How to use regular expressions for pattern matching in Ruby?
In Ruby, regular expressions (regex or regexp) are a powerful tool for pattern matching and text manipulation. They allow you to define patterns that can be used to search, extract, or manipulate strings based on specific criteria. Here’s how you can use regular expressions in Ruby:
- Creating Regular Expressions:
You can create a regular expression in Ruby by using the `/` delimiters. For example, to create a regex that matches the word “ruby” in a case-insensitive manner, you can use:
The `i` at the end makes it case-insensitive.
- Matching Patterns:
You can use the `=~` operator to check if a string matches a regular expression:
```ruby if "I love Ruby programming" =~ /ruby/i puts "Match found!" else puts "No match." end ```
This code will output “Match found!” since “Ruby” matches the pattern.
- Match Data:
When a match is found, Ruby provides a `MatchData` object that contains information about the match, including the matched text and captured groups. You can access this data using the `$~` global variable or the `Regexp.last_match` method.
- String Methods:
Ruby’s `String` class provides methods like `scan`, `sub`, and `gsub` that allow you to work with regular expressions. For example, `scan` finds all matches in a string, `sub` replaces the first occurrence, and `gsub` replaces all occurrences.
```ruby text = "Ruby is a dynamic language. Ruby is fun." matches = text.scan(/ruby/i) ```
- Capture Groups:
You can use parentheses `()` in your regular expressions to create capture groups, which allow you to extract specific parts of a matched string.
```ruby text = "Ruby is a dynamic language. Ruby is fun." matches = text.scan(/ruby/i) ```
- Modifiers:
Ruby supports various modifiers that can be added to regular expressions to change their behavior, such as `/i` for case-insensitivity and `/m` for multiline matching.
Regular expressions are a versatile tool for text processing in Ruby, and they are widely used for tasks like input validation, text parsing, and data extraction. While they can be incredibly powerful, they can also become complex, so it’s essential to understand how to use them effectively and efficiently for your specific needs.
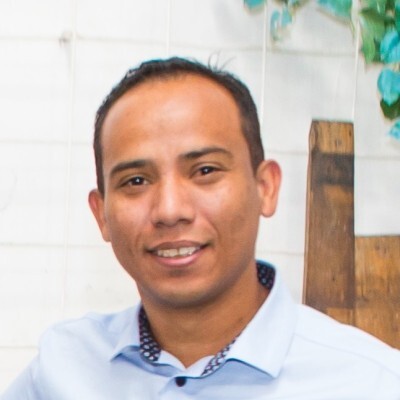
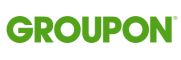