What is the ‘retry’ keyword in Ruby?
In Ruby, the `retry` keyword is used within a rescue block to reattempt the execution of a block of code that has raised an exception. It allows you to retry the same operation that failed, giving you the opportunity to handle exceptional conditions and recover gracefully from errors.
Here’s how the `retry` keyword works:
- Exception Handling: The `retry` keyword is typically used within a `rescue` block, which catches and handles exceptions that occur during the execution of a block of code. When an exception is raised, the program’s control flow jumps to the nearest `rescue` block that can handle that exception type.
- Retry: If you encounter a situation where you believe the error is temporary or can be resolved by retrying the same operation, you can place the `retry` keyword within the `rescue` block. When Ruby encounters `retry`, it will re-enter the code block that caused the exception, effectively restarting its execution.
Here’s a simple example to illustrate the use of `retry`:
```ruby attempts = 0 begin # Some code that may raise an exception result = 10 / rand(2) rescue ZeroDivisionError puts "Zero division error, retrying..." attempts += 1 retry if attempts < 3 end puts "Result: #{result}" ```
In this example, we attempt to perform a division operation that might result in a `ZeroDivisionError`. If the error occurs, we rescue it, display a message, and retry the operation up to three times (`retry if attempts < 3`). This allows us to handle the error gracefully and continue the program.
The `retry` keyword can be a powerful tool when used judiciously. However, it should be used with caution, as excessive retries or improper handling can lead to infinite loops and unexpected behavior. It’s important to ensure that the underlying issue causing the exception is resolved during the retry attempts to prevent an endless retry loop.
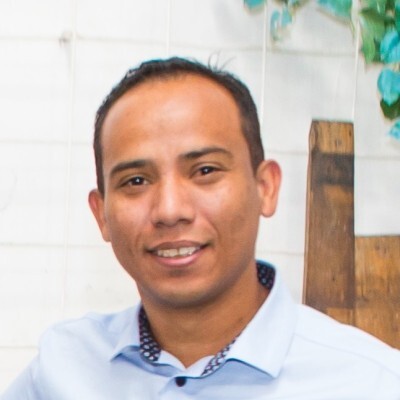
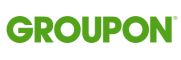