What is the ‘self’ keyword in Ruby?
In Ruby, the `self` keyword is a fundamental concept that refers to the current object or the current instance of a class. It plays a crucial role in determining the context in which code is executed and in accessing both instance and class-level methods and variables.
Here are some key aspects of the `self` keyword in Ruby:
- Instance Methods: Inside an instance method, `self` refers to the instance of the class on which the method was called. It allows you to access and modify instance variables specific to that instance. For example:
```ruby class Person def initialize(name) @name = name end def introduce "Hi, I'm #{@name}." end end person = Person.new("Alice") puts person.introduce # Output: Hi, I'm Alice. ```
In the `introduce` method, `self` refers to the `person` instance.
- Class Methods: Inside a class method, `self` refers to the class itself. It allows you to define and access class-level variables and methods. Class methods are defined using the `self` keyword followed by a dot. For example.
```ruby class MyClass def self.my_class_method "This is a class method." end end puts MyClass.my_class_method # Output: This is a class method. ```
In the `my_class_method`, `self` refers to the class `MyClass`.
- Implicit Receiver: In Ruby, you can omit the explicit use of `self` when calling methods or accessing variables. Ruby will automatically determine the context and apply `self` accordingly. For instance:
```ruby class Dog def initialize(name) @name = name end def bark "Woof, I'm #{@name}!" end end dog = Dog.new("Buddy") puts dog.bark # Output: Woof, I'm Buddy! ```
In the `bark` method, `@name` is implicitly treated as `self.@name`, where `self` refers to the `dog` instance.
Understanding the `self` keyword is essential for working effectively with Ruby classes, as it helps you manage instance-specific and class-specific behavior, variables, and methods. It’s a core concept in object-oriented programming in Ruby and provides the foundation for encapsulation and object-oriented design principles.
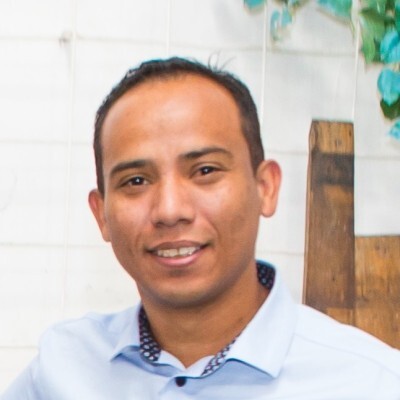
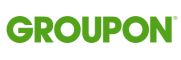