Building RESTful Web Services with Ruby and Sinatra
In today’s digital landscape, building robust and efficient web services is crucial for connecting applications and enabling seamless data exchange. Ruby, a dynamic and expressive programming language, coupled with Sinatra, a lightweight and flexible web framework, provides an excellent platform for creating RESTful web services. In this guide, we’ll take you through the process of building RESTful APIs using Ruby and Sinatra, exploring essential concepts, best practices, and hands-on code examples.
Table of Contents
1. Why Ruby and Sinatra?
Before we dive into the technical details, let’s briefly discuss why Ruby and Sinatra are a great choice for building RESTful web services.
- Expressive Syntax: Ruby’s elegant and human-readable syntax allows developers to write clean and concise code, making it easier to create and maintain APIs.
- Modularity: Sinatra is a minimalistic web framework that offers just what you need, without the complexity of larger frameworks. This modularity allows developers to choose and integrate components as required for their specific project.
- Ease of Use: Sinatra’s simplicity makes it an ideal choice for both beginners and experienced developers. It has a shallow learning curve, enabling quick development of APIs.
- Flexibility: While Sinatra is lightweight, it’s also highly customizable. You can add third-party libraries and extensions to enhance its capabilities according to your project’s needs.
2. Getting Started: Setting Up Your Environment
To start building RESTful web services with Ruby and Sinatra, you’ll need to have Ruby and the Sinatra gem installed on your machine. If you haven’t installed Ruby yet, you can download it from the official Ruby website or use a version manager like RVM or rbenv. Once you have Ruby set up, installing the Sinatra gem is as simple as running:
ruby gem install sinatra
With Sinatra installed, you’re ready to create your first RESTful web service.
3. Creating Your First API Endpoint
Let’s begin by creating a basic API endpoint that returns a simple message when accessed. Sinatra makes it incredibly easy to define routes and their corresponding actions. In a new Ruby file (e.g., app.rb), add the following code:
ruby require 'sinatra' get '/hello' do "Hello, world!" end
In this example, we’ve defined a GET route at the path “/hello”. When this route is accessed, the block of code inside the do and end keywords will be executed, and the string “Hello, world!” will be returned as the response.
4. Handling Request Parameters
Most APIs require handling different types of HTTP requests and processing parameters sent by clients. Sinatra makes it straightforward to handle request parameters. Let’s create an API endpoint that takes a user’s name as a parameter and responds with a personalized greeting:
ruby require 'sinatra' get '/greet/:name' do name = params[:name] "Hello, #{name}!" end
In this example, we’re using a route parameter :name to capture the user’s name from the URL. The params hash allows us to access this parameter and use it in our response.
5. Responding with JSON
RESTful APIs often need to respond with JSON data. Sinatra makes it easy to generate JSON responses using the json method. Let’s modify our greeting API to respond with JSON:
ruby require 'sinatra' require 'json' get '/greet/:name' do content_type :json name = params[:name] { message: "Hello, #{name}!" }.to_json end
By setting the content_type to JSON and converting a hash to JSON using to_json, we ensure that the response is properly formatted as JSON.
6. Handling POST Requests
Creating resources via POST requests is a fundamental aspect of RESTful APIs. Let’s create an API endpoint that accepts POST requests to create new tasks:
ruby require 'sinatra' require 'json' tasks = [] post '/tasks' do content_type :json request_body = JSON.parse(request.body.read) task = { id: tasks.length + 1, title: request_body['title'] } tasks << task task.to_json end
In this example, we’re storing tasks in a simple array. The POST request body is parsed as JSON, and a new task is created and added to the array.
7. Error Handling and Status Codes
Proper error handling and status codes are essential for a well-designed API. Sinatra provides a way to set the HTTP status code and return error messages. Let’s enhance our task creation API to handle errors:
ruby require 'sinatra' require 'json' tasks = [] post '/tasks' do content_type :json begin request_body = JSON.parse(request.body.read) raise 'Title missing' if request_body['title'].nil? task = { id: tasks.length + 1, title: request_body['title'] } tasks << task status 201 task.to_json rescue StandardError => e status 400 { error: e.message }.to_json end end
Here, we’ve added error handling for cases where the title is missing in the request body. We set the appropriate status code and return an error message in JSON format.
Conclusion
Building RESTful web services with Ruby and Sinatra empowers developers to create powerful APIs with minimal effort. From setting up your environment to handling various HTTP requests, Sinatra’s simplicity and Ruby’s expressiveness make the process straightforward and enjoyable. As you continue to explore and expand your knowledge, you’ll find yourself building sophisticated APIs that drive modern applications forward. So, harness the potential of Ruby and Sinatra, and start creating your own RESTful web services today!
Table of Contents
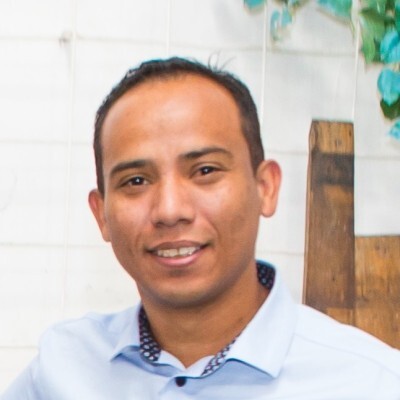
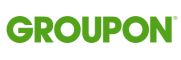