Scaling Ruby on Rails Applications: Strategies for High-Performance
In today’s fast-paced digital landscape, building web applications that can handle high levels of traffic and deliver seamless user experiences is essential. Ruby on Rails (RoR) has gained popularity for its rapid development capabilities and elegant code structure, but as your application grows, ensuring it maintains high performance becomes a critical challenge. In this blog, we’ll explore strategies and best practices for scaling Ruby on Rails applications to achieve exceptional performance even under heavy loads.
Table of Contents
1. Understanding the Scaling Challenge
Scaling a Ruby on Rails application involves optimizing its performance as user demand increases. This is crucial to prevent slow response times, downtime, and a negative user experience. As the user base grows, you need to ensure that your application can handle concurrent requests, maintain database efficiency, and utilize server resources effectively.
1.1. Horizontal vs. Vertical Scaling
Before delving into strategies, let’s differentiate between two common scaling approaches: horizontal and vertical scaling.
- Horizontal Scaling: Involves adding more servers to distribute the load. This is often achieved using a load balancer that routes incoming requests across multiple server instances.
- Vertical Scaling: Involves upgrading the existing server hardware to handle increased load. This might include adding more RAM, CPU power, or other resources to a single server.
2. Performance Optimization Strategies
2.1. Efficient Database Queries
Database queries are often a performance bottleneck. Optimize queries by using proper indexing, avoiding N+1 query problems, and utilizing the ActiveRecord’s query optimization methods.
ruby # Avoid N+1 queries using eager loading @posts = Post.includes(:comments) # Use indexes to speed up queries add_index :users, :email
2.2. Caching
Implement caching to store frequently accessed data and reduce the load on the database. Ruby on Rails supports various caching mechanisms, including page caching, fragment caching, and HTTP caching.
ruby # Fragment caching example <% cache @user do %> <div class="user-profile"> <!-- User profile content --> </div> <% end %>
2.3. Asynchronous Processing
Offload time-consuming tasks to background jobs using tools like Sidekiq or Resque. This prevents your main application from getting bogged down by tasks that don’t need to be processed in real-time.
ruby # Using Sidekiq for background jobs class NotificationWorker include Sidekiq::Worker def perform(user_id, message) # Send notification to user end end
2.4. Load Balancing
Implement a load balancer to distribute incoming requests across multiple server instances. This improves response times and ensures high availability.
ruby # Load balancing configuration example in Nginx upstream rails_app { server app_server1; server app_server2; } server { listen 80; server_name yourdomain.com; location / { proxy_pass http://rails_app; } }
2.5. Optimize Asset Delivery
Efficiently deliver assets like images, stylesheets, and JavaScript files by utilizing content delivery networks (CDNs) and asset compression.
ruby # Using the asset pipeline for asset optimization <%= stylesheet_link_tag 'application', media: 'all' %> <%= javascript_include_tag 'application' %>
3. Scalability Best Practices
3.1. Use Background Jobs for Long Processes
Certain operations, such as sending emails or generating reports, can take a long time. Instead of making users wait, offload these tasks to background jobs.
3.2. Employ a CDN for Static Assets
Content Delivery Networks (CDNs) distribute static assets to servers worldwide, reducing the load on your main application server and decreasing load times for users globally.
3.3. Choose the Right Hosting Provider
Select a hosting provider that supports your application’s growth. Cloud platforms like AWS, Google Cloud, and Heroku offer scalable infrastructure options.
3.4. Monitor and Optimize
Regularly monitor your application’s performance using tools like New Relic or Scout. Identify bottlenecks and optimize accordingly.
3.5. Scale the Database
As your application grows, your database might become a performance bottleneck. Consider database sharding, read replicas, or using specialized databases like Redis for caching.
4. Case Study: Twitter’s Scaling Strategy
Twitter, built on Ruby on Rails, faced immense scaling challenges due to its rapid growth. They employed several strategies to handle the high demand:
- Sharding: Twitter sharded its database to distribute the load across multiple servers. Each shard held a portion of user data, tweets, and interactions.
- Caching: Caching played a crucial role. Twitter used a combination of in-memory caching and distributed caches to reduce database load.
- Asynchronous Processing: Background jobs were extensively used for tasks like tweet processing, notifications, and email delivery.
- Load Balancing: Twitter employed load balancers to distribute requests across multiple servers, ensuring high availability.
Conclusion
Scaling Ruby on Rails applications for high performance is a continuous effort that involves a combination of code optimization, infrastructure upgrades, and smart architectural decisions. By following the strategies and best practices outlined in this article, you can ensure your application maintains exceptional performance even as it handles increasing traffic and user demands. Remember, the key is to plan for scalability from the start and to regularly monitor, test, and optimize your application as it grows. With the right approach, you can deliver a seamless user experience regardless of the traffic volume.
Table of Contents
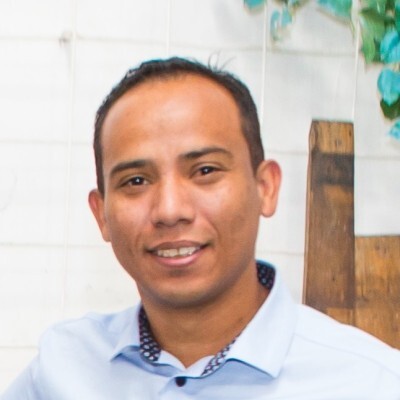
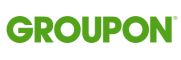