Deploying Ruby Applications: Strategies and Deployment Tools
Deploying Ruby applications is a crucial aspect of software development. It involves the process of taking your Ruby code from a local development environment and making it available to users on the internet or within your organization. A successful deployment ensures that your application runs smoothly, is scalable, and provides a great user experience.
Table of Contents
In this blog post, we will explore various strategies and deployment tools to help you deploy your Ruby applications efficiently. Whether you are deploying a web application, API, or any other Ruby-based project, understanding these strategies and tools will save you time, reduce errors, and make the deployment process more manageable.
1. Understanding the Importance of Deployment
Before diving into the deployment strategies and tools, it’s crucial to recognize why deployment is such a crucial phase in the software development lifecycle. A successful deployment ensures that the application reaches the users without any hiccups and performs as expected. Additionally, deployment allows developers to gather real-world feedback, analyze performance, and make iterative improvements.
2. Different Deployment Strategies
2.1. Manual Deployment
Manual deployment is a straightforward approach where developers manually copy the code and its dependencies to the target server. While it may be suitable for small projects or early development stages, it quickly becomes cumbersome and error-prone as the application grows.
2.2. Continuous Integration/Continuous Deployment (CI/CD)
CI/CD is a modern and widely adopted approach that automates the deployment process. With CI/CD, developers continuously integrate their code into a shared repository, run automated tests, and deploy the application automatically to staging or production environments when tests pass. This approach ensures that each code change is thoroughly tested before reaching the end-users.
2.3. Containerization
Containerization, often done using tools like Docker, allows developers to package their applications along with all required dependencies and configurations into a single container. This container can be easily deployed across different environments, ensuring consistency and reducing the risk of discrepancies between development, staging, and production environments.
3. Essential Deployment Tools
3.1. Capistrano
Capistrano is a popular Ruby deployment tool that automates the process of deploying applications to one or more servers. It simplifies tasks such as code updates, asset compilation, database migrations, and more. Capistrano’s flexibility and ease of use make it a preferred choice for deploying Ruby applications.
ruby # Capfile # Load DSL and set up stages require "capistrano/setup" # Include default deployment tasks require "capistrano/deploy" # Load the SCM plugin appropriate to your project: require "capistrano/scm/git" install_plugin Capistrano::SCM::Git # Load custom tasks from your `lib/capistrano/tasks` directory Dir.glob("lib/capistrano/tasks/*.rake").each { |r| import r }
3.2. Heroku
Heroku is a cloud platform that offers a fully-managed environment for deploying, managing, and scaling applications. It simplifies the deployment process, allowing developers to focus on writing code without worrying about infrastructure management. Heroku supports various Ruby web frameworks, making it an excellent choice for web application deployment.
3.3. Docker
Docker is a powerful containerization platform that enables developers to create, deploy, and run applications in isolated containers. Using Docker, you can ensure that your Ruby application runs consistently across different environments, from development to production.
Dockerfile # Dockerfile FROM ruby:2.7 # Set working directory WORKDIR /app # Install dependencies COPY Gemfile Gemfile.lock ./ RUN bundle install # Copy application code COPY . . # Start the application CMD ["ruby", "app.rb"]
3.4. Kubernetes
Kubernetes is a container orchestration platform that automates the deployment, scaling, and management of containerized applications. While it has a steeper learning curve compared to other tools, Kubernetes provides unparalleled flexibility and scalability for Ruby applications.
4. Building a Deployment Pipeline
4.1. Setting up a Version Control System (VCS)
Version control is essential for tracking changes to your codebase, collaborating with other developers, and enabling easy rollbacks if needed. Git is the most widely used version control system, and platforms like GitHub or GitLab provide excellent hosting options for Ruby projects.
4.2. Implementing Automated Tests
Automated tests play a vital role in ensuring that your application functions correctly and remains stable after each deployment. Tools like RSpec and Capybara are commonly used for writing tests in Ruby.
ruby # RSpec Example # spec/calculator_spec.rb require "calculator" RSpec.describe Calculator do describe "#add" do it "returns the sum of two numbers" do calculator = Calculator.new result = calculator.add(2, 3) expect(result).to eq(5) end end end
4.3. Staging Environment
The staging environment serves as a pre-production environment where you can test your application in conditions that mimic the production setup. This step helps catch any issues that may arise when deploying to the production environment.
4.4. Production Environment
The production environment is where your application is accessible to end-users. It’s essential to have strict security measures, load balancing, and performance monitoring in place to ensure a smooth user experience.
5. Code Samples and Best Practices
5.1. Configuring Environment Variables
Use environment variables to store sensitive information like API keys, database credentials, and other configuration details. Popular gems like dotenv and Figaro help manage environment variables in Ruby applications.
ruby # .env DATABASE_URL=postgres://username:password@localhost/mydatabase API_KEY=your_api_key
5.2. Database Migrations
When deploying updates that require changes to the database schema, use database migrations to manage these changes smoothly.
ruby # db/migrate/20230717094300_create_users.rb class CreateUsers < ActiveRecord::Migration[6.1] def change create_table :users do |t| t.string :name t.integer :age t.timestamps end end end
5.3. Logging and Error Handling
Implement comprehensive logging and error handling mechanisms in your application to track issues, monitor performance, and troubleshoot problems efficiently.
ruby # Logging Example # app.rb require "sinatra" get "/" do logger.info("Processing request...") # Your application logic here end
6. Monitoring and Scaling
6.1. Monitoring Tools
Use monitoring tools like New Relic or Prometheus to collect valuable data about your application’s performance, resource usage, and potential bottlenecks.
6.2. Scaling Strategies
Implement scaling strategies, such as horizontal scaling with load balancers or vertical scaling with more powerful servers, to accommodate increased user demand and ensure high availability.
Conclusion
Deploying Ruby applications can be a seamless and efficient process when using the right strategies and deployment tools. From manual deployment to modern CI/CD pipelines and containerization, the choices are vast, each catering to different application needs. By understanding these strategies and adopting best practices, you can confidently deploy your Ruby applications, delivering a robust and reliable experience to your users.
Table of Contents
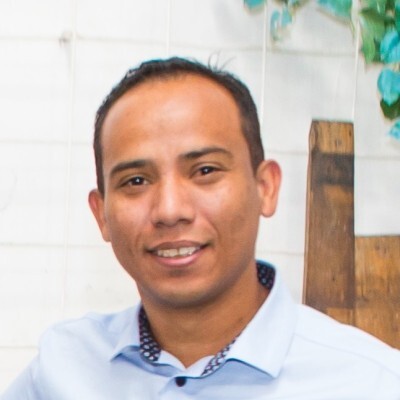
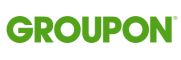