Secure Coding in Ruby: Protecting Your Applications
In today’s digital landscape, software security is paramount. With cyber threats and data breaches on the rise, developers must prioritize secure coding practices to protect their applications and users. Ruby, a popular and powerful programming language, is widely used for web development and offers a range of tools and practices to build secure applications. In this blog, we’ll explore the best practices and techniques for secure coding in Ruby, empowering you to fortify your applications against potential attacks.
Table of Contents
1. Understanding Ruby Security
Before diving into the specifics of secure coding, let’s briefly understand the security aspects of Ruby as a language. Ruby’s design philosophy promotes programmer productivity, but this should not come at the cost of security. Here are some inherent security features of Ruby:
1.1. Sandboxing
Ruby employs a sandbox mechanism, allowing you to restrict access to potentially harmful operations. This feature enables you to run untrusted code in a controlled environment, preventing malicious code from causing harm.
1.2. Secure APIs
Ruby’s standard library provides secure APIs for various tasks, such as data serialization, cryptography, and input/output operations. By using these APIs, you can mitigate common security risks associated with these tasks.
1.3. String Encoding
Ruby’s string encoding system helps avoid common security vulnerabilities like SQL injection and cross-site scripting (XSS). Always ensure proper encoding and escaping when handling user-generated data.
2. Secure Coding Best Practices
To create robust and secure Ruby applications, follow these essential best practices:
2.1. Input Validation
Validate and sanitize all user inputs to prevent malicious data from reaching sensitive parts of your application. Utilize regular expressions, validation libraries, or built-in methods like String#match to enforce input validation.
ruby # Example of input validation using a regular expression if params[:user_input] =~ /^[a-zA-Z0-9]+$/ # Process the input securely else # Handle the error or reject the input end
2.2. Parameterized Queries
When dealing with databases, use parameterized queries or prepared statements instead of constructing SQL queries with string interpolation. This prevents SQL injection attacks.
ruby # Avoid query = "SELECT * FROM users WHERE username = '#{user_input}' AND password = '#{password}'" # Prefer query = "SELECT * FROM users WHERE username = ? AND password = ?" result = db.execute(query, user_input, password)
2.3. Authentication and Authorization
Implement robust authentication and authorization mechanisms to ensure that only authenticated and authorized users can access specific parts of your application.
ruby # Example of user authentication def authenticate_user(username, password) user = User.find_by(username: username) if user && user.authenticate(password) # Authentication successful, generate and return an access token generate_access_token(user.id) else # Authentication failed nil end end
2.4. Password Security
Enforce strong password policies and use secure password hashing algorithms like bcrypt to store user passwords securely.
ruby require 'bcrypt' # Generating a secure password hash password = 'my_password' hashed_password = BCrypt::Password.create(password) # Verifying the password if BCrypt::Password.new(hashed_password) == password # Password is correct else # Password is incorrect end
2.5. Cross-Site Scripting (XSS) Prevention
Prevent XSS attacks by properly escaping and encoding user-generated content before rendering it in the application’s views.
ruby # Using Rails' built-in `h` method for HTML escaping <%= h(user_input) %>
2.6. Cross-Site Request Forgery (CSRF) Protection
Protect your application from CSRF attacks by using CSRF tokens and verifying them on each non-GET request.
ruby # Using Rails' built-in CSRF protection <%= csrf_meta_tags %>
3. Secure Third-Party Libraries
While using third-party libraries can enhance productivity, they can also introduce security risks if not carefully selected and maintained. Consider the following tips:
3.1. Regularly Update Dependencies
Keep your Ruby gems and libraries up to date by monitoring security advisories and updates from their maintainers.
ruby # Use bundler to manage dependencies and update gems $ bundle update
3.2. Verify Library Reputation
Before integrating a new library into your project, research its reputation, community support, and history of security issues.
3.3. Monitor Vulnerability Databases
Stay informed about the latest vulnerabilities by subscribing to security databases like the National Vulnerability Database (NVD) or Ruby’s vulnerability announcement mailing list.
4. Secure File Handling
Handle files with care to avoid security breaches, unauthorized access, and code injections.
4.1. File Uploads
If your application allows file uploads, validate the file type and use a secure directory for storage. Rename uploaded files to prevent directory traversal attacks.
ruby def secure_file_upload(file) if file.content_type =~ /^image\// # Proceed with secure file handling and storage else # Reject the file end end
4.2. File Read/Write Operations
When reading or writing files, avoid using hardcoded paths. Instead, use relative paths to ensure portability across different environments.
ruby # Avoid File.open('/path/to/file.txt', 'w') { |file| file.write('data') } # Prefer File.open(File.join(__dir__, 'file.txt'), 'w') { |file| file.write('data') }
5. Regular Security Audits
Perform regular security audits to identify and fix potential vulnerabilities in your Ruby application. Consider employing static code analysis tools and security scanners to automate the process.
Conclusion
By following secure coding practices in Ruby, you can significantly reduce the risk of security breaches and protect your applications and users from potential threats. Regularly update your knowledge about emerging security risks and apply appropriate security measures throughout your development process. Remember that security is an ongoing effort, and staying vigilant is essential to safeguarding your valuable assets in today’s ever-changing threat landscape. Happy coding!
Table of Contents
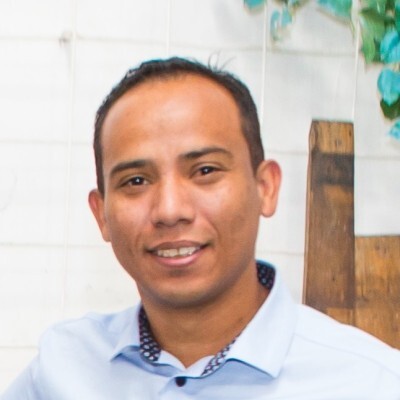
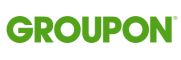