Design Patterns in Ruby: Applying Reusable Solutions
In the realm of software development, the importance of writing clean, maintainable, and efficient code cannot be overstated. As projects grow in complexity, maintaining a structured codebase becomes a significant challenge. This is where design patterns come into play. Design patterns provide a proven way to solve recurring problems in software design, promoting code reusability, scalability, and maintainability. In this blog post, we will delve into the world of design patterns in the context of the Ruby programming language, exploring various patterns and their practical applications with illustrative code examples.
Table of Contents
1. Introduction to Design Patterns
1.1. What Are Design Patterns?
Design patterns are reusable solutions to common software design problems. They provide a template for solving recurring challenges in software architecture and design. By following established design patterns, developers can benefit from tried-and-tested solutions that enhance the efficiency, maintainability, and scalability of their codebase.
1.2. Why Use Design Patterns in Ruby?
Ruby is a versatile and dynamic programming language that supports object-oriented programming (OOP) principles. Design patterns are especially useful in Ruby due to its flexibility and emphasis on OOP. By incorporating design patterns into your Ruby code, you can encapsulate behaviors, relationships, and responsibilities, resulting in a more organized and modular application.
2. Creational Design Patterns
Creational design patterns focus on object creation mechanisms, providing ways to create objects in a manner that suits the situation. Let’s explore a few key creational design patterns in Ruby.
2.1. Singleton Pattern
The Singleton pattern ensures that a class has only one instance and provides a global point of access to that instance. This can be particularly useful for managing resources that should be shared across the application.
ruby class DatabaseConnection private_class_method :new @@instance = nil def self.instance @@instance ||= new end end # Usage connection = DatabaseConnection.instance
2.2. Factory Method Pattern
The Factory Method pattern defines an interface for creating objects but allows subclasses to alter the type of objects that will be created. It promotes loose coupling between client code and the created objects.
ruby class VehicleFactory def create_vehicle raise NotImplementedError, "Subclasses must implement this method" end end class CarFactory < VehicleFactory def create_vehicle Car.new end end class BikeFactory < VehicleFactory def create_vehicle Bike.new end end # Usage car_factory = CarFactory.new car = car_factory.create_vehicle
2.3. Builder Pattern
The Builder pattern separates the construction of a complex object from its representation, allowing the same construction process to create different representations. It’s useful when dealing with objects that have multiple attributes.
ruby class PizzaBuilder def build_dough raise NotImplementedError end def build_sauce raise NotImplementedError end def build_toppings raise NotImplementedError end end class MargheritaPizzaBuilder < PizzaBuilder def build_dough "Thin crust" end def build_sauce "Tomato sauce" end def build_toppings ["Mozzarella cheese", "Fresh basil"] end end # Usage director = PizzaDirector.new(MargheritaPizzaBuilder.new) margherita_pizza = director.build_pizza
2.4. Prototype Pattern
The Prototype pattern involves creating new objects by copying an existing object, known as the prototype. It’s useful when creating objects is costly or complex.
ruby class Sheep attr_accessor :name, :category def initialize(name, category) @name = name @category = category end def clone Sheep.new(@name, @category) end end # Usage original_sheep = Sheep.new("Dolly", "Domestic") cloned_sheep = original_sheep.clone
3. Structural Design Patterns
Structural design patterns focus on class composition and object relationships. They help define how objects and classes can be combined to form larger structures while keeping the system flexible and efficient.
3.1. Adapter Pattern
The Adapter pattern allows incompatible interfaces to work together by providing a wrapper with a consistent interface. It’s particularly useful when integrating legacy code or third-party libraries.
ruby class Adaptee def specific_request "Specific request" end end class Target def request "Target request" end end class Adapter < Target def initialize(adaptee) @adaptee = adaptee end def request @adaptee.specific_request end end # Usage adaptee = Adaptee.new adapter = Adapter.new(adaptee) adapter.request
3.2. Decorator Pattern
The Decorator pattern allows behavior to be added to individual objects, either statically or dynamically, without affecting the behavior of other objects from the same class.
ruby class Coffee def cost 5 end end class MilkDecorator def initialize(component) @component = component end def cost @component.cost + 2 end end class SugarDecorator def initialize(component) @component = component end def cost @component.cost + 1 end end # Usage simple_coffee = Coffee.new milk_coffee = MilkDecorator.new(simple_coffee) sugar_milk_coffee = SugarDecorator.new(milk_coffee)
3.3. Facade Pattern
The Facade pattern provides a simplified interface to a complex system of classes, helping to reduce dependencies and improve usability.
ruby class SubsystemA def operation "Subsystem A operation" end end class SubsystemB def operation "Subsystem B operation" end end class Facade def initialize(subsystem_a, subsystem_b) @subsystem_a = subsystem_a @subsystem_b = subsystem_b end def operation result = [] result << @subsystem_a.operation result << @subsystem_b.operation result.join("\n") end end # Usage subsystem_a = SubsystemA.new subsystem_b = SubsystemB.new facade = Facade.new(subsystem_a, subsystem_b) facade.operation
3.4. Composite Pattern
The Composite pattern composes objects into tree structures to represent part-whole hierarchies. It allows clients to treat individual objects and compositions of objects uniformly.
ruby class Component def operation raise NotImplementedError end end class Leaf < Component def operation "Leaf operation" end end class Composite < Component def initialize @children = [] end def add_child(child) @children << child end def operation result = [] @children.each do |child| result << child.operation end result.join("\n") end end # Usage leaf1 = Leaf.new leaf2 = Leaf.new composite = Composite.new composite.add_child(leaf1) composite.add_child(leaf2) composite.operation
4. Behavioral Design Patterns
Behavioral design patterns focus on communication between objects and how objects collaborate. They enhance the flexibility of communication and allow you to define more effective ways of interaction.
4.1. Observer Pattern
The Observer pattern defines a one-to-many relationship between objects, where changes in one object trigger updates in dependent objects.
ruby class Subject attr_accessor :observers def initialize @observers = [] end def add_observer(observer) @observers << observer end def remove_observer(observer) @observers.delete(observer) end def notify_observers @observers.each(&:update) end end class ConcreteObserver def update puts "Observer updated" end end # Usage subject = Subject.new observer = ConcreteObserver.new subject.add_observer(observer) subject.notify_observers
4.2. Strategy Pattern
The Strategy pattern defines a family of algorithms, encapsulates them, and makes them interchangeable. It allows a client to choose an algorithm from a family of algorithms at runtime.
ruby class Strategy def execute raise NotImplementedError end end class ConcreteStrategyA < Strategy def execute "Strategy A executed" end end class ConcreteStrategyB < Strategy def execute "Strategy B executed" end end class Context def initialize(strategy) @strategy = strategy end def execute_strategy @strategy.execute end end # Usage strategy_a = ConcreteStrategyA.new context = Context.new(strategy_a) context.execute_strategy
4.3. Template Method Pattern
The Template Method pattern defines the structure of an algorithm in a base class but allows subclasses to override specific steps of the algorithm without changing its structure.
ruby class AbstractTemplate def template_method step_one step_two step_three end def step_one raise NotImplementedError end def step_two raise NotImplementedError end def step_three raise NotImplementedError end end class ConcreteTemplate < AbstractTemplate def step_one "Step one completed" end def step_two "Step two completed" end def step_three "Step three completed" end end # Usage template = ConcreteTemplate.new template.template_method
4.4. Command Pattern
The Command pattern turns a request into a stand-alone object, containing all the necessary information about the request. This decouples sender and receiver and allows for parameterization of objects with operations.
ruby class Receiver def perform_action "Action performed by receiver" end end class Command def initialize(receiver) @receiver = receiver end def execute @receiver.perform_action end end class Invoker def initialize(command) @command = command end def invoke @command.execute end end # Usage receiver = Receiver.new command = Command.new(receiver) invoker = Invoker.new(command) invoker.invoke
Conclusion
Design patterns are an invaluable tool in the software developer’s arsenal, providing standardized solutions to recurring problems. By mastering design patterns in Ruby, you can significantly improve your code’s maintainability, scalability, and reusability. We’ve explored various categories of design patterns—creational, structural, and behavioral—providing insights and practical code examples for each. As you continue your journey in software development, don’t hesitate to leverage the power of design patterns to elevate your coding skills and deliver robust, well-organized applications. Happy coding!
Table of Contents
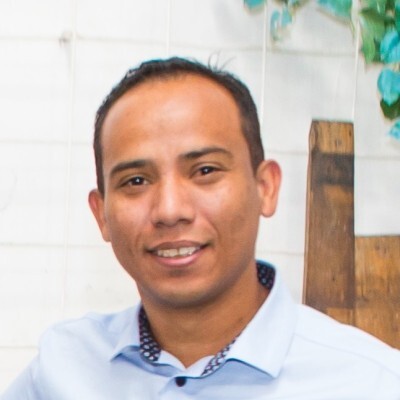
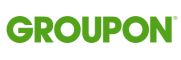