Exploring Ruby’s Standard Library: Powerful Tools at Your Fingertips
When we think about Ruby, we often focus on its elegant syntax, object-oriented nature, and the vibrant community that supports it. However, one aspect of Ruby that often goes unnoticed but is incredibly valuable is its Standard Library. The Ruby Standard Library is a collection of pre-built modules and classes that come bundled with the language installation. These modules cover a wide range of functionalities, from file manipulation to network programming, data serialization to regular expressions, and much more. In this blog post, we’re going to delve into the treasures of Ruby’s Standard Library, exploring some powerful tools that are readily available at your fingertips.
Table of Contents
1. The Richness of Ruby’s Standard Library
The Ruby Standard Library is a testament to the language’s design philosophy of “convention over configuration.” It offers a plethora of features and functionalities that might otherwise require third-party gems or libraries in other languages. This makes Ruby not only a joy to work with but also highly productive. Let’s take a look at some key areas within the Standard Library that can significantly boost your coding productivity.
1.1. File and Path Manipulation
Managing files and directories is a common task in many programming projects. Ruby’s Standard Library provides modules like File, Dir, and Pathname that make these tasks a breeze. Here’s an example of how you can create a new directory and write a file within it using these modules:
ruby require 'fileutils' dir_name = 'my_directory' FileUtils.mkdir(dir_name) file_path = File.join(dir_name, 'sample.txt') File.write(file_path, 'Hello, Ruby Standard Library!')
1.2. Regular Expressions
Regular expressions are a powerful tool for text processing and pattern matching. Ruby’s Regexp module offers extensive support for working with regular expressions. Here’s a simple example of using a regular expression to extract email addresses from a text:
ruby text = 'Contact us at email@example.com or support@domain.com' pattern = /\b[\w\.-]+@[\w\.-]+\.\w+\b/ matches = text.scan(pattern) puts matches
1.3. JSON and YAML
Dealing with data serialization formats like JSON and YAML is a common requirement in modern applications. Ruby’s Standard Library provides the json and yaml modules for working with these formats:
ruby require 'json' require 'yaml' data = { name: 'John', age: 30, city: 'Exampleville' } # Convert to JSON json_data = data.to_json puts json_data # Convert to YAML yaml_data = data.to_yaml puts yaml_data
1.4. Networking and HTTP
Ruby’s Standard Library allows you to perform network-related tasks easily. The net/http module provides functionality for making HTTP requests. Here’s a simple example of fetching a web page using this module:
ruby require 'net/http' url = URI('https://www.example.com') response = Net::HTTP.get_response(url) puts response.body if response.is_a?(Net::HTTPSuccess)
1.5. Date and Time
Working with dates and times is a fundamental aspect of many applications. Ruby’s Standard Library offers the date and time modules, which provide comprehensive support for date and time manipulation:
ruby require 'date' current_date = Date.today puts "Today's date: #{current_date}" future_date = current_date + 7 puts "Date after 7 days: #{future_date}"
2. Unlocking the Hidden Potential
Now that we’ve explored some key areas within Ruby’s Standard Library, it’s important to note that this is just the tip of the iceberg. The Standard Library offers numerous modules that can greatly simplify complex tasks. To get the most out of it, here are a few tips:
2.1. Documentation is Your Friend
The Ruby documentation is a goldmine of information. When exploring the Standard Library, refer to the official documentation to understand the capabilities and proper usage of each module.
2.2. Experiment and Practice
The best way to grasp the power of the Standard Library is to experiment with different modules. Create small projects or scripts that utilize various functionalities. This hands-on approach will solidify your understanding and make you more confident in using these tools.
2.3. Read Open Source Code
Many open-source Ruby projects rely on the Standard Library. By studying their source code, you can learn how experienced developers leverage these modules to build robust and efficient applications.
2.4. Combine with Third-Party Gems
While the Standard Library is comprehensive, there are situations where third-party gems might offer additional features or optimizations. Don’t hesitate to combine the power of the Standard Library with gems when needed.
Conclusion
Ruby’s Standard Library is a treasure trove of tools that can significantly enhance your productivity as a developer. Its diverse modules cover a wide range of domains, from file manipulation to network programming, making it an indispensable part of the Ruby programming ecosystem. By exploring and mastering the functionalities offered by the Standard Library, you’ll not only write cleaner and more efficient code but also gain a deeper understanding of the language itself. So, the next time you embark on a Ruby programming journey, remember that the powerful tools you need might already be at your fingertips, waiting to be discovered in the Ruby Standard Library.
Table of Contents
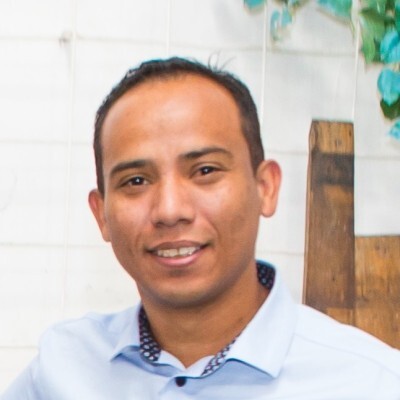
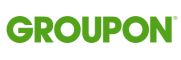