How to reverse a string in Ruby?
In Ruby, you can reverse a string in several ways, each offering different levels of simplicity and flexibility. Here are some common methods to reverse a string:
- Using the `.reverse` Method:
Ruby provides a built-in method called `.reverse` that allows you to reverse the characters in a string. You can simply call this method on a string to obtain its reverse.
```ruby original_string = "Hello, World!" reversed_string = original_string.reverse ```
The `reversed_string` will now contain “!dlroW ,olleH”.
- Using String Concatenation:
You can reverse a string by iterating through its characters in reverse order and concatenating them to a new string.
```ruby original_string = "Hello, World!" reversed_string = "" original_string.each_char { |char| reversed_string = char + reversed_string } ```
The `reversed_string` variable will hold the reversed content.
- Using the `.chars` Method and `join`:
Another approach is to use the `.chars` method to convert the string into an array of characters and then use the `.join` method to concatenate them in reverse order.
```ruby original_string = "Hello, World!" reversed_string = original_string.chars.reverse.join ```
This code will produce the same reversed string.
- Using String Indexing:
You can reverse a string by accessing its characters in reverse order using negative indices.
```ruby original_string = "Hello, World!" reversed_string = "" (original_string.length - 1).downto(0) { |i| reversed_string += original_string[i] } ```
This method explicitly loops through the characters and constructs the reversed string.
All of these methods achieve the same result, but the choice of which one to use depends on your coding style and specific requirements. The `.reverse` method is the simplest and most direct approach, while the other methods offer more fine-grained control and can be useful in certain situations.
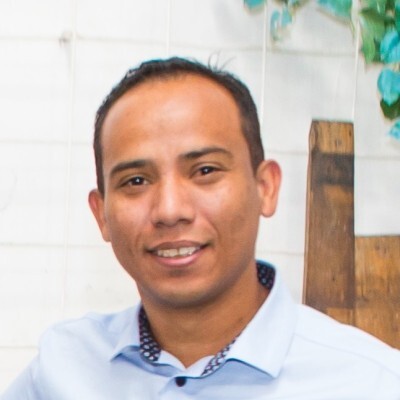
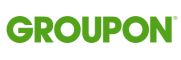