What is the ‘rescue’ clause in Ruby?
In Ruby, the `rescue` clause is a fundamental part of exception handling that allows you to define code blocks to handle specific exceptions when they occur during program execution. The primary purpose of the `rescue` clause is to gracefully manage and recover from exceptional conditions, preventing your program from terminating abruptly due to errors. Here’s a more detailed explanation of the `rescue` clause in Ruby:
- Exception Handling: The `rescue` clause is used within a `begin`…`rescue`…`end` block to catch and handle exceptions. When an exception occurs within the `begin` block, Ruby searches for a matching `rescue` block that specifies how to handle that particular exception. If a match is found, the code within the corresponding `rescue` block is executed.
```ruby begin # Code that might raise an exception result = 10 / 0 rescue ZeroDivisionError # Code to handle the ZeroDivisionError puts "Error: Division by zero" end ```
In this example, the `rescue` clause catches the `ZeroDivisionError` exception and executes the code to handle it, which displays an error message.
- Multiple Rescue Blocks: You can have multiple `rescue` blocks within the same `begin`…`rescue`…`end` block to handle different types of exceptions. Ruby will search for the first matching `rescue` block and execute its code.
```ruby begin # Code that might raise an exception rescue ZeroDivisionError # Code to handle ZeroDivisionError rescue TypeError # Code to handle TypeError end ```
- Rescue Without Specifying Exception: You can use a `rescue` block without specifying a particular exception to catch all exceptions. However, it’s generally recommended to rescue specific exceptions whenever possible to avoid masking unexpected issues.
```ruby begin # Code that might raise an exception rescue # Code to handle any exception end ```
- Ensure Block: You can include an `ensure` block after the `rescue` block to specify code that will always execute, whether an exception is raised or not. This is useful for cleanup tasks.
```ruby begin # Code that might raise an exception rescue # Code to handle any exception ensure # Code that always executes end ```
The `rescue` clause in Ruby is a critical part of exception handling, enabling you to handle specific exceptions gracefully and recover from errors, ensuring your program continues to run smoothly even when unexpected issues arise. It promotes robust and resilient code, making your applications more reliable and user-friendly.
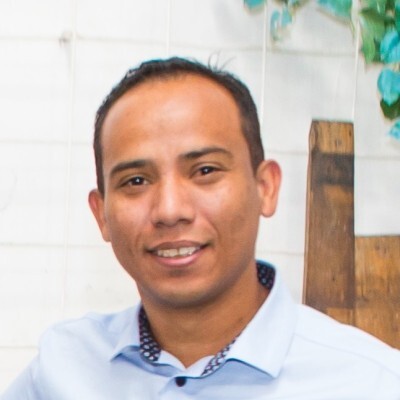
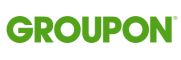