Building Real-Time Applications with Ruby and WebSockets
In today’s fast-paced digital landscape, real-time communication has become an integral part of many applications. Whether it’s live chat, collaborative tools, online gaming, or financial applications that require live updates, developers need reliable and efficient methods to enable real-time interactions. This is where WebSockets come into play. WebSockets provide a full-duplex communication channel over a single TCP connection, allowing bidirectional communication between clients and servers. In this guide, we’ll explore how to leverage the power of WebSockets to build robust real-time applications using the Ruby programming language.
Table of Contents
1. Understanding WebSockets
Before diving into the implementation details, it’s essential to understand what WebSockets are and why they are crucial for real-time applications. Unlike traditional HTTP requests, which follow a request-response pattern, WebSockets enable continuous communication between a client and a server. This persistent connection allows data to be transmitted in both directions without the overhead of repeatedly establishing new connections.
2. The Need for Real-Time Communication
Consider a live chat application where users exchange messages in real time. Using traditional HTTP requests, the client would need to poll the server at regular intervals to check for new messages, leading to unnecessary network traffic and delays. WebSockets eliminate this inefficiency by establishing a long-lived connection that facilitates instant data transmission. This ability to push updates to clients as soon as they are available is a game-changer for applications requiring real-time interactions.
3. Setting Up a Ruby WebSocket Server
Now that we have a grasp of why WebSockets are beneficial, let’s dive into implementing them using the Ruby programming language. For this tutorial, we’ll use the popular ‘websocket-ruby’ gem, which provides a robust WebSocket server implementation.
3.1. Prerequisites
Before getting started, ensure you have Ruby and Bundler installed on your system. You can install the ‘websocket-ruby’ gem by adding it to your project’s Gemfile and running bundle install.
3.2. Implementing the WebSocket Server
Below is a step-by-step guide to creating a basic WebSocket server using the ‘websocket-ruby’ gem.
Step 1: Require Dependencies
First, require the necessary dependencies and set up the WebSocket server.
ruby require 'websocket' require 'websocket/server' require 'socket' # Set up the server to listen on a specific port server = TCPServer.new('0.0.0.0', 3000)
Step 2: Accept WebSocket Connections
Next, accept incoming WebSocket connections and establish a WebSocket server for each client.
ruby sockets = [] loop do client_socket = server.accept socket = WebSocket::Server.new(socket: client_socket) sockets << socket Thread.new do socket.listen sockets.delete(socket) end end
Step 3: Handle Incoming Messages
Within the WebSocket server loop, you can now handle incoming messages from clients.
ruby socket.on(:message) do |message| puts "Received message: #{message}" # Process the message and send a response back to the client socket.send("You said: #{message}") end
4. Enabling Real-Time Communication
With the WebSocket server in place, you can now enable real-time communication between clients and the server. Let’s explore how to create a simple client using HTML, JavaScript, and the ‘websocket’ JavaScript library.
4.1. Setting Up the HTML
Start by creating an HTML file that will host the WebSocket client.
html <!DOCTYPE html> <html> <head> <title>WebSocket Client</title> </head> <body> <input type="text" id="messageInput" placeholder="Enter your message"> <button id="sendButton">Send</button> <div id="output"></div> <script src="websocket.min.js"></script> <script src="client.js"></script> </body> </html>
4.2. Implementing the JavaScript Client
Create a ‘client.js’ file to implement the WebSocket client.
javascript const socket = new WebSocket('ws://localhost:3000'); const messageInput = document.getElementById('messageInput'); const sendButton = document.getElementById('sendButton'); const outputDiv = document.getElementById('output'); socket.onopen = () => { console.log('WebSocket connection opened.'); }; socket.onmessage = (event) => { const message = event.data; outputDiv.innerHTML += `<p>${message}</p>`; }; socket.onclose = () => { console.log('WebSocket connection closed.'); }; sendButton.addEventListener('click', () => { const message = messageInput.value; socket.send(message); messageInput.value = ''; });
5. Use Cases for Real-Time Ruby WebSocket Applications
Now that you have a functional real-time Ruby WebSocket application, let’s explore some common use cases where this technology shines.
5.1. Live Chat Applications
Real-time chat applications, whether for customer support or social interactions, rely heavily on instant message delivery. With WebSockets, messages can be sent and received without delay, creating a seamless chatting experience for users.
5.2. Collaborative Tools
Collaborative tools, such as document editors or project management platforms, benefit from real-time updates. With WebSockets, multiple users can work on the same document or project, seeing changes in real time without the need to constantly refresh.
5.3. Online Gaming
WebSockets are a natural fit for online gaming, where timely updates are critical to gameplay. Players can engage in multiplayer experiences, battling opponents or collaborating with teammates, all in real time.
5.4. Financial Applications
In financial applications, real-time data is essential for tracking stock prices, currency exchange rates, and market trends. WebSockets allow traders and investors to stay updated on market changes without delays.
Conclusion
WebSockets have revolutionized the way we build real-time applications, enabling seamless and instant communication between clients and servers. In this guide, we explored the basics of WebSockets, implemented a Ruby WebSocket server using the ‘websocket-ruby’ gem, and created a JavaScript WebSocket client. We also discussed various use cases where real-time communication is vital, such as live chat, collaborative tools, online gaming, and financial applications. By harnessing the power of WebSockets and Ruby, developers can create dynamic and engaging applications that provide users with real-time experiences like never before. So go ahead and start building your own real-time applications with confidence and innovation!
Table of Contents
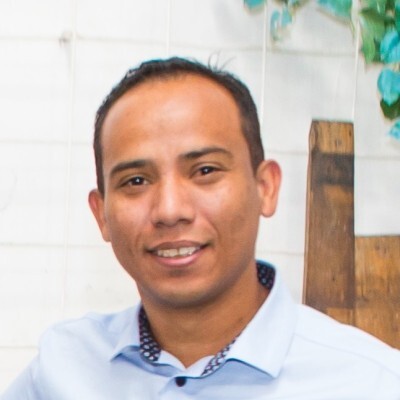
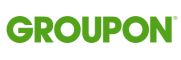