What is string interpolation in Ruby?
String interpolation in Ruby is a powerful feature that allows you to embed expressions, variables, or method calls within a string. When a string contains interpolation, Ruby evaluates the embedded content and replaces it with the resulting value, creating a new string. String interpolation is commonly used for constructing dynamic strings with variable data or including the results of calculations within a string.
In Ruby, string interpolation is typically done within double-quoted strings (`” “`). You can use the `#{}` sequence to enclose the expression or variable you want to interpolate. Here’s a simple example:
```ruby name = "Alice" age = 30 greeting = "Hello, #{name}! You are #{age} years old." puts greeting ```
In this code, `#{name}` and `#{age}` are placeholders that get replaced with the values of the `name` and `age` variables when the `greeting` string is constructed. The output will be:
``` Hello, Alice! You are 30 years old. ```
String interpolation not only makes your code more concise and readable but also provides a convenient way to include dynamic data in your strings. You can interpolate variables, method results, or even complex expressions, making it a valuable tool for generating customized messages, formatting output, and constructing SQL queries, among other use cases. It’s an essential feature for building flexible and expressive Ruby programs.
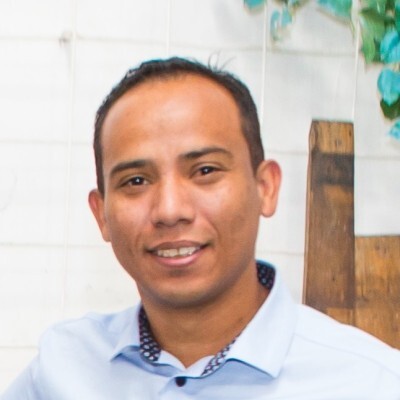
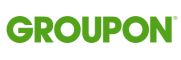