How to remove duplicates from an array in Ruby?
In Ruby, you can remove duplicates from an array using various methods and techniques, depending on your preferences and the desired outcome. Here are some common approaches:
- Using the `.uniq` Method:
The most straightforward way to remove duplicates from an array is to use the `.uniq` method. This method returns a new array with duplicate elements removed, preserving the order of the original array.
```ruby original_array = [1, 2, 2, 3, 4, 4, 5] unique_array = original_array.uniq ```
After running this code, the `unique_array` will contain `[1, 2, 3, 4, 5]`, with duplicates removed.
- Using the `|` Operator:
You can also remove duplicates by converting the array to a set and then back to an array using the `|` (union) operator.
```ruby original_array = [1, 2, 2, 3, 4, 4, 5] unique_array = original_array.to_set.to_a ```
This method removes duplicates while maintaining the order of the original array.
- Using a Custom Approach:
If you need more control over duplicate removal, you can use custom code to create a new array with duplicates removed. Here’s an example using a loop and a hash:
```ruby original_array = [1, 2, 2, 3, 4, 4, 5] unique_array = [] seen = {} original_array.each do |element| unless seen[element] unique_array << element seen[element] = true end end ```
This approach allows you to customize the logic for removing duplicates if needed.
Selecting the method for removing duplicates depends on your specific requirements, including whether you want to preserve the order of elements, maintain the original array, or customize the removal process. In most cases, the `.uniq` method provides a simple and effective way to achieve this task.
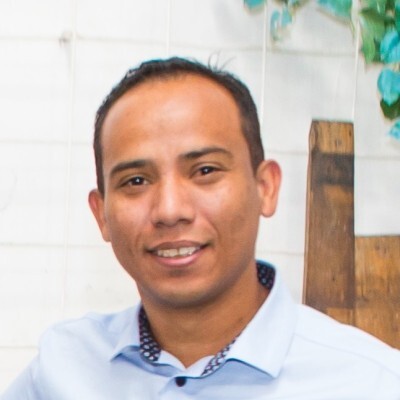
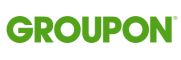