Exploring Ruby’s Reflection API: Introspecting Objects at Runtime
Ruby is renowned for its flexibility and dynamic nature, making it a popular choice among developers for various applications. One of the features that exemplifies this dynamic capability is Ruby’s Reflection API. This powerful tool allows you to examine and manipulate objects during runtime, opening up opportunities for introspection, metaprogramming, and more. In this blog post, we’ll take an in-depth journey into Ruby’s Reflection API, exploring its various facets, use cases, and code samples.
Table of Contents
1. Introduction to Ruby’s Reflection API
1.1. What is Reflection?
Reflection, in the context of programming languages, refers to the ability of a program to examine and manipulate its own structure, behavior, and data during runtime. Ruby’s Reflection API provides mechanisms to introspect classes, modules, methods, and objects while a program is executing. This means you can retrieve information about classes, their methods, instance variables, and even create or modify them dynamically.
1.2. Why is Reflection Important?
Reflection opens the door to powerful metaprogramming techniques, where you can create code that writes code, making your applications more flexible and adaptable. It’s often used in frameworks, libraries, and development tools to automate repetitive tasks, enhance debugging capabilities, and provide a richer development experience.
2. Exploring Reflection Techniques
2.1. Retrieving Class and Module Information
Ruby’s Reflection API offers various methods to retrieve information about classes and modules. The Object class provides methods like #class and #module to access an object’s class and module, respectively. Additionally, the Module class provides methods like #ancestors to retrieve a list of classes and modules in the inheritance hierarchy.
ruby class MyClass end obj = MyClass.new puts obj.class # Output: MyClass puts obj.class.ancestors # Output: [MyClass, Object, Kernel, BasicObject]
2.2. Accessing Method and Instance Variable Details
You can also use Reflection to access method and instance variable details of a class or object. The #methods method returns an array of symbols representing the available methods, while #instance_variables returns an array of instance variable names.
ruby class Person attr_accessor :name, :age def greet puts "Hello, I'm #{@name}!" end end person = Person.new puts person.methods - Object.methods # Output: [:name, :name=, :age, :age=, :greet, ...] puts person.instance_variables # Output: []
3. Introspection: Peering into Object Details
3.1. #inspect and #to_s Methods
The #inspect method is one of the most commonly used Reflection techniques in Ruby. It returns a string representation of an object, providing valuable information about its class, instance variables, and their values. The #to_s method, on the other hand, returns a user-friendly string representation of an object.
ruby class Book attr_accessor :title, :author def initialize(title, author) @title = title @author = author end end book = Book.new("Ruby Magic", "John Doe") puts book.inspect # Output: #<Book:0x00007fcf9b861b08 @title="Ruby Magic", @author="John Doe"> puts book.to_s # Output: Ruby Magic by John Doe
3.2. Navigating Object Hierarchy with #ancestors
The #ancestors method is not limited to classes; it also works on modules. This method returns an array of classes and modules in the inheritance hierarchy, which is crucial for understanding how method lookup works in Ruby’s dynamic dispatch system.
ruby module A end class B include A end class C < B end puts C.ancestors # Output: [C, B, A, Object, Kernel, BasicObject]
4. Metaprogramming with Reflection
4.1. Defining Methods Dynamically
Ruby’s Reflection API enables powerful metaprogramming, allowing you to define methods dynamically. The Module#define_method method can be used to define new instance methods within a class.
ruby class Greeter def initialize(greeting) @greeting = greeting end end greeter = Greeter.new("Hello") Greeter.define_method(:say_hello) do puts @greeting end greeter.say_hello # Output: Hello
4.2. Modifying Existing Classes with class_eval
Reflection also empowers you to modify existing classes during runtime using the class_eval method. This method allows you to execute arbitrary code within the context of a class, enabling you to add, modify, or redefine methods.
ruby class String def reverse_and_upcase reverse.upcase end end puts "reflection".reverse_and_upcase # Output: NOITCILFER
5. Use Cases for Reflection
5.1. Testing and Debugging
Reflection is particularly valuable in testing and debugging scenarios. You can use it to write comprehensive tests by dynamically generating test cases for different classes and methods. Moreover, during debugging, you can inspect the internal state of objects and classes to identify issues more effectively.
5.2. Frameworks and Libraries
Many popular Ruby frameworks and libraries utilize Reflection to achieve their functionalities. For instance, Rails’ ActiveRecord uses Reflection to map database tables to Ruby classes, and RSpec leverages it to provide a rich DSL for describing and testing code behavior.
6. Pitfalls and Best Practices
6.1. Performance Considerations
While Reflection is a powerful tool, it can have performance implications. Introspecting objects at runtime can be slower than direct method calls or property access. It’s essential to use Reflection judiciously and consider caching when necessary.
6.2. Security Implications
Reflection also introduces potential security risks. Malicious users might exploit Reflection to gain unauthorized access or manipulate objects in unexpected ways. Be cautious when dynamically evaluating user input using methods like eval and send.
Conclusion
Ruby’s Reflection API offers a window into the inner workings of your code during runtime. It empowers developers to introspect, create, and modify objects and classes dynamically, enhancing the flexibility and adaptability of applications. By leveraging Reflection, you can achieve powerful metaprogramming, build more comprehensive testing suites, and streamline the development process. However, it’s essential to balance its power with performance and security considerations. As you explore the world of Reflection, you’ll unlock a new level of understanding and control over your Ruby applications.
Table of Contents
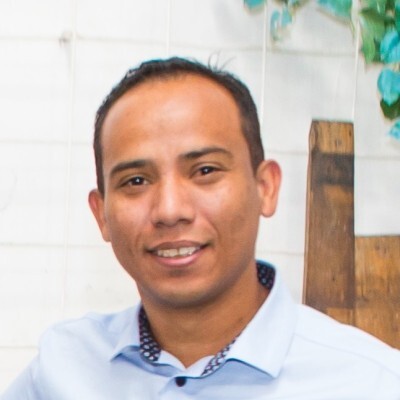
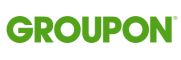