Ruby for Video Game Development: Creating Engaging Experiences
In the ever-evolving world of video game development, programmers are constantly on the lookout for versatile and efficient programming languages. While heavyweights like C++, Java, and Python have traditionally dominated this field, there’s another contender that’s been making waves in recent years: Ruby.
Table of Contents
In this blog post, we’ll explore the exciting realm of Ruby for video game development. We’ll delve into why Ruby is a viable option for creating engaging gaming experiences, explore its benefits, and provide practical code samples to get you started on your game development journey. So, whether you’re a seasoned game developer looking to expand your toolkit or a novice eager to dive into the world of game programming, this guide is for you.
1. Why Choose Ruby for Game Development?
1.1. Elegance and Productivity
Ruby is renowned for its elegant and concise syntax, which emphasizes readability and developer-friendly coding practices. This elegance translates well into game development, making your codebase cleaner and more maintainable. Ruby’s syntax allows you to focus on the game logic rather than wrestling with complex syntax rules, boosting productivity and creativity.
Let’s take a look at a simple example of Ruby’s elegance. Here’s how you can define a class for a player character in a game:
ruby class Player attr_accessor :name, :health, :score def initialize(name) @name = name @health = 100 @score = 0 end def take_damage(damage) @health -= damage end def increase_score(points) @score += points end end
The code is clean, easy to understand, and allows you to quickly define and manipulate game objects.
1.2. Active and Supportive Community
Ruby boasts an active and supportive community of developers who are passionate about the language. This means you’ll find a wealth of resources, libraries, and frameworks that can be leveraged to streamline your game development process. One such example is Gosu, a 2D game development library for Ruby, which provides essential tools for creating games with ease.
1.3. Cross-Platform Compatibility
With Ruby, you can create games that run on multiple platforms with minimal effort. Thanks to its cross-platform capabilities, you can target Windows, macOS, and Linux, allowing you to reach a broader audience. This flexibility is particularly valuable for indie developers and small studios with limited resources.
2. Getting Started with Ruby Game Development
Now that we’ve covered why Ruby is a compelling choice for game development, let’s dive into the practical steps to get started. In this section, we’ll outline the essential tools and libraries and provide code samples to illustrate key concepts.
2.1. Setting Up Your Development Environment
Before you start coding your game, you’ll need to set up your development environment. Ensure you have Ruby installed on your system, and consider using a version management tool like RVM (Ruby Version Manager) to manage different Ruby versions for various projects.
Once you have Ruby installed, you can start using the Gosu gem. Install it using the following command:
bash gem install gosu
2.2. Creating Your First Game
Let’s begin by creating a simple “Hello World” game using Gosu. This example will help you understand the basics of setting up a game window and handling user input.
ruby require 'gosu' class MyGame < Gosu::Window def initialize super(640, 480) self.caption = 'My First Gosu Game' end def update # Game logic goes here end def draw # Rendering code goes here end end game = MyGame.new game.show
In this code, we first require the Gosu library, create a class that inherits from Gosu::Window, and define the initialize, update, and draw methods. The initialize method sets up the game window, while the update and draw methods are where you implement your game’s logic and rendering, respectively.
2.3. Handling User Input
Games often rely on user input for player interaction. Gosu simplifies this process by providing built-in methods to handle keyboard and mouse input. Here’s a basic example of how you can move a player character using arrow keys:
ruby class MyGame < Gosu::Window def initialize # ... end def update if Gosu.button_down?(Gosu::KB_LEFT) # Move the player left elsif Gosu.button_down?(Gosu::KB_RIGHT) # Move the player right end end def draw # ... end end
In this code, we check if the left or right arrow keys are pressed using Gosu.button_down? and then perform the corresponding actions. This simple example demonstrates how easily you can handle user input in a Ruby-based game.
2.4. Creating Game Objects
In any game, you’ll need to manage various game objects, such as characters, enemies, and items. Ruby’s object-oriented nature makes it an excellent choice for modeling these objects. Here’s an example of creating a basic game object class:
ruby class GameObject attr_accessor :x, :y def initialize(x, y) @x = x @y = y end def update # Logic for updating the object goes here end def draw # Rendering code for the object goes here end end
This GameObject class can serve as a blueprint for creating various game objects in your Ruby game.
3. Tips for Successful Ruby Game Development
As you embark on your journey to create engaging video game experiences with Ruby, consider these tips to ensure a smooth development process:
3.1. Plan Your Game
Before diving into coding, have a clear game design in mind. Define your game mechanics, objectives, and overall vision. Planning helps you stay focused and organized throughout the development process.
3.2. Use Libraries and Frameworks Wisely
Take advantage of existing Ruby game development libraries and frameworks like Gosu, Chingu, and TexPlay. These tools can save you time and effort by providing pre-built functionality for common game development tasks.
3.3. Optimize Performance
While Ruby offers productivity benefits, it may not be as performant as languages like C++. Be mindful of performance bottlenecks, and optimize critical sections of your code if necessary.
3.4. Test Thoroughly
Testing is crucial in game development. Ensure your game functions as intended by conducting thorough testing and debugging. Tools like RSpec and MiniTest can help streamline the testing process.
3.5. Learn Continuously
Stay up-to-date with Ruby game development trends, and continuously improve your skills. Engage with the Ruby community, read books, and follow online tutorials to expand your knowledge.
Conclusion
Ruby may not be the first language that comes to mind for game development, but it offers a unique blend of elegance, productivity, and community support that can make it a valuable choice for crafting engaging gaming experiences. Whether you’re a hobbyist or a professional game developer, Ruby provides a fresh perspective on game programming that’s worth exploring.
In this blog post, we’ve touched on the key reasons to consider Ruby for game development, provided a step-by-step guide to getting started, and offered tips for success along the way. With the right mindset and the power of Ruby at your fingertips, you can embark on an exciting journey to create captivating video games that captivate players worldwide. So, what are you waiting for? Start coding your dream game today!
Table of Contents
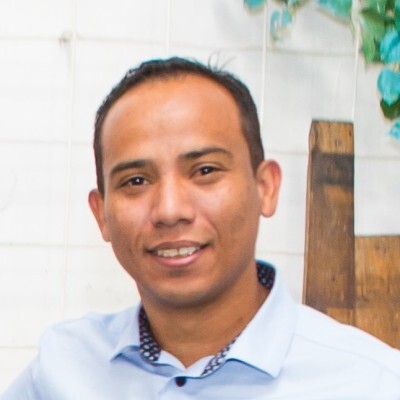
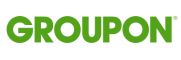