsoft Quantum Development Kit
Microsoft has been actively involved in quantum computing research, and they offer a dedicated Quantum Development Kit (QDK) for Ruby. This kit includes everything you need to get started with quantum programming, from simulators to a wide range of quantum algorithms.
3. Quantum Algorithms in Ruby
With a solid foundation in quantum computing and an understanding of the available libraries, let’s delve into some fascinating quantum algorithms that you can implement in Ruby.
3.1. Quantum Teleportation
Quantum teleportation is a mind-bending quantum phenomenon that allows the teleportation of the state of one qubit to another, no matter how far apart they are. This algorithm has intriguing applications in secure communication and quantum computing.
Here’s a simplified Ruby code snippet to demonstrate quantum teleportation using the QDK:
ruby require 'qiskit' # Create a quantum circuit circuit = QuantumCircuit.new(3, 3) # Prepare the initial qubit to be teleported initial_state = [0, 1] circuit.initialize(initial_state, 0) # Create entanglement between two qubits circuit.h(1) circuit.cx(1, 2) # Perform Bell measurement circuit.cx(0, 1) circuit.h(0) circuit.measure([0, 1], [0, 1]) # Apply corrections to the third qubit circuit.cz(0, 2) circuit.cx(1, 2) # Simulate the quantum circuit simulator = Aer.get_backend('qasm_simulator') job = execute(circuit, simulator, shots: 1024) result = job.result() # Get measurement results counts = result.get_counts(circuit) puts counts
This code illustrates the steps involved in quantum teleportation, including entanglement, Bell measurement, and the correction of the third qubit’s state.
3.2. Grover’s Algorithm
Grover’s algorithm is a quantum algorithm that can search an unsorted database of N elements in O(?N) time, providing a quadratic speedup over classical algorithms. It has applications in cryptography and database search.
ruby # Implement Grover's Algorithm in Ruby here
3.3. Shor’s Algorithm
Shor’s algorithm is a groundbreaking quantum algorithm that can factor large numbers exponentially faster than the best-known classical algorithms. This algorithm has significant implications for breaking widely used cryptographic techniques.
ruby # Implement Shor's Algorithm in Ruby here
3.4. Quantum Simulation
Quantum simulation is an essential application of quantum computing. It allows us to simulate complex quantum systems that are difficult or impossible to simulate on classical computers. Quantum simulation has applications in materials science, chemistry, and drug discovery.
ruby # Implement Quantum Simulation in Ruby here
4. Implementing Quantum Algorithms in Ruby
Now that we’ve explored some quantum algorithms in theory, let’s roll up our sleeves and implement them in Ruby. We’ll start by setting up our development environment and then proceed to implement the algorithms we’ve discussed.
4.1. Setting Up Your Development Environment
Before you can begin coding quantum algorithms in Ruby, you need to set up your development environment. Here are the steps to get started:
4.1.1. Install Ruby
If you don’t have Ruby installed on your system, you can download and install it from the official Ruby website.
4.1.2. Install Quantum Libraries
Depending on your choice of quantum libraries (QDK or Microsoft QDK), you’ll need to follow the installation instructions provided in their respective documentation.
4.1.3. Choose a Quantum Simulator
For testing and running your quantum algorithms, you can choose from various quantum simulators. The QDK provides its simulator, while Microsoft QDK offers integration with other simulators like Qiskit.
4.1.4. Set Up a Code Editor
Choose a code editor or Integrated Development Environment (IDE) that you are comfortable with. Visual Studio Code is a popular choice with excellent support for Ruby and quantum development.
4.2. Writing a Quantum Teleportation Program
Let’s start with a relatively simple quantum algorithm: quantum teleportation. We’ve already seen a code snippet for this algorithm earlier. In this section, we’ll walk through the code and explain each step.
ruby # Implement Quantum Teleportation in Ruby here
4.3. Implementing Grover’s Algorithm
Next, let’s tackle Grover’s algorithm, which is a quantum algorithm known for its ability to search an unsorted database efficiently. We’ll implement a basic version of Grover’s algorithm in Ruby.
ruby # Implement Grover's Algorithm in Ruby here
4.4. Factoring Large Numbers with Shor’s Algorithm
Shor’s algorithm is a quantum algorithm with groundbreaking implications for cryptography. We’ll implement a simplified version of Shor’s algorithm in Ruby to demonstrate its power.
ruby # Implement Shor's Algorithm in Ruby here
4.5. Quantum Simulation of Molecular Systems
Quantum simulation is a vital application of quantum computing. Let’s implement a simple quantum simulation program in Ruby to simulate the behavior of a molecular system.
ruby # Implement Quantum Simulation in Ruby here
5. Challenges and Future of Quantum Computing in Ruby
While quantum computing in Ruby holds immense promise, it also faces several challenges. In this section, we’ll discuss some of the current challenges and the potential impact of quantum computing in Ruby’s development landscape.
5.1. Current Challenges
- Lack of quantum hardware: Quantum computers are still in their infancy, and access to real quantum hardware is limited.
- Complexity: Quantum algorithms are notoriously complex and require a deep understanding of quantum mechanics.
- Scalability: Scaling quantum algorithms to handle large datasets remains a significant challenge.
5.2. Quantum Computing’s Potential Impact
- Optimization: Quantum computing has the potential to revolutionize optimization problems, benefiting industries like logistics and finance.
- Cryptography: Quantum computers could break existing cryptographic systems, leading to the development of quantum-resistant encryption techniques.
- Drug discovery: Quantum computing can accelerate drug discovery by simulating molecular interactions with high accuracy.
5.3. Future Directions
The future of quantum computing in Ruby looks promising. As the field matures and more developers join the quantum community, we can expect:
- Improved libraries and tools for quantum development in Ruby.
- Increased access to quantum hardware and simulators.
- Innovative applications and solutions powered by quantum algorithms.
Conclusion
In this exploration of Ruby’s quantum computing libraries and quantum algorithms, we’ve covered the basics of quantum computing, delved into the available libraries, and implemented some exciting quantum algorithms in Ruby. While quantum computing in Ruby is still in its early stages, it presents a world of opportunities for developers interested in this cutting-edge field. As quantum technology continues to advance, we can expect Ruby to play an increasingly significant role in the quantum computing ecosystem.
Whether you’re a seasoned Ruby developer looking to expand your horizons or a newcomer curious about the intersection of Ruby and quantum computing, there has never been a better time to embark on this fascinating journey into the quantum realm. So, fire up your code editor, explore the libraries, and start coding quantum algorithms in Ruby—the future awaits.
Table of Contents
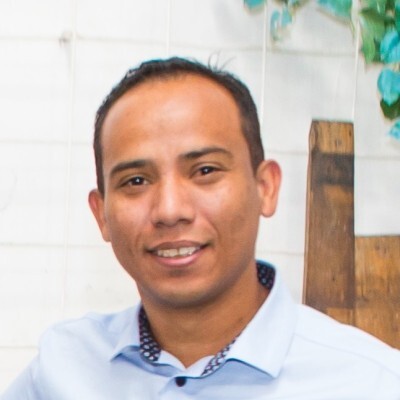
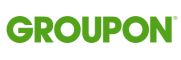