Testing Ruby Applications: Unit Testing and Test-Driven Development (TDD)
Testing is an indispensable part of software development that ensures the reliability and robustness of applications. In the realm of Ruby programming, two fundamental approaches dominate the testing landscape: Unit Testing and Test-Driven Development (TDD). In this blog, we will delve into the world of testing Ruby applications, exploring the concepts of Unit Testing and TDD, and how they contribute to building high-quality, maintainable, and bug-free code.
Table of Contents
1. Why Testing is Crucial
Before diving into the specifics of Unit Testing and TDD, let’s understand why testing is crucial for Ruby applications. Testing plays a pivotal role in:
- Bug Detection: Tests help identify bugs and errors in the code, ensuring that issues are caught early in the development process, making debugging less cumbersome and time-consuming.
- Code Confidence: Robust test suites instill confidence in developers and project stakeholders, allowing them to make changes to the codebase with the assurance that existing functionality remains intact.
- Refactoring Safety: A well-tested codebase provides a safety net during refactoring. Developers can confidently make changes, knowing that the tests will catch any regressions.
- Documentation: Tests act as living documentation for the codebase, showcasing how various parts of the application should work.
- Collaboration: A comprehensive test suite fosters seamless collaboration among developers, making it easier to work on shared codebases.
- Continuous Integration: Automated tests are an essential part of continuous integration pipelines, ensuring that new code doesn’t introduce breaking changes.
Now that we understand the importance of testing, let’s explore two essential approaches to testing Ruby applications: Unit Testing and Test-Driven Development (TDD).
2. Unit Testing in Ruby
Unit Testing involves testing individual units or components of an application in isolation. In the context of Ruby applications, a unit typically refers to a method or function. The primary goal of unit testing is to ensure that each unit functions correctly in isolation.
A unit test should be:
- Fast: Unit tests should execute quickly, allowing developers to run them frequently during development.
- Isolated: Each unit test must run independently of other tests and not rely on external resources or state.
- Deterministic: Unit tests should produce the same result every time they are run.
- Focused: Each test should focus on testing a specific aspect or behavior of the unit.
3. Writing a Unit Test in Ruby
Consider the following simple Ruby method that calculates the factorial of a given positive integer:
ruby # factorial.rb def factorial(n) return 1 if n == 0 return n * factorial(n - 1) end
Now, let’s write a unit test for this method using Ruby’s built-in testing framework, MiniTest:
ruby # test_factorial.rb require 'minitest/autorun' require_relative 'factorial' class TestFactorial < Minitest::Test def test_factorial_of_5 assert_equal 120, factorial(5) end def test_factorial_of_0 assert_equal 1, factorial(0) end end
In this example, we’ve defined two test cases using Minitest’s test framework. The test_factorial_of_5 and test_factorial_of_0 methods test the factorial method for the input values 5 and 0, respectively. The assert_equal method verifies if the expected output matches the actual output of the factorial method.
4. Running Unit Tests
To run the unit tests, execute the following command in the terminal:
ruby test_factorial.rb
If the tests pass, you’ll see an output indicating the number of tests run and the duration. If any test fails, the output will provide information about the failure, helping you diagnose and fix the issue.
5. Test-Driven Development (TDD) in Ruby
Test-Driven Development (TDD) is a development approach where tests are written before the actual code implementation. The TDD cycle typically consists of three steps: Red, Green, and Refactor.
- Red: In this phase, you start by writing a test that will initially fail because there is no code to satisfy it.
- Green: The next step involves writing the minimum code necessary to make the test pass. The focus is on making the test pass, without concerning yourself with the overall design or efficiency at this stage.
- Refactor: After the test is passing, you can refactor the code to improve its design, remove duplication, and enhance performance. The test suite acts as a safety net to ensure the code remains functional during refactoring.
The TDD approach provides several benefits, including:
- Clear Requirements: Tests serve as a clear specification of what the code should do, guiding the development process.
- Focused Development: TDD helps developers stay focused on building the features that are essential to the application.
- Rapid Feedback: TDD provides rapid feedback about the code’s correctness, leading to early bug detection.
- Sustainable Codebase: By writing tests first, developers are encouraged to keep the codebase clean, modular, and maintainable.
6. Example of Test-Driven Development (TDD) in Ruby
Let’s continue with the previous factorial example and implement the factorial method using the TDD approach:
Red: We begin by writing a test for the factorial method, knowing that the method doesn’t exist yet. We expect the test to fail.
ruby # test_factorial_tdd.rb require 'minitest/autorun' require_relative 'factorial' class TestFactorialTDD < Minitest::Test def test_factorial_of_5 assert_equal 120, factorial(5) end def test_factorial_of_0 assert_equal 1, factorial(0) end def test_factorial_of_10 assert_equal 3628800, factorial(10) end end Green: Now, let's implement the factorial method in the factorial.rb file: ruby Copy code # factorial.rb def factorial(n) return 1 if n == 0 return n * factorial(n - 1) end
Refactor: Since we have a simple and functional implementation, there is no immediate need for refactoring in this case. However, during a real-world development process, you might refactor the code to improve its readability, performance, and maintainability.
7. Running the TDD Tests
Execute the following command to run the TDD tests:
ruby test_factorial_tdd.rb
The TDD approach ensures that the code is developed incrementally, with a strong focus on test coverage. The process of writing tests first, implementing the code, and then refactoring promotes a sustainable and reliable codebase.
Conclusion
In this blog, we explored the world of testing Ruby applications, focusing on two essential approaches: Unit Testing and Test-Driven Development (TDD). We discussed the importance of testing and how it contributes to building high-quality, maintainable, and bug-free code. With the knowledge of Unit Testing and TDD, Ruby developers can confidently write code that stands the test of time, empowers collaboration, and delivers exceptional software products.
Remember, testing is not a one-time activity; it is an ongoing process that evolves with your application. Embrace testing as an integral part of your development workflow, and you’ll reap the rewards in the form of a robust and reliable Ruby application. Happy testing!
Table of Contents
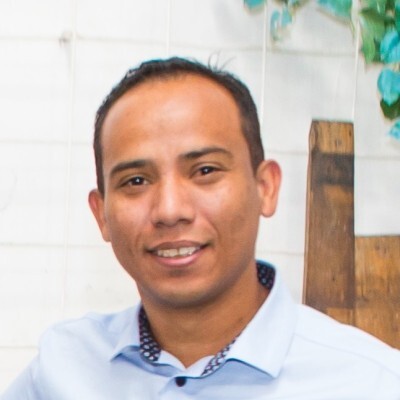
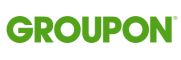