Exploring Ruby’s Functional Reactive Programming: RxRuby and Beyond
In the world of software development, staying ahead of the curve means constantly exploring new programming paradigms and tools. Functional Reactive Programming (FRP) is one such paradigm that has gained traction in recent years due to its ability to simplify the management of complex and asynchronous user interfaces. In this blog post, we’ll delve into the world of Functional Reactive Programming in Ruby, focusing on the powerful RxRuby library and also touching on the broader landscape of FRP in Ruby.
Table of Contents
1. Introduction to Functional Reactive Programming (FRP)
1.1. What is FRP?
Functional Reactive Programming (FRP) is a programming paradigm that focuses on managing and propagating changes in an application’s state over time. Unlike the traditional imperative programming, where you explicitly define step-by-step instructions, FRP revolves around the concept of streams and events. It allows you to express complex behaviors and interactions in a more declarative and elegant manner.
1.2. Why Choose FRP?
FRP brings numerous benefits to the table. By modeling your application as a set of streams and events, you can create more responsive and interactive user interfaces. This is particularly useful in scenarios where events can occur at any time, such as user interactions, network responses, or sensor data.
1.3. The Role of RxRuby
RxRuby is a powerful FRP library for Ruby developers. It’s based on the ReactiveX (Rx) paradigm and provides a wide range of tools to work with asynchronous data streams. RxRuby allows you to compose and transform streams of data, making it an ideal choice for building event-driven applications.
2. Getting Started with RxRuby
2.1. Installation and Setup
To begin exploring RxRuby, you’ll need to install the library. You can do this using the following command:
ruby gem install rx_ruby
Once installed, you can start utilizing RxRuby in your projects.
2.2. Observables and Observers
At the core of RxRuby are two key components: observables and observers. Observables represent a source of data that emits events over time. Observers, on the other hand, are entities that subscribe to these observables to receive and react to events.
2.3. Creating Observables
Creating an observable is straightforward:
ruby require 'rx_ruby' observable = RxRuby::Observable.create do |observer| observer.on_next("Hello") observer.on_next("World") observer.on_completed end
2.4. Subscribing to Observables
To listen to events emitted by an observable, you need to subscribe to it:
ruby subscription = observable.subscribe( lambda { |value| puts value }, lambda { |error| puts "Error: #{error.message}" }, lambda { puts "Completed" } ) # Don't forget to dispose of the subscription when you're done subscription.dispose
3. Working with Streams and Events
3.1. Streams in FRP
In FRP, streams are sequences of events that occur over time. These events can be anything from user inputs to HTTP responses. RxRuby provides a variety of operators to work with streams and manipulate events as they flow through your application.
3.2. Creating Stream of Events
Let’s say you want to create a stream that emits a random number every second:
ruby number_stream = RxRuby::Observable.interval(1) .map { rand(1..100) }
Here, interval(1) emits an event every second, and map transforms the emitted value into a random number between 1 and 100.
3.3. Transforming and Filtering Events
You can apply various operators to transform and filter events in a stream. For instance, you might want to only consider even numbers:
ruby even_number_stream = number_stream.filter { |num| num.even? }
3.4. Combining Multiple Streams
In many scenarios, you’ll need to combine multiple streams to create more complex behaviors. RxRuby offers operators like merge, concat, and combine_latest to help you achieve this.
ruby combined_stream = RxRuby::Observable.merge(stream1, stream2)
4. Managing State with RxRuby
4.1. State as an Observable
In traditional programming, managing state changes and updates can become complex. However, in FRP, you can model state as an observable stream. This allows you to easily track changes and propagate them throughout your application.
4.2. State Mutation and Immutability
In FRP, immutability is crucial. Instead of directly modifying state, you create new instances with the updated values. RxRuby provides operators like scan that make managing state changes more intuitive:
ruby state_stream = RxRuby::Observable.from_array([0]) .scan { |acc, value| acc + value }
4.3. Reducing State with Operators
You can use various operators to aggregate and manipulate the state stream. For example, you might want to calculate the sum of the last three values:
ruby sum_stream = state_stream.buffer(3, 1) .map { |values| values.sum }
4.4. Handling Errors and Side Effects
FRP allows you to handle errors and side effects more elegantly. Operators like catch and do_on_next can be used to intercept errors and perform side effects, respectively.
ruby error_handling_stream = stream.catch { |error| RxRuby::Observable.return(error_message) }
5. Building Responsive UI with RxRuby
5.1. Event Handling in UI
FRP shines in UI development by simplifying event handling. You can create streams of user interactions and respond to them with ease.
5.2. Declarative UI Updates
Traditional imperative programming often involves manually updating UI elements. With FRP, you can declaratively express how UI elements should react to changes in the underlying data stream.
5.3. Reacting to User Input
Consider a scenario where you want to enable a button only when a user enters valid input:
ruby input_stream = RxRuby::Observable.from_event(input_field, 'input') valid_input_stream = input_stream .map { |event| event.target.value } .map { |value| value.length >= 8 } valid_input_stream.subscribe { |valid| submit_button.disabled = !valid }
5.4. Throttling and Debouncing
FRP also provides mechanisms to manage event frequency. Throttling and debouncing allow you to control how often events are emitted from a stream. This can be useful to prevent excessive updates, such as in search bars.
ruby search_stream = RxRuby::Observable.from_event(search_input, 'input') throttled_search_stream = search_stream.throttle(300) # Throttle events every 300ms
6. Beyond RxRuby: Exploring Other FRP Libraries
While RxRuby is a powerful choice for FRP in Ruby, there are other libraries worth exploring as well:
6.1. Frappuccino
Frappuccino is a lightweight FRP library that focuses on simplicity. It’s great for smaller projects and getting started quickly.
6.2. ReactiveRuby
ReactiveRuby integrates FRP concepts into the Ruby on Rails framework, allowing you to build reactive web applications with ease.
6.3. Celluloid
Celluloid offers a more actor-based approach to FRP, providing concurrency and parallelism for improved performance.
6.4. Comparing Different Libraries
Each library has its strengths and weaknesses, so it’s important to consider your project’s requirements before choosing one. RxRuby is known for its robustness and extensive operator set, making it suitable for a wide range of applications.
7. Real-world Applications of FRP in Ruby
FRP’s benefits extend beyond theoretical concepts. It’s actively used in various domains to create powerful and responsive applications:
7.1. GUI Applications
FRP is highly beneficial in creating graphical user interfaces. The declarative approach simplifies event handling and UI updates.
7.2. Real-time Data Processing
In applications that deal with real-time data, FRP can help manage and process streams of incoming data efficiently.
7.3. Game Development
FRP is well-suited for game development, where events and interactions are central to gameplay. Libraries like RxRuby can simplify input handling and game state management.
7.4. IoT Applications
In the Internet of Things (IoT) domain, FRP can be used to manage and react to sensor data, making it easier to create responsive and adaptive systems.
8. Best Practices and Considerations
8.1. Choosing the Right Paradigm
While FRP offers numerous advantages, it might not be the best fit for every project. Consider the complexity of your application and whether FRP aligns with your team’s expertise.
8.2. Designing for Composition
FRP promotes composability. Design your application in a modular way, focusing on small, reusable components that can be easily combined.
8.3. Handling Complex Scenarios
As with any programming paradigm, complex scenarios can arise. Utilize the wide array of operators provided by RxRuby to tackle intricate situations effectively.
8.4. Testing and Debugging FRP Code
Testing and debugging FRP code can be different from traditional imperative code. Use RxRuby’s testing utilities and debugging tools to ensure your code behaves as expected.
Conclusion
Functional Reactive Programming, with its focus on streams and events, provides a fresh perspective on building responsive and event-driven applications. RxRuby, as a powerful FRP library for Ruby, equips developers with the tools needed to manage complex asynchronous behavior while keeping code elegant and maintainable. By exploring RxRuby and other FRP libraries, you can unlock the potential to create more interactive user interfaces, handle real-time data efficiently, and revolutionize the way you develop software in Ruby. So, embrace the paradigm shift and dive into the world of Functional Reactive Programming in Ruby – your applications and users will thank you.
Table of Contents
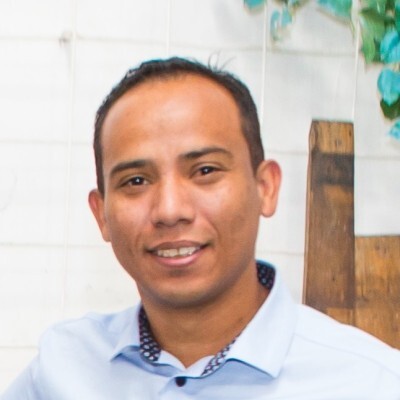
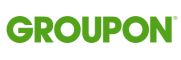