How to use the ‘super’ keyword in Ruby?
In Ruby, the `super` keyword is used to call a method with the same name as the one in which `super` appears but in a superclass. It allows you to invoke the superclass’s implementation of a method from within the subclass’s method. The primary purpose of `super` is to extend or override the behavior of a method defined in a superclass while preserving some of its functionality.
Here’s how you can use the `super` keyword in Ruby:
- Call a Superclass Method: You can use `super` inside a subclass to call the corresponding method in the superclass. This is particularly useful when you want to extend the functionality of a method without entirely redefining it. Here’s an example:
```ruby class Parent def greet "Hello from the Parent class!" end end class Child < Parent def greet super + " And Hello from the Child class!" end end child = Child.new puts child.greet ```
In this example, the `super` keyword is used in the `Child` class’s `greet` method to invoke the `Parent` class’s `greet` method and then append additional text.
- Pass Arguments to Superclass Methods: You can also pass arguments to the superclass method using `super`. This allows you to customize the behavior of the superclass method while still leveraging its functionality. Here’s an example:
```ruby class Parent def add(a, b) a + b end end class Child < Parent def add(a, b) result = super(a, b) "The sum is #{result}" end end child = Child.new puts child.add(5, 3) ```
Here, the `super(a, b)` call passes the arguments `a` and `b` to the `add` method in the `Parent` class, and the `Child` class customizes the output.
Using the `super` keyword allows you to follow the DRY (Don’t Repeat Yourself) principle by reusing existing code in the superclass while adding specific behavior in subclasses. It’s a valuable tool for creating flexible and maintainable class hierarchies in Ruby.
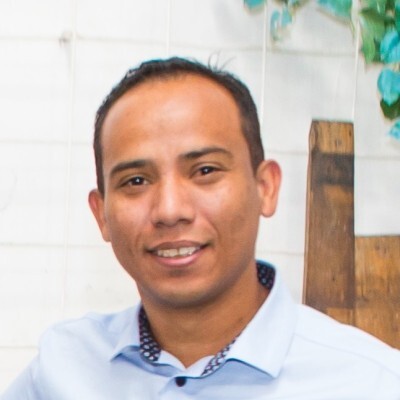
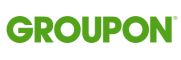